Compare commits
No commits in common. "master" and "master" have entirely different histories.
@ -1,36 +0,0 @@
|
||||
# Static files
|
||||
static/instructions
|
||||
static/minifigs
|
||||
static/parts
|
||||
static/sets
|
||||
|
||||
# Docker
|
||||
Dockerfile
|
||||
compose.yaml
|
||||
|
||||
# Documentation
|
||||
docs/
|
||||
LICENSE
|
||||
*.md
|
||||
*.sample
|
||||
|
||||
# Temporary
|
||||
*.csv
|
||||
|
||||
# Database
|
||||
*.db
|
||||
*.db-shm
|
||||
*.db-wal
|
||||
|
||||
# Python
|
||||
**/__pycache__
|
||||
*.pyc
|
||||
|
||||
# Git
|
||||
.git
|
||||
|
||||
# IDE
|
||||
.vscode
|
||||
|
||||
# Dev
|
||||
test-server.sh
|
316
.env.sample
316
.env.sample
@ -1,314 +1,2 @@
|
||||
# Note on *_DEFAULT_ORDER
|
||||
# If set, it will append a direct ORDER BY <whatever you set> to the SQL query
|
||||
# while listing objects. You can look at the structure of the SQLite database to
|
||||
# see the schema and the column names. Some fields are compound and not visible
|
||||
# directly from the schema (joins). You can check the query in the */list.sql and */base/*.sql files
|
||||
# in the source to see all column names.
|
||||
# The usual syntax for those variables is "<table>"."<column>" [ASC|DESC].
|
||||
# For composite fields (CASE, SUM, COUNT) the syntax is <field>, there is no <table> name.
|
||||
# For instance:
|
||||
# - "table"."name" (by "table"."name", default order)
|
||||
# - "table"."name" ASC (by "table"."name", ascending)
|
||||
# - "table"."name" DESC (by "table"."name", descending)
|
||||
# - "field" (by "field", default order)
|
||||
# - ...
|
||||
# You can combine the ordering options.
|
||||
# You can use the special column name 'rowid' to order by insertion order.
|
||||
|
||||
# Optional: A unique password to protect sensitive areas of the app
|
||||
# Useful if you want to share the page with other in read-only
|
||||
# Security: Currently not fully protecting the socket action, would be better
|
||||
# to have server-side sessions, with flask-session for instance
|
||||
# BK_AUTHENTICATION_PASSWORD=my-secret-password
|
||||
|
||||
# Optional/Mandatory: A unique key used to sign the secrets when using authentication
|
||||
# Do not share it with anyone, and you MUST make it random.
|
||||
# You can use the following command in your terminal to generate such random secret:
|
||||
# python3 -c 'import secrets; print(secrets.token_hex())'
|
||||
# BK_AUTHENTICATION_KEY=change-this-to-something-random
|
||||
|
||||
# Optional: Pattern of the link to Bricklink for a part. Will be passed to Python .format()
|
||||
# Default: https://www.bricklink.com/v2/catalog/catalogitem.page?P={part}
|
||||
# BK_BRICKLINK_LINK_PART_PATTERN=
|
||||
|
||||
# Optional: Display Bricklink links wherever applicable
|
||||
# Default: false
|
||||
# BK_BRICKLINK_LINKS=true
|
||||
|
||||
# Optional: Path to the database.
|
||||
# Useful if you need it mounted in a Docker volume. Keep in mind that it will not
|
||||
# do any check on the existence of the path, or if it is dangerous.
|
||||
# Default: ./app.db
|
||||
# BK_DATABASE_PATH=/var/lib/bricktracker/app.db
|
||||
|
||||
# Optional: Format of the timestamp added to the database file when downloading it
|
||||
# Check https://docs.python.org/3/library/time.html#time.strftime for format details
|
||||
# Default: %Y-%m-%d-%H-%M-%S
|
||||
# BK_DATABASE_TIMESTAMP_FORMAT=%Y%m%d-%H%M%S
|
||||
|
||||
# Optional: Enable debugging.
|
||||
# Default: false
|
||||
# BK_DEBUG=true
|
||||
|
||||
# Optional: Default number of items per page displayed for big tables
|
||||
# You can put whatever value but the exist steps are: 10, 25, 50, 100, 500, 1000
|
||||
# Default: 25
|
||||
# BK_DEFAULT_TABLE_PER_PAGE=50
|
||||
|
||||
# Optional: if set up, will add a CORS allow origin restriction to the socket.
|
||||
# Default:
|
||||
# Legacy name: DOMAIN_NAME
|
||||
# BK_DOMAIN_NAME=http://localhost:3333
|
||||
|
||||
# Optional: Format of the timestamp for files on disk (instructions, themes)
|
||||
# Check https://docs.python.org/3/library/time.html#time.strftime for format details
|
||||
# Default: %d/%m/%Y, %H:%M:%S
|
||||
# BK_FILE_DATETIME_FORMAT=%m/%d/%Y, %H:%M
|
||||
|
||||
# Optional: IP address the server will listen on.
|
||||
# Default: 0.0.0.0
|
||||
# BK_HOST=0.0.0.0
|
||||
|
||||
# Optional: By default, accordion items are linked together and only one can be in
|
||||
# a collapsed state. This makes all the items indepedent.
|
||||
# Default: false
|
||||
# BK_INDEPENDENT_ACCORDIONS=true
|
||||
|
||||
# Optional: A comma separated list of extensions allowed for uploading and displaying
|
||||
# instruction files. You need to keep the dot (.) in the extension.
|
||||
# Security: not really
|
||||
# Default: .pdf
|
||||
# BK_INSTRUCTIONS_ALLOWED_EXTENSIONS=.pdf, .docx, .png
|
||||
|
||||
# Optional: Folder where to store the instructions, relative to the '/app/static/' folder
|
||||
# Default: instructions
|
||||
# BK_INSTRUCTIONS_FOLDER=/var/lib/bricktracker/instructions/
|
||||
|
||||
# Optional: Hide the 'Add' entry from the menu. Does not disable the route.
|
||||
# Default: false
|
||||
# BK_HIDE_ADD_SET=true
|
||||
|
||||
# Optional: Hide the 'Bulk add' entry from the add page. Does not disable the route.
|
||||
# Default: false
|
||||
# BK_HIDE_ADD_BULK_SET=true
|
||||
|
||||
# Optional: Hide the 'Admin' entry from the menu. Does not disable the route.
|
||||
# Default: false
|
||||
# BK_HIDE_ADMIN=true
|
||||
|
||||
# Optional: Hide the 'Instructions' entry from the menu. Does not disable the route.
|
||||
# Default: false
|
||||
# BK_HIDE_ALL_INSTRUCTIONS=true
|
||||
|
||||
# Optional: Hide the 'Minifigures' entry from the menu. Does not disable the route.
|
||||
# Default: false
|
||||
# BK_HIDE_ALL_MINIFIGURES=true
|
||||
|
||||
# Optional: Hide the 'Parts' entry from the menu. Does not disable the route.
|
||||
# Default: false
|
||||
# BK_HIDE_ALL_PARTS=true
|
||||
|
||||
# Optional: Hide the 'Problems' entry from the menu. Does not disable the route.
|
||||
# Default: false
|
||||
# Legacy name: BK_HIDE_MISSING_PARTS
|
||||
# BK_HIDE_ALL_PROBLEMS_PARTS=true
|
||||
|
||||
# Optional: Hide the 'Sets' entry from the menu. Does not disable the route.
|
||||
# Default: false
|
||||
# BK_HIDE_ALL_SETS=true
|
||||
|
||||
# Optional: Hide the 'Storages' entry from the menu. Does not disable the route.
|
||||
# Default: false
|
||||
# BK_HIDE_ALL_STORAGES=true
|
||||
|
||||
# Optional: Hide the 'Instructions' entry in a Set card
|
||||
# Default: false
|
||||
# BK_HIDE_SET_INSTRUCTIONS=true
|
||||
|
||||
# Optional: Hide the 'Damaged' column from the parts table.
|
||||
# Default: false
|
||||
# BK_HIDE_TABLE_DAMAGED_PARTS=true
|
||||
|
||||
# Optional: Hide the 'Missing' column from the parts table.
|
||||
# Default: false
|
||||
# BK_HIDE_TABLE_MISSING_PARTS=true
|
||||
|
||||
# Optional: Hide the 'Wishlist' entry from the menu. Does not disable the route.
|
||||
# Default: false
|
||||
# BK_HIDE_WISHES=true
|
||||
|
||||
# Optional: Change the default order of minifigures. By default ordered by insertion order.
|
||||
# Useful column names for this option are:
|
||||
# - "rebrickable_minifigures"."figure": minifigure ID (fig-xxxxx)
|
||||
# - "rebrickable_minifigures"."number": minifigure ID as an integer (xxxxx)
|
||||
# - "rebrickable_minifigures"."name": minifigure name
|
||||
# Default: "rebrickable_minifigures"."name" ASC
|
||||
# BK_MINIFIGURES_DEFAULT_ORDER="rebrickable_minifigures"."name" ASC
|
||||
|
||||
# Optional: Folder where to store the minifigures images, relative to the '/app/static/' folder
|
||||
# Default: minifigs
|
||||
# BK_MINIFIGURES_FOLDER=minifigures
|
||||
|
||||
# Optional: Disable threading on the task executed by the socket.
|
||||
# You should not need to change this parameter unless you are debugging something with the
|
||||
# socket itself.
|
||||
# Default: false
|
||||
# BK_NO_THREADED_SOCKET=true
|
||||
|
||||
# Optional: Change the default order of parts. By default ordered by insertion order.
|
||||
# Useful column names for this option are:
|
||||
# - "bricktracker_parts"."part": part number
|
||||
# - "bricktracker_parts"."spare": part is a spare part
|
||||
# - "rebrickable_parts"."name": part name
|
||||
# - "rebrickable_parts"."color_name": part color name
|
||||
# - "total_missing": number of missing parts
|
||||
# Default: "rebrickable_parts"."name" ASC, "rebrickable_parts"."color_name" ASC, "bricktracker_parts"."spare" ASC
|
||||
# BK_PARTS_DEFAULT_ORDER="total_missing" DESC, "rebrickable_parts"."name"."name" ASC
|
||||
|
||||
# Optional: Folder where to store the parts images, relative to the '/app/static/' folder
|
||||
# Default: parts
|
||||
# BK_PARTS_FOLDER=parts
|
||||
|
||||
# Optional: Port the server will listen on.
|
||||
# Default: 3333
|
||||
# BK_PORT=3333
|
||||
|
||||
# Optional: Format of the timestamp for purchase dates
|
||||
# Check https://docs.python.org/3/library/time.html#time.strftime for format details
|
||||
# Default: %d/%m/%Y
|
||||
# BK_PURCHASE_DATE_FORMAT=%m/%d/%Y
|
||||
|
||||
# Optional: Currency to display for purchase prices.
|
||||
# Default: €
|
||||
# BK_PURCHASE_CURRENCY=£
|
||||
|
||||
# Optional: Change the default order of purchase locations. By default ordered by insertion order.
|
||||
# Useful column names for this option are:
|
||||
# - "bricktracker_metadata_purchase_locations"."name" ASC: storage name
|
||||
# Default: "bricktracker_metadata_purchase_locations"."name" ASC
|
||||
# BK_PURCHASE_LOCATION_DEFAULT_ORDER="bricktracker_metadata_purchase_locations"."name" ASC
|
||||
|
||||
# Optional: Shuffle the lists on the front page.
|
||||
# Default: false
|
||||
# Legacy name: RANDOM
|
||||
# BK_RANDOM=true
|
||||
|
||||
# Optional/Mandatory: The API key used to retrieve sets from the Rebrickable API.
|
||||
# It is not necessary to set it to display the site, but it will limit its capabilities
|
||||
# as you will not be able to add new sets
|
||||
# Default:
|
||||
# Legacy name: REBRICKABLE_API_KEY
|
||||
# BK_REBRICKABLE_API_KEY=xxxx
|
||||
|
||||
# Optional: URL of the image representing a missing image in Rebrickable
|
||||
# Default: https://rebrickable.com/static/img/nil.png
|
||||
# BK_REBRICKABLE_IMAGE_NIL=
|
||||
|
||||
# Optional: URL of the image representing a missing minifigure image in Rebrickable
|
||||
# Default: https://rebrickable.com/static/img/nil_mf.jpg
|
||||
# BK_REBRICKABLE_IMAGE_NIL_MINIFIGURE=
|
||||
|
||||
# Optional: Pattern of the link to Rebrickable for a minifigure. Will be passed to Python .format()
|
||||
# Default: https://rebrickable.com/minifigs/{figure}
|
||||
# BK_REBRICKABLE_LINK_MINIFIGURE_PATTERN=
|
||||
|
||||
# Optional: Pattern of the link to Rebrickable for a part. Will be passed to Python .format()
|
||||
# Default: https://rebrickable.com/parts/{part}/_/{color}
|
||||
# BK_REBRICKABLE_LINK_PART_PATTERN=
|
||||
|
||||
# Optional: Pattern of the link to Rebrickable for instructions. Will be passed to Python .format()
|
||||
# Default: https://rebrickable.com/instructions/{path}
|
||||
# BK_REBRICKABLE_LINK_INSTRUCTIONS_PATTERN=
|
||||
|
||||
# Optional: User-Agent to use when querying Rebrickable outside of the Rebrick python library
|
||||
# Default: 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
|
||||
# BK_REBRICKABLE_USER_AGENT=
|
||||
|
||||
# Optional: Display Rebrickable links wherever applicable
|
||||
# Default: false
|
||||
# Legacy name: LINKS
|
||||
# BK_REBRICKABLE_LINKS=true
|
||||
|
||||
# Optional: The amount of items to retrieve per Rebrickable API call when.
|
||||
# Default: 100
|
||||
# BK_REBRICKABLE_PAGE_SIZE=200
|
||||
|
||||
# Optional: URL to the unofficial retired sets list on Google Sheets
|
||||
# Default: https://docs.google.com/spreadsheets/d/1rlYfEXtNKxUOZt2Mfv0H17DvK7bj6Pe0CuYwq6ay8WA/gviz/tq?tqx=out:csv&sheet=Sorted%20by%20Retirement%20Date
|
||||
# BK_RETIRED_SETS_FILE_URL=
|
||||
|
||||
# Optional: Path to the unofficial retired sets lists
|
||||
# You can name it whatever you want, but content has to be a CSV
|
||||
# Default: ./retired_sets.csv
|
||||
# BK_RETIRED_SETS_PATH=/var/lib/bricktracker/retired_sets.csv
|
||||
|
||||
# Optional: Change the default order of sets. By default ordered by insertion order.
|
||||
# Useful column names for this option are:
|
||||
# - "rebrickable_sets"."set": set number as a string
|
||||
# - "rebrickable_sets"."number": the number part of set as an integer
|
||||
# - "rebrickable_sets"."version": the version part of set as an integer
|
||||
# - "rebrickable_sets"."name": set name
|
||||
# - "rebrickable_sets"."year": set release year
|
||||
# - "rebrickable_sets"."number_of_parts": set number of parts
|
||||
# - "total_missing": number of missing parts
|
||||
# - "total_minifigures": number of minifigures
|
||||
# Default: "rebrickable_sets"."number" DESC, "rebrickable_sets"."version" ASC
|
||||
# BK_SETS_DEFAULT_ORDER="rebrickable_sets"."year" ASC
|
||||
|
||||
# Optional: Folder where to store the sets images, relative to the '/app/static/' folder
|
||||
# Default: sets
|
||||
# BK_SETS_FOLDER=sets
|
||||
|
||||
# Optional: Make the grid filters displayed by default, rather than collapsed
|
||||
# Default: false
|
||||
# BK_SHOW_GRID_FILTERS=true
|
||||
|
||||
# Optional: Make the grid sort displayed by default, rather than collapsed
|
||||
# Default: false
|
||||
# BK_SHOW_GRID_SORT=true
|
||||
|
||||
# Optional: Skip saving or displaying spare parts
|
||||
# Default: false
|
||||
# BK_SKIP_SPARE_PARTS=true
|
||||
|
||||
# Optional: Namespace of the Socket.IO socket
|
||||
# Default: bricksocket
|
||||
# BK_SOCKET_NAMESPACE=customsocket
|
||||
|
||||
# Optional: Namespace of the Socket.IO path
|
||||
# Default: /bricksocket/
|
||||
# BK_SOCKET_PATH=custompath
|
||||
|
||||
# Optional: Change the default order of storages. By default ordered by insertion order.
|
||||
# Useful column names for this option are:
|
||||
# - "bricktracker_metadata_storages"."name" ASC: storage name
|
||||
# Default: "bricktracker_metadata_storages"."name" ASC
|
||||
# BK_STORAGE_DEFAULT_ORDER="bricktracker_metadata_storages"."name" ASC
|
||||
|
||||
# Optional: URL to the themes.csv.gz on Rebrickable
|
||||
# Default: https://cdn.rebrickable.com/media/downloads/themes.csv.gz
|
||||
# BK_THEMES_FILE_URL=
|
||||
|
||||
# Optional: Path to the themes file
|
||||
# You can name it whatever you want, but content has to be a CSV
|
||||
# Default: ./themes.csv
|
||||
# BK_THEMES_PATH=/var/lib/bricktracker/themes.csv
|
||||
|
||||
# Optional: Timezone to use to display datetimes
|
||||
# Check your system for available timezone/TZ values
|
||||
# Default: Etc/UTC
|
||||
# BK_TIMEZONE=Europe/Copenhagen
|
||||
|
||||
# Optional: Use remote image rather than the locally stored ones
|
||||
# Also prevents downloading any image when adding sets
|
||||
# Default: false
|
||||
# BK_USE_REMOTE_IMAGES=true
|
||||
|
||||
# Optional: Change the default order of sets. By default ordered by insertion order.
|
||||
# Useful column names for this option are:
|
||||
# - "bricktracker_wishes"."set": set number as a string
|
||||
# - "bricktracker_wishes"."name": set name
|
||||
# - "bricktracker_wishes"."year": set release year
|
||||
# - "bricktracker_wishes"."number_of_parts": set number of parts
|
||||
# Default: "bricktracker_wishes"."rowid" DESC
|
||||
# BK_WISHES_DEFAULT_ORDER="bricktracker_wishes"."set" DESC
|
||||
REBRICKABLE_API_KEY=xxxx
|
||||
DOMAIN_NAME=https://lego.example.com
|
||||
|
33
.gitignore
vendored
33
.gitignore
vendored
@ -1,26 +1,11 @@
|
||||
# Application
|
||||
api
|
||||
.env
|
||||
*.db
|
||||
*.db-shm
|
||||
*.db-wal
|
||||
|
||||
# Python specifics
|
||||
__pycache__/
|
||||
*.pyc
|
||||
|
||||
# Static folders
|
||||
static/instructions/
|
||||
static/minifigs/
|
||||
static/minifigures/
|
||||
static/parts/
|
||||
static/sets/
|
||||
|
||||
# IDE
|
||||
.vscode/
|
||||
|
||||
# Temporary
|
||||
*.csv
|
||||
/local/
|
||||
|
||||
# Apple idiocy
|
||||
.DS_Store
|
||||
*.db
|
||||
*.png
|
||||
*.pdf
|
||||
*.jpg
|
||||
*.log
|
||||
/info
|
||||
/__pycache__
|
||||
*.bk
|
||||
|
323
CHANGELOG.md
323
CHANGELOG.md
@ -1,323 +0,0 @@
|
||||
# Changelog
|
||||
|
||||
## 1.2.2:
|
||||
|
||||
This release fixes a bug where orphaned parts in the `inventory` table are blocking the database upgrade.
|
||||
|
||||
## 1.2.1:
|
||||
|
||||
This release fixes a bug where you could not add a set if no metadata was configured.
|
||||
|
||||
## 1.2.0:
|
||||
|
||||
> **Warning**
|
||||
> "Missing" part has been renamed to "Problems" to accomodate for missing and damaged parts.
|
||||
> The associated environment variables have changed named (the old names are still valid)
|
||||
|
||||
### Environment
|
||||
|
||||
- Renamed: `BK_HIDE_MISSING_PARTS` -> `BK_HIDE_ALL_PROBLEMS_PARTS`
|
||||
- Added: `BK_HIDE_TABLE_MISSING_PARTS`, hide the Missing column in all tables
|
||||
- Added: `BK_HIDE_TABLE_DAMAGED_PARTS`, hide the Damaged column in all tables
|
||||
- Added: `BK_SHOW_GRID_SORT`, show the sort options on the grid by default
|
||||
- Added: `BK_SHOW_GRID_FILTERS`, show the filter options on the grid by default
|
||||
- Added: `BK_HIDE_ALL_STORAGES`, hide the "Storages" menu entry
|
||||
- Added: `BK_STORAGE_DEFAULT_ORDER`, ordering of storages
|
||||
- Added: `BK_PURCHASE_LOCATION_DEFAULT_ORDER`, ordering of purchase locations
|
||||
- Added: `BK_PURCHASE_CURRENCY`, currency to display for purchase prices
|
||||
- Added: `BK_PURCHASE_DATE_FORMAT`, date format for purchase dates
|
||||
- Documented: `BK_FILE_DATETIME_FORMAT`, date format for files on disk (instructions, theme)
|
||||
|
||||
### Code
|
||||
|
||||
- Changer
|
||||
- Revert the checked state of a checkbox if an error occured
|
||||
|
||||
- Form
|
||||
- Migrate missing input fields to BrickChanger
|
||||
|
||||
- General cleanup
|
||||
|
||||
- Metadata
|
||||
- Underlying class to implement more metadata-like features
|
||||
|
||||
- Minifigure
|
||||
- Deduplicate
|
||||
- Compute number of parts
|
||||
|
||||
- Parts
|
||||
- Damaged parts
|
||||
|
||||
- Sets
|
||||
- Refresh data from Rebrickable
|
||||
- Fix missing @login_required for set deletion
|
||||
- Ownership
|
||||
- Tags
|
||||
- Storage
|
||||
- Purchase location, date, price
|
||||
|
||||
- Storage
|
||||
- Storage content and list
|
||||
|
||||
- Socket
|
||||
- Add decorator for rebrickable, authenticated and threaded socket actions
|
||||
|
||||
- SQL
|
||||
- Allow for advanced migration scenarios through companion python files
|
||||
- Add a bunch of the requested fields into the database for future implementation
|
||||
|
||||
- Wish
|
||||
- Requester
|
||||
|
||||
### UI
|
||||
|
||||
- Add
|
||||
- Allow adding or bulk adding by pressing Enter in the input field
|
||||
|
||||
- Admin
|
||||
- Grey out legacy tables in the database view
|
||||
- Checkboxes renamed to Set statuses
|
||||
- List of sets that may need to be refreshed
|
||||
|
||||
- Cards
|
||||
- Use macros for badge in the card header
|
||||
|
||||
- Form
|
||||
- Add a clear button for dynamic text inputs
|
||||
- Add error message in a tooltip for dynamic inputs
|
||||
|
||||
- Minifigure
|
||||
- Display number of parts
|
||||
|
||||
- Parts
|
||||
- Use Rebrickable URL if stored (+ color code)
|
||||
- Display color and transparency
|
||||
- Display if print of another part
|
||||
- Display prints using the same base
|
||||
- Damaged parts
|
||||
- Display same parts using a different color
|
||||
|
||||
- Sets
|
||||
- Add a flag to hide instructions in a set
|
||||
- Make checkbox clickable on the whole width of the card
|
||||
- Management
|
||||
- Ownership
|
||||
- Tags
|
||||
- Refresh
|
||||
- Storage
|
||||
- Purchase location, date, price
|
||||
|
||||
- Sets grid
|
||||
- Collapsible controls depending on screen size
|
||||
- Manually collapsible filters (with configuration variable for default state)
|
||||
- Manually collapsible sort (with configuration variable for default state)
|
||||
- Clear search bar
|
||||
|
||||
- Storage
|
||||
- Storage list
|
||||
- Storage content
|
||||
|
||||
- Wish
|
||||
- Requester
|
||||
|
||||
## 1.1.1: PDF Instructions Download
|
||||
|
||||
### Instructions
|
||||
|
||||
- Added buttons for instructions download from Rebrickable
|
||||
|
||||
|
||||
## 1.1.0: Deduped sets, custom checkboxes and database upgrade
|
||||
|
||||
### Database
|
||||
|
||||
- Sets
|
||||
- Deduplicating rebrickable sets (unique) and bricktracker sets (can be n bricktracker sets for one rebrickable set)
|
||||
|
||||
### Docs
|
||||
|
||||
- Removed extra `<br>` to accomodate Gitea Markdown
|
||||
- Add an organized DOCS.md documentation page
|
||||
- Database upgrade/migration
|
||||
- Checkboxes
|
||||
|
||||
### Code
|
||||
|
||||
- Admin
|
||||
- Split the views before admin because an unmanageable monster view
|
||||
|
||||
- Checkboxes
|
||||
- Customizable checkboxes for set (amount and names, displayed on the grid or not)
|
||||
- Replaced the 3 original routes to update the status with a generic route to accomodate any custom status
|
||||
|
||||
- Instructions
|
||||
- Base instructions on RebrickableSet (the generic one) rather than BrickSet (the specific one)
|
||||
- Refine set number detection in file name by making sure each first items is an integer
|
||||
|
||||
- Python
|
||||
- Make stricter function definition with no "arg_or_keyword" parameters
|
||||
|
||||
- Records
|
||||
- Consolidate the select() -> not None or Exception -> ingest() process duplicated in every child class
|
||||
|
||||
- SQL
|
||||
- Forward-only migration mechanism
|
||||
- Check for database too far in version
|
||||
- Inject the database version in the file when downloading it
|
||||
- Quote all indentifiers as best practice
|
||||
- Allow insert query to be overriden
|
||||
- Allow insert query to force not being deferred even if not committed
|
||||
- Allow select query to push context in BrickRecord and BrickRecordList
|
||||
- Make SQL record counters failsafe as they are used in the admin and it should always work
|
||||
- Remove BrickSQL.initialize() as it is replaced by upgrade()
|
||||
|
||||
- Sets
|
||||
- Now that it is deduplicated, adding the same set more than once will not pull it fully from the Rebrickable API (minifigures and parts)
|
||||
- Make RebrickableSet extend BrickRecord since it is now an item in database
|
||||
- Make BrickSet extend RebrickableSet now that RebrickableSet is a proper database item
|
||||
|
||||
### UI
|
||||
|
||||
- Checkboxes
|
||||
- Possibility to hide the checkbox in the grid ("Sets") but sill have all them in the set details
|
||||
- Management
|
||||
|
||||
- Database
|
||||
- Migration tool
|
||||
|
||||
- Javascript
|
||||
- Generic BrickChanger class to handle quick modification through a JSON request with a visual feedback indicator
|
||||
- Simplify the way javascript scripts are loaded and instantiated
|
||||
|
||||
- Set grid
|
||||
- Filter by checkboxes and NOT checkboxes
|
||||
|
||||
- Tables
|
||||
- Fix table search looking inside links pills
|
||||
|
||||
- Wishlist
|
||||
- Add Rebrickable link badge for sets (@matthew)
|
||||
|
||||
## 1.0.0: New Year revamp
|
||||
|
||||
### Code
|
||||
|
||||
- Authentication
|
||||
- Basic authentication mechanism with ONE password to protect admin and writes
|
||||
- CSV
|
||||
- Remove dependencies to numpy and panda for simpler built-in csv
|
||||
- Code
|
||||
- Refactored the Python code
|
||||
- Modularity (more functions, splitting files)
|
||||
- Type hinting whenever possible
|
||||
- Flake8 linter
|
||||
- Retained most of the original behaviour (with its quirks)
|
||||
- Colors
|
||||
- Remove dependency on color.csv
|
||||
- Configuration
|
||||
- Moved all the hard-coded parameters into configuration variables
|
||||
- Most of the variables are configuration through environment variables
|
||||
- Force instruction, sets, etc path to be relative to static
|
||||
- Docker
|
||||
- Added an entrypoint to grab PORT / HOST from the environment if set
|
||||
- Remove the need to seed the container with files (*.csv, nil files)
|
||||
- Flask
|
||||
- Fix improper socketio.run(app.run()) call which lead to hard crash on ^C
|
||||
- Make use of url_for to create URLs
|
||||
- Use blueprints to implement routes
|
||||
- Move views into their own files
|
||||
- Split GET and POST methods into two different routes for clarity
|
||||
- Images
|
||||
- Add an option to use remote images from the Rebrickable CDN rather than downloading everything locally
|
||||
- Handle nil.png and nil_mf.jpg as true images in /static/sets/ so that they are downloaded whenever necessary when importing a se with missing images
|
||||
- Instructions
|
||||
- Scan the files once for the whole app, and re-use the data
|
||||
- Refresh the instructions from the admin
|
||||
- More lenient set number detection
|
||||
- Update when uploading a new one
|
||||
- Basic file management
|
||||
- Logs
|
||||
- Added log lines for change actions (add, check, missing, delete) so that the server is not silent when DEBUG=false
|
||||
- Minifigures
|
||||
- Added a variable to control default ordering
|
||||
- Part(s)
|
||||
- Added a variable to control default ordering of listing
|
||||
- Retired sets
|
||||
- Open the themes once for the whole app, and re-use the data
|
||||
- Do not hard fail if themes.csv is missing, simply display the IDs
|
||||
- Light management: resync, download
|
||||
- Set(s)
|
||||
- Reworked the set checkboxes with a dedicated route per status
|
||||
- Switch from homemade ID generator to proven UUID4 for sets ID
|
||||
- Does not interfere with previously created sets
|
||||
- Do not rely on sets.csv to check if the set exists
|
||||
- When adding, commit the set to database only once everything has been processed
|
||||
- Added a bulk add page
|
||||
- Keep spare parts when importing
|
||||
- Added a variable to control default ordering of listing
|
||||
- Socket
|
||||
- Make use of socket.io rooms to avoid broadcasting messages to all clients
|
||||
- SQLite
|
||||
- Do not hard fail if the database is not present or not initialized
|
||||
- Open the database once for the context, and re-use the connection
|
||||
- Move queries to .sql files and load them as Jinja templates
|
||||
- Use named arguments rather than sets for SQLite queries
|
||||
- Allow execute() to be deferred to the commit() call to avoid locking the database for long period while importing (locked while downloading images)
|
||||
- Themes
|
||||
- Open the themes once for the whole app, and re-use the data
|
||||
- Do not hard fail if themes.csv is missing, simply display the IDs
|
||||
- Light management: resync, download
|
||||
|
||||
### UI
|
||||
|
||||
- Admin
|
||||
- Initialize the database from the web interface
|
||||
- Reset the database
|
||||
- Delete the database
|
||||
- Download the database
|
||||
- Import the database
|
||||
- Display the configuration variables
|
||||
- Many things
|
||||
- Accordions
|
||||
- Added a flag to make the accordion items independent
|
||||
- Branding:
|
||||
- Add a brick as a logo (CC0 image from: https://iconduck.com/icons/71631/brick)
|
||||
- Global
|
||||
- Redesign of the whole app
|
||||
- Sticky menu bar on top of the page
|
||||
- Execution time and SQL stats for fun
|
||||
- Libraries
|
||||
- Switch from Bulma to Bootstrap, arbitrarily :D
|
||||
- Use of baguettebox for images (https://github.com/feimosi/baguetteBox.js)
|
||||
- Use of tinysort to sort and filter the grid (https://github.com/Sjeiti/TinySort)
|
||||
- Use of sortable for set card tables (https://github.com/tofsjonas/sortable)
|
||||
- Use of simple-datatables for big tables (https://github.com/fiduswriter/simple-datatables)
|
||||
- Minifigures
|
||||
- Added a detail view for a minifigure
|
||||
- Display which sets are using a minifigure
|
||||
- Display which sets are missing a minifigure
|
||||
- Parts
|
||||
- Added a detail view for a part
|
||||
- Display which sets are using a part
|
||||
- Display which sets are missing a part
|
||||
- Templates
|
||||
- Use a common base template
|
||||
- Use HTML fragments/macros for repeted or parametrics items
|
||||
- a 404 page for wrong URLs
|
||||
- an error page for expected error messages
|
||||
- an exception page for unexpected error messages
|
||||
- Set add
|
||||
- Two-tiered (with override) import where you see what you will import before importing it
|
||||
- Add a visual indicator that the socket is connected
|
||||
- Set card
|
||||
- Badges to display info like theme, year, parts, etc
|
||||
- Set image on top of the card, filling the space
|
||||
- Trick to have a blurry background image fill the void in the card
|
||||
- Save missing parts on input change rather than by clicking
|
||||
- Visual feedback of success
|
||||
- Parts and minifigure in accordions
|
||||
- Instructions file list
|
||||
- Set grid
|
||||
- 4-2-1 card distribution depending on screen size
|
||||
- Display the index with no set added, rather than redirecting
|
||||
- Keep last sort in a cookie, and trigger it on page load (can be cleared)
|
15
Dockerfile
15
Dockerfile
@ -1,11 +1,8 @@
|
||||
FROM python:3-slim
|
||||
|
||||
FROM python:slim
|
||||
WORKDIR /app
|
||||
|
||||
# Bricktracker
|
||||
COPY requirements.txt .
|
||||
RUN pip install -r requirements.txt
|
||||
COPY . .
|
||||
|
||||
# Python library requirements
|
||||
RUN pip --no-cache-dir install -r requirements.txt
|
||||
|
||||
ENTRYPOINT ["./entrypoint.sh"]
|
||||
RUN bash lego.sh
|
||||
#CMD ["python", "app.py"]
|
||||
CMD ["gunicorn","--bind","0.0.0.0:3333","app:app","--worker-class","eventlet"]
|
||||
|
204
README.md
204
README.md
@ -2,33 +2,215 @@
|
||||
|
||||
A web application for organizing and tracking LEGO sets, parts, and minifigures. Uses the Rebrickable API to fetch LEGO data and allows users to track missing pieces and collection status.
|
||||
|
||||
<a href="https://www.buymeacoffee.com/frederikb" target="_blank"><img src="https://cdn.buymeacoffee.com/buttons/v2/default-yellow.png" alt="Buy Me A Coffee" height="41" width="174"></a>
|
||||
> **Screenshots at the end of the readme!**
|
||||
|
||||
## Features
|
||||
|
||||
- Track multiple LEGO sets with their parts and minifigures
|
||||
- Mark sets as checked/collected
|
||||
- Mark minifigures as collected for a set
|
||||
- Track missing pieces
|
||||
- View parts inventory across sets
|
||||
- View minifigures across sets
|
||||
- Automatic updates for LEGO data (themes, colors, sets)
|
||||
- Wishlist to keep track of what to buy
|
||||
|
||||
## Prefered setup: pre-build docker image
|
||||
## Prerequisites
|
||||
|
||||
Use the provided [compose.yaml](compose.yaml) file.
|
||||
- Docker
|
||||
- Docker Compose
|
||||
- Rebrickable API key (from [Rebrickable](https://rebrickable.com/api/))
|
||||
|
||||
See [Quickstart](docs/quickstart.md) to get up and running right away.
|
||||
## Setup
|
||||
|
||||
See [Setup](docs/setup.md) for a more setup guide.
|
||||
1. Clone the repository:
|
||||
```bash
|
||||
git clone https://gitea.baerentsen.space/FrederikBaerentsen/BrickTracker.git
|
||||
cd BrickTracker
|
||||
mkdir static/{sets,instructions,parts,minifigs}
|
||||
```
|
||||
|
||||
2. Create a `.env` file with your configuration:
|
||||
```
|
||||
REBRICKABLE_API_KEY=your_api_key_here
|
||||
DOMAIN_NAME=https://your.domain.com
|
||||
```
|
||||
|
||||
If using locally, set `DOMAIN_NAME` to `http://localhost:3333`.
|
||||
|
||||
3. Deploy with Docker Compose:
|
||||
```bash
|
||||
docker compose up -d
|
||||
```
|
||||
|
||||
4. Access the web interface at `http://localhost:3333`
|
||||
|
||||
5. The database is created, csv files are downloaded and you will be redirected to the `/create` page for inputting a set number.
|
||||
|
||||
## Setup using pre-build Docker image
|
||||
|
||||
1. Setup folders and files:
|
||||
```bash
|
||||
mkdir BrickTracker
|
||||
cd BrickTracker
|
||||
mkdir -p static/{sets,instructions,parts,minifigs}
|
||||
touch app.db
|
||||
```
|
||||
|
||||
2. Create a `.env` file with your configuration:
|
||||
```
|
||||
REBRICKABLE_API_KEY=your_api_key_here
|
||||
DOMAIN_NAME=https://your.domain.com
|
||||
```
|
||||
|
||||
If using locally, set `DOMAIN_NAME` to `http://localhost:3333`.
|
||||
|
||||
3. Create Docker Compose file:
|
||||
```bash
|
||||
services:
|
||||
bricktracker:
|
||||
container_name: BrickTracker
|
||||
restart: unless-stopped
|
||||
image: gitea.baerentsen.space/frederikbaerentsen/bricktracker:latest
|
||||
ports:
|
||||
- "3333:3333"
|
||||
volumes:
|
||||
- ./.env:/app/.env
|
||||
- ./static/parts:/app/static/parts
|
||||
- ./static/instructions:/app/static/instructions
|
||||
- ./static/sets:/app/static/sets
|
||||
- ./app.db:/app/app.db
|
||||
```
|
||||
|
||||
4. Deploy with Docker Compose:
|
||||
```bash
|
||||
docker compose up -d
|
||||
```
|
||||
|
||||
4. Access the web interface at `http://localhost:3333`
|
||||
|
||||
5. The database is created, csv files are downloaded and you will be redirected to the `/create` page for inputting a set number.
|
||||
|
||||
6. csv files are downloaded inside the container. If you delete the container, go to `/config` and redownload them again.
|
||||
|
||||
## Usage
|
||||
|
||||
See [first steps](docs/first-steps.md).
|
||||
### Adding Sets
|
||||
1. Go to the Create page
|
||||
2. Enter a LEGO set number (e.g., "42115")
|
||||
3. Wait for the set to be downloaded and processed
|
||||
|
||||
## Documentation
|
||||
### Managing Sets
|
||||
- Mark sets as checked/collected using the checkboxes
|
||||
- Track missing pieces by entering quantities in the parts table
|
||||
- Note, the checkbox for missing pieces is updated automatically, if the set has missing pieces. It cannot be manually checked off.
|
||||
- View all missing pieces across sets in the Missing page
|
||||
- View complete parts inventory in the Parts page
|
||||
- View all minifigures in the Minifigures page
|
||||
|
||||
Most of the pages should be self explanatory to use.
|
||||
However, you can find more specific documentation in the [documentation](docs/DOCS.md).
|
||||
### Instructions
|
||||
|
||||
You can find screenshots of the application in the [overview](docs/overview.md) documentation file.
|
||||
Instructions can be added to the `static/instructions` folder. Instructions **must** be named:
|
||||
|
||||
- SetNumber.pdf: `10312-1.pdf` or `7001-1.pdf`. Sets with multiple versions (eg. collectible minifigures use `-1`, `-2` etc) like `71039-1.pdf` and `71039-2.pdf`.
|
||||
- SetNumber-pdf_number.pdf: `10294-1-1.pdf`, `10294-1-2.pdf` and `10294-1-3.pdf` for all three PDFs of the `10294-1` set.
|
||||
|
||||
Instructions are not automatically downloaded!
|
||||
|
||||
## Docker Configuration
|
||||
|
||||
The application uses two main configuration files:
|
||||
|
||||
### docker-compose.yml
|
||||
```yaml
|
||||
services:
|
||||
bricktracker:
|
||||
container_name: BrickTracker
|
||||
restart: unless-stopped
|
||||
build: .
|
||||
ports:
|
||||
- "3333:3333"
|
||||
volumes:
|
||||
- .:/app
|
||||
env_file:
|
||||
- .env
|
||||
```
|
||||
|
||||
### Dockerfile
|
||||
```dockerfile
|
||||
FROM python:slim
|
||||
WORKDIR /app
|
||||
COPY requirements.txt .
|
||||
RUN pip install -r requirements.txt
|
||||
COPY . .
|
||||
RUN bash lego.sh
|
||||
CMD ["gunicorn","--bind","0.0.0.0:3333","app:app","--worker-class","eventlet"]
|
||||
```
|
||||
|
||||
## Development
|
||||
|
||||
The application is built with:
|
||||
- Flask (Python web framework)
|
||||
- SQLite (Database)
|
||||
- Socket.IO (Real-time updates)
|
||||
- Rebrickable API (LEGO data)
|
||||
|
||||
Key files:
|
||||
- `app.py`: Main application code
|
||||
- `db.py`: Database operations
|
||||
- `downloadRB.py`: Rebrickable data download utilities
|
||||
|
||||
## Notes
|
||||
|
||||
- The application stores images locally in the `static` directory
|
||||
- Database is stored in `app.db` (SQLite)
|
||||
- LEGO data is cached in CSV files from Rebrickable
|
||||
- Images are downloaded from Rebrickable when entering a set and then stored locally.
|
||||
- The code is AS-IS! I am not a professional programmer and this has been a hobby projects for a long time. Don't expect anything neat!
|
||||
|
||||
## Screenshots
|
||||
|
||||
### Front page
|
||||
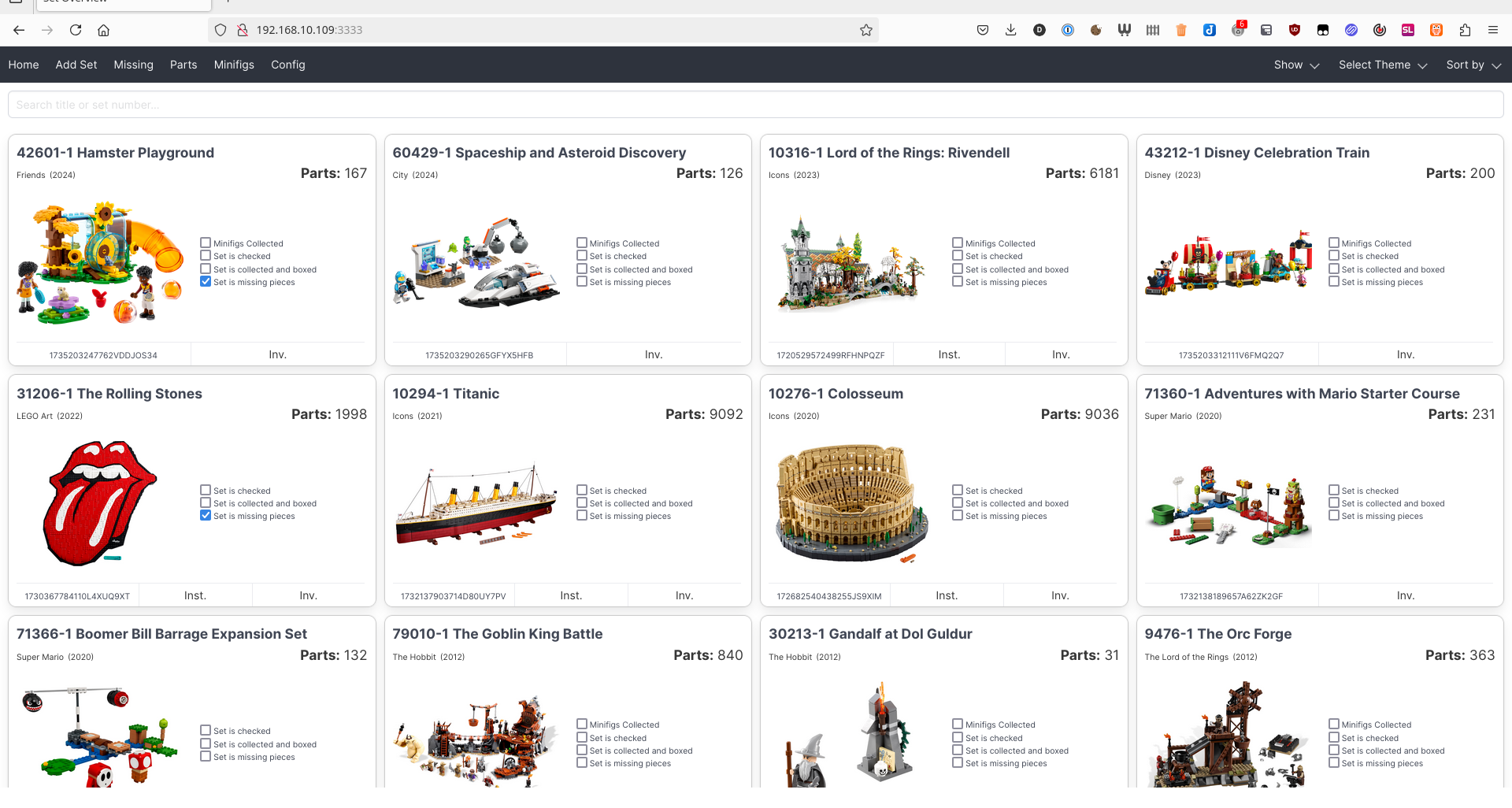
|
||||
|
||||
Search your inventory and sort by theme, year, parts, id, name or sort by missing pieces. If you download instructions as PDF, add them to a specific folder and they show up [under each set](https://xbackbone.baerentsen.space/LaMU8/ZIyIQUdo31.png/raw)
|
||||
|
||||
### Inventory
|
||||
|
||||
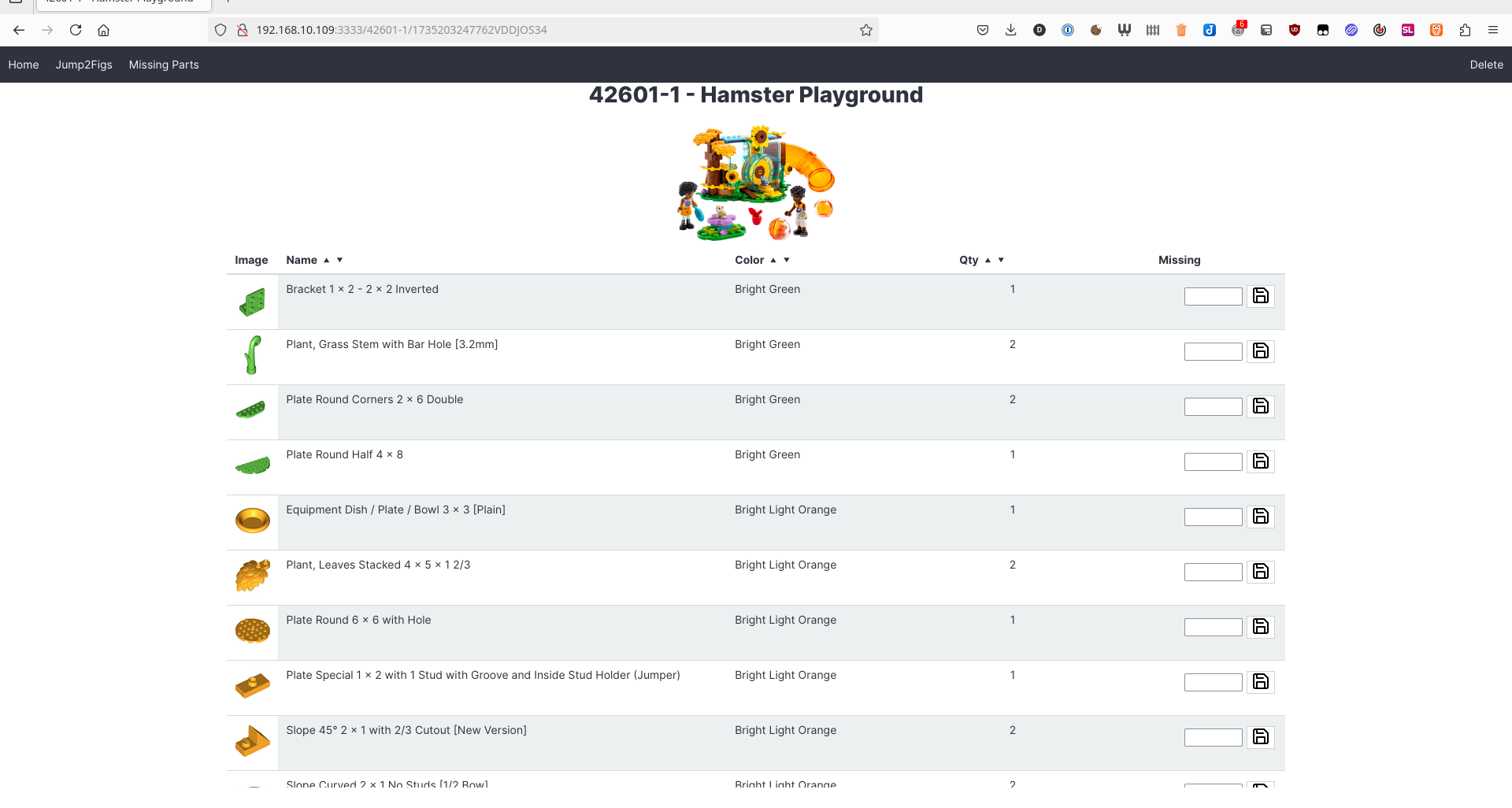
|
||||
|
||||
Filter by color, quantity, name. Add if a piece is missing. Press images to [show them](https://xbackbone.baerentsen.space/LaMU8/FIFOQicE66.png/raw). Filter by only [missing pieces](https://xbackbone.baerentsen.space/LaMU8/LUQeTETA28.png). Minifigures and their parts are listed [at the end](https://xbackbone.baerentsen.space/LaMU8/nEPujImi75.png/raw).
|
||||
|
||||
### Missing pieces
|
||||
|
||||
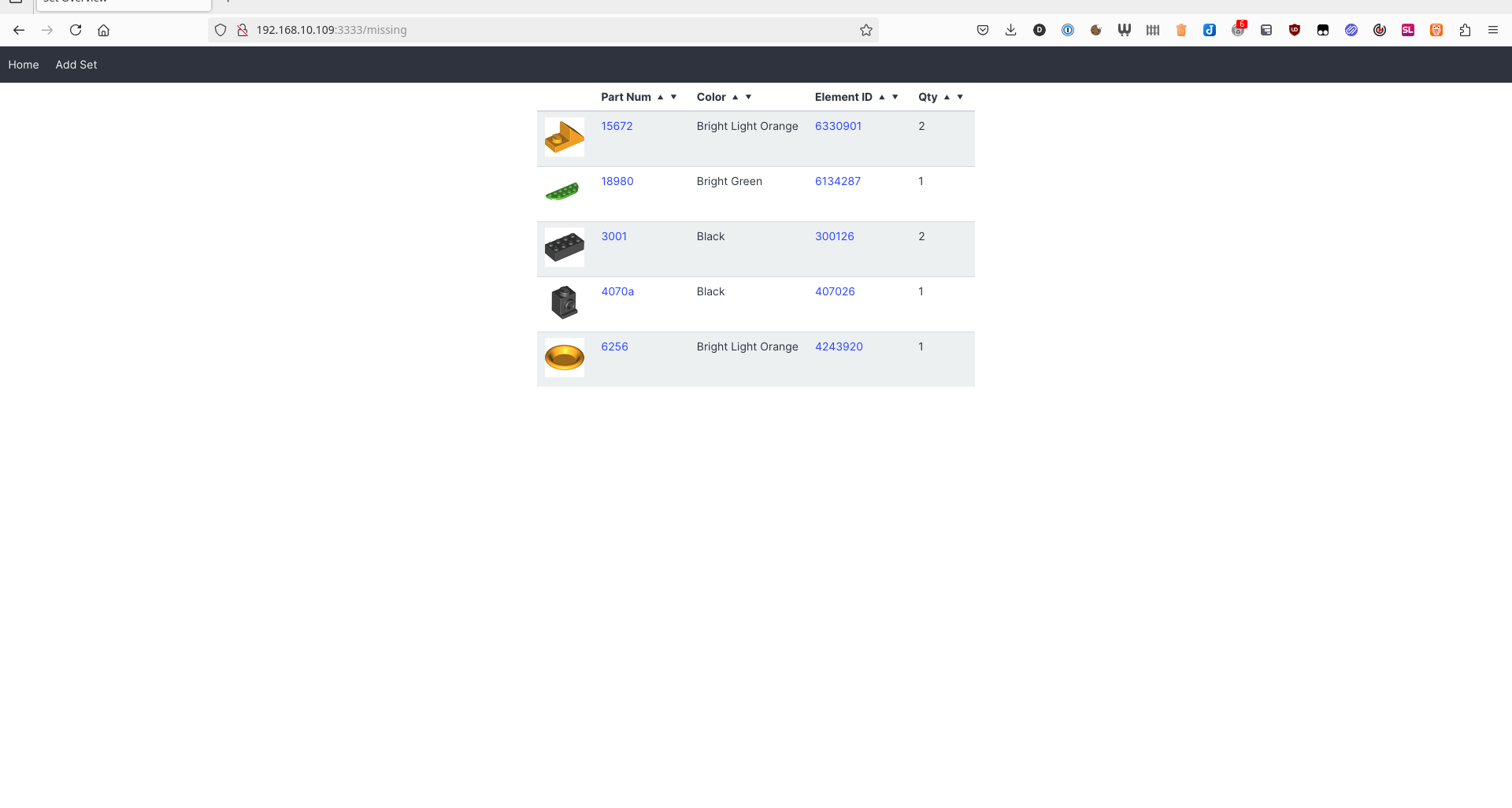
|
||||
|
||||
List of all your missing pieces, with links to bricklink and rebrickable.
|
||||
|
||||
### All parts
|
||||
|
||||
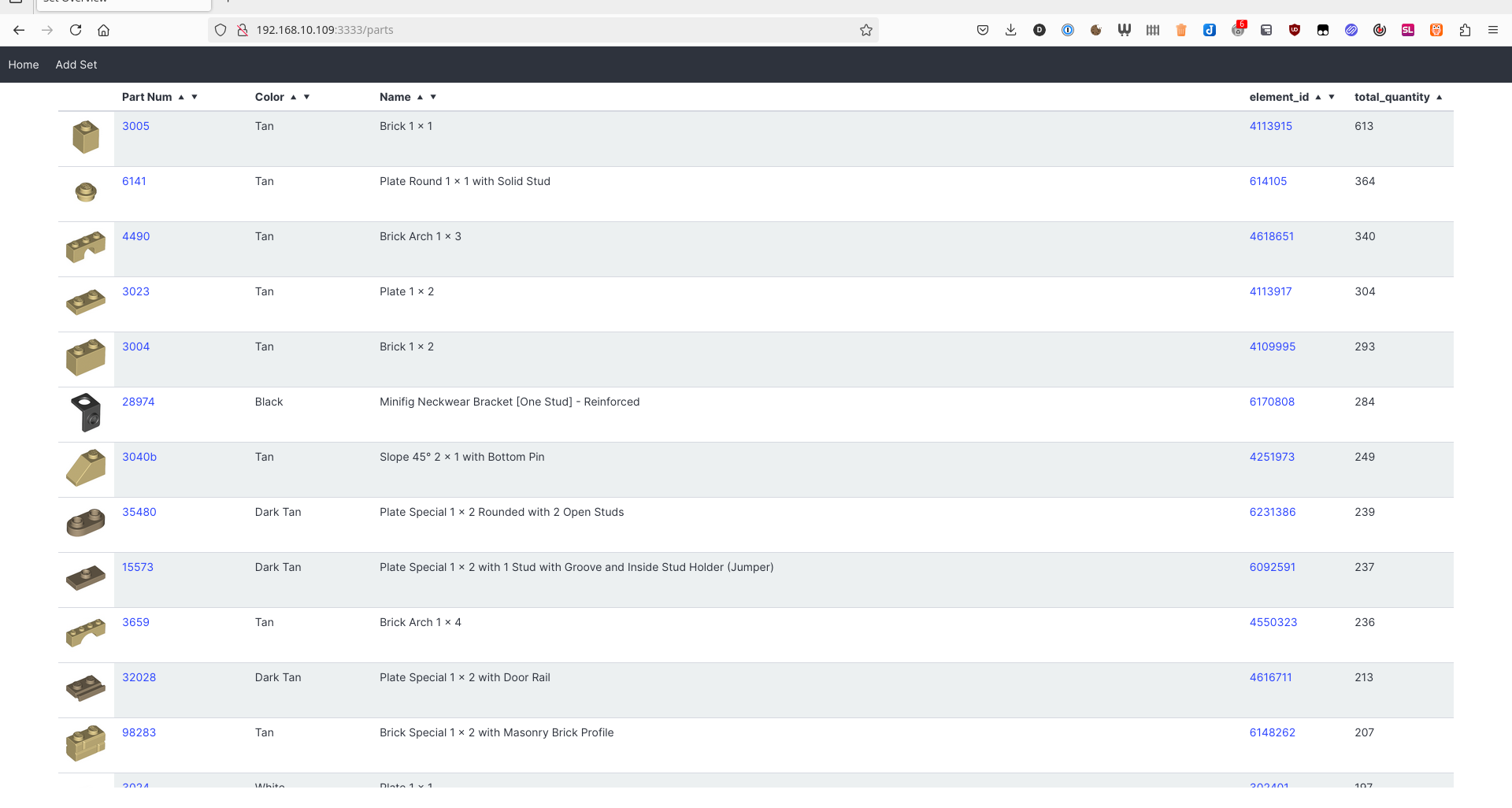
|
||||
List of all parts in your inventory.
|
||||
|
||||
### Minifigures
|
||||
|
||||
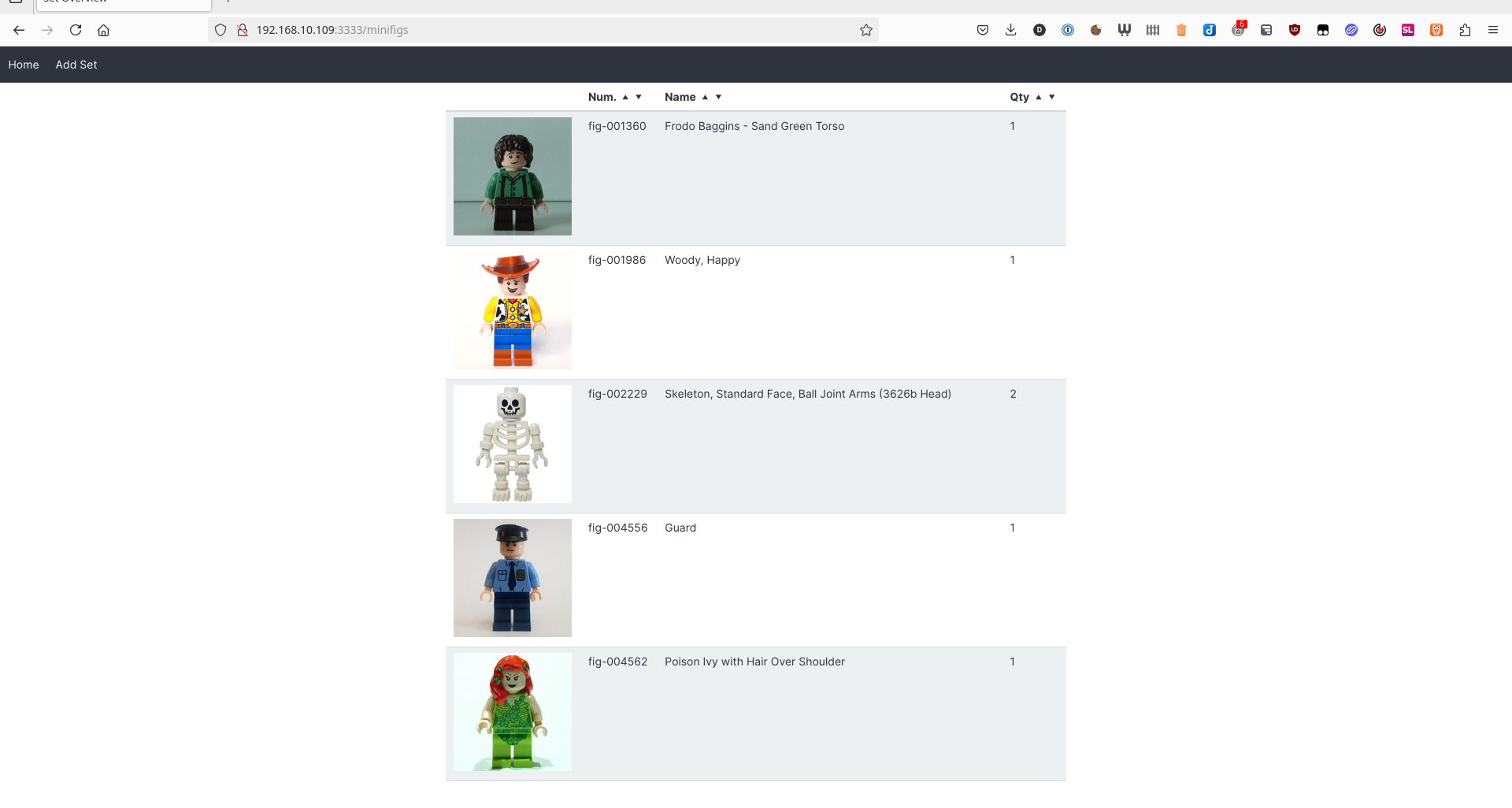
|
||||
|
||||
List of all minifigures in your inventory and quantity.
|
||||
|
||||
### Multiple sets
|
||||
|
||||
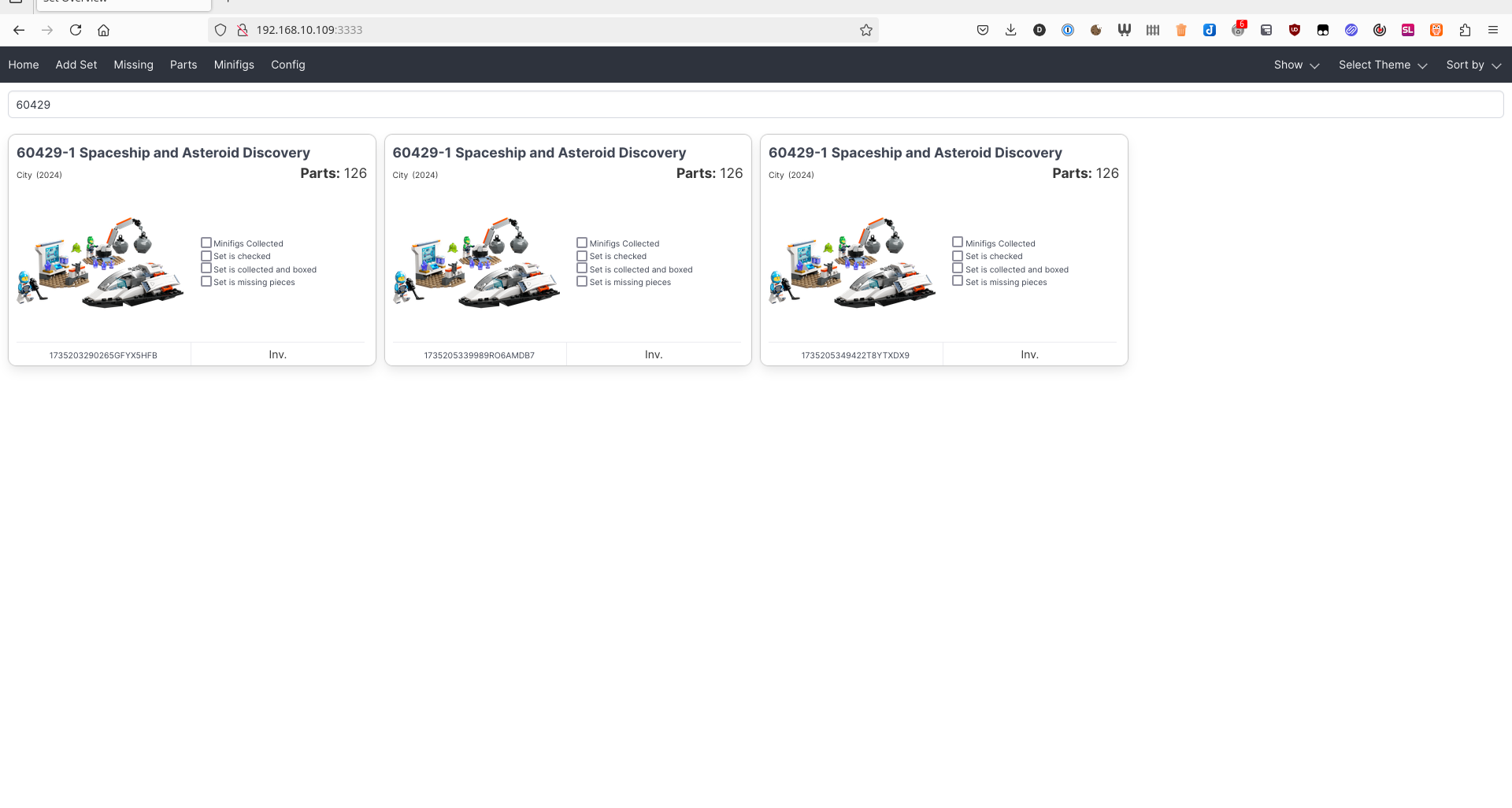
|
||||
|
||||
Each set is given a unique ID, such that you can have multiple copies of a set with different pieces missing in each copy. Sets can also easily be [deleted](https://xbackbone.baerentsen.space/LaMU8/xeroHupE22.png/raw) from the inventory.
|
||||
|
||||
### Add set
|
||||
|
||||
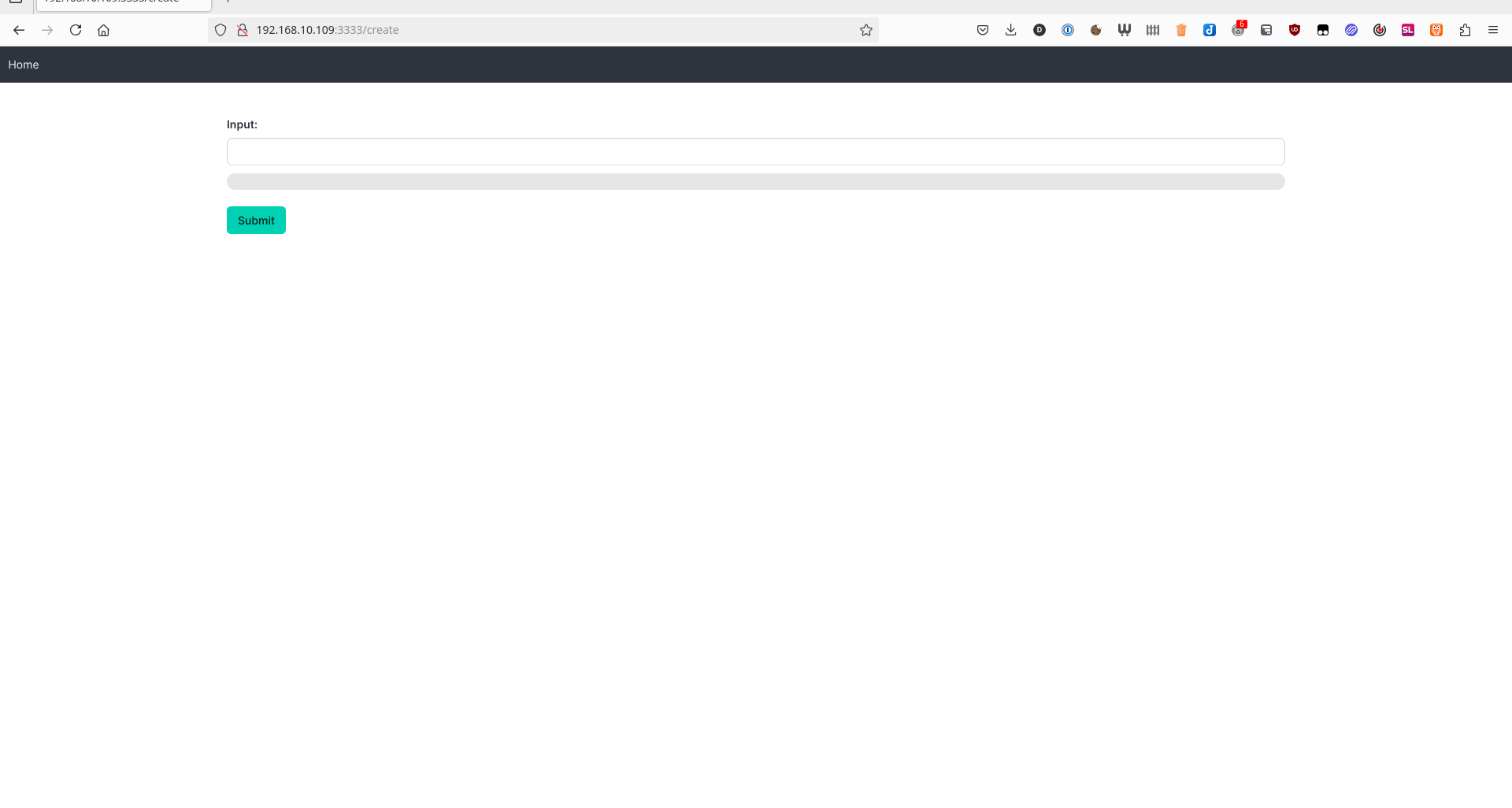
|
||||
|
||||
Sets are added from rebrickable using your own API key. Set numbers are checked against sets.csv from rebrickable and can be updated from the [config page](https://xbackbone.baerentsen.space/LaMU8/lErImaCE12.png/raw). When a set is added, all images from rebrickable are downloaded and stored locally, so if multiple sets contains the same part/color, only one image is downloaded and stored. This also make no calls to rebrickable when just browsing and using the site. Only time rebrickable to used it when up adding a new set.
|
||||
|
||||
### Wishlist
|
||||
|
||||
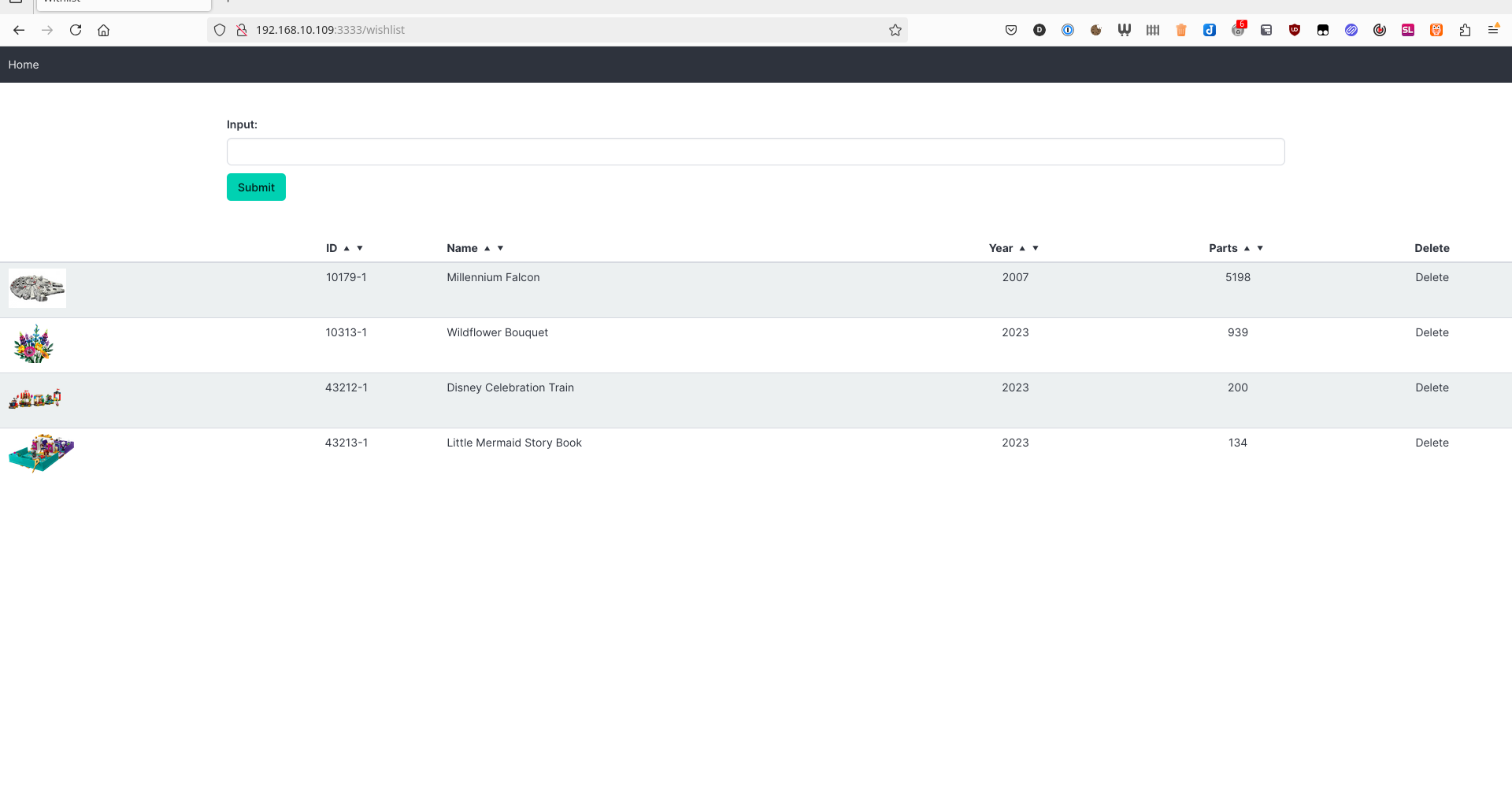
|
||||
|
||||
Sets are added from rebrickable and again checked against sets.csv. If you can't add a brand new set, consider updating your data from the [`/config` page](https://xbackbone.baerentsen.space/LaMU8/lErImaCE12.png/raw). Press the delete button to remove a set. Known Issue: If multiple sets of the same number is added, they will all be deleted.
|
||||
|
193
archive/lego.py
Normal file
193
archive/lego.py
Normal file
@ -0,0 +1,193 @@
|
||||
import sys #using argv
|
||||
import logging #logging errors
|
||||
|
||||
from pathlib import Path # creating folders
|
||||
import rebrick #rebrickable api
|
||||
|
||||
# json things
|
||||
import json
|
||||
from pprint import pprint as pp
|
||||
import requests # request img from web
|
||||
import shutil # save img locally
|
||||
|
||||
log_name='lego.log'
|
||||
|
||||
logging.basicConfig(filename=log_name, level=logging.DEBUG)
|
||||
logging.FileHandler(log_name,mode='w')
|
||||
|
||||
if '-' not in sys.argv[1]:
|
||||
set_num = sys.argv[1] + '-1'
|
||||
else:
|
||||
set_num=sys.argv[1]
|
||||
|
||||
#online_set_num=set_num+"-1"
|
||||
|
||||
print ("Adding set: " + set_num)
|
||||
|
||||
set_path="./static/sets/" + set_num + "/"
|
||||
Path('./static/parts').mkdir(parents=True, exist_ok=True)
|
||||
Path('./static/figs').mkdir(parents=True, exist_ok=True)
|
||||
Path('./info').mkdir(parents=True, exist_ok=True)
|
||||
|
||||
with open('api','r') as f:
|
||||
api_key = f.read().replace('\n','')
|
||||
|
||||
rb = rebrick.init(api_key)
|
||||
|
||||
# if Path(set_path).is_dir():
|
||||
# print('Set exists, exitting')
|
||||
# logging.error('Set exists!')
|
||||
# #exit()
|
||||
|
||||
Path(set_path).mkdir(parents=True, exist_ok=True)
|
||||
|
||||
# Get set info
|
||||
response = json.loads(rebrick.lego.get_set(set_num).read())
|
||||
|
||||
if Path("./info/"+set_num + ".json").is_file():
|
||||
|
||||
ans = input('Set exists, would you like to add another copy (Y/N)?\n')
|
||||
if ans.lower() == 'yes' or ans.lower() == 'y':
|
||||
with open("./info/" + set_num + ".json",'r') as f:
|
||||
data = json.load(f)
|
||||
data['count'] = data['count'] + 1
|
||||
|
||||
tmp = {"location": "","minifigs": "","bricks": {"missing": []}}
|
||||
|
||||
data['unit'].append(tmp)
|
||||
pp(data)
|
||||
with open("./info/" + set_num + ".json",'w') as f:
|
||||
json.dump(data,f,indent = 4)
|
||||
|
||||
with open(set_path+'info.json', 'w', encoding='utf-8') as f:
|
||||
json.dump(response, f, ensure_ascii=False, indent=4)
|
||||
|
||||
# save set image to folder
|
||||
set_img_url = response["set_img_url"]
|
||||
|
||||
print('Saving set image:',end='')
|
||||
|
||||
res = requests.get(set_img_url, stream = True)
|
||||
|
||||
if res.status_code == 200:
|
||||
with open(set_path+"cover.jpg",'wb') as f:
|
||||
shutil.copyfileobj(res.raw, f)
|
||||
print(' OK')
|
||||
else:
|
||||
print('Image Couldn\'t be retrieved for set ' + set_num)
|
||||
logging.error('set_img_url: ' + set_num)
|
||||
print(' ERROR')
|
||||
|
||||
# set inventory
|
||||
print('Saving set inventory')
|
||||
response = json.loads(rebrick.lego.get_set_elements(set_num,page_size=20000).read())
|
||||
with open(set_path+'inventory.json', 'w', encoding='utf-8') as f:
|
||||
json.dump(response, f, ensure_ascii=False, indent=4)
|
||||
|
||||
# get part images if not exists
|
||||
print('Saving part images')
|
||||
for i in response["results"]:
|
||||
try:
|
||||
if i['element_id'] == None:
|
||||
if not Path("./static/parts/p_"+i["part"]["part_id"]+".jpg").is_file():
|
||||
res = requests.get(i["part"]["part_img_url"], stream = True)
|
||||
if res.status_code == 200:
|
||||
with open("./static/parts/p_"+i["part"]["part_id"]+".jpg",'wb') as f:
|
||||
shutil.copyfileobj(res.raw, f)
|
||||
else:
|
||||
if not Path("./static/parts/"+i["element_id"]+".jpg").is_file():
|
||||
res = requests.get(i["part"]["part_img_url"], stream = True)
|
||||
|
||||
if res.status_code == 200:
|
||||
with open("./static/parts/"+i["element_id"]+".jpg",'wb') as f:
|
||||
shutil.copyfileobj(res.raw, f)
|
||||
if not Path("./static/parts/"+i["element_id"]+".jpg").is_file():
|
||||
res = requests.get(i["part"]["part_img_url"], stream = True)
|
||||
|
||||
if res.status_code == 200:
|
||||
with open("./static/parts/"+i["element_id"]+".jpg",'wb') as f:
|
||||
shutil.copyfileobj(res.raw, f)
|
||||
except Exception as e:
|
||||
print(e)
|
||||
logging.error(set_num + ": " + str(e))
|
||||
|
||||
# read info file with missing pieces
|
||||
|
||||
if Path("./info/"+set_num + ".json").is_file():
|
||||
with open("./info/" + set_num + ".json") as f:
|
||||
data = json.load(f)
|
||||
else:
|
||||
shutil.copy("set_template.json", "./info/"+set_num+".json")
|
||||
|
||||
## Minifigs ##
|
||||
print('Savings minifigs')
|
||||
response = json.loads(rebrick.lego.get_set_minifigs(set_num).read())
|
||||
|
||||
figures = {"figs": []}
|
||||
|
||||
for x in response["results"]:
|
||||
print(" " + x["set_name"])
|
||||
fig = {
|
||||
"set_num": x["set_num"],
|
||||
"name": x["set_name"],
|
||||
"quantity": x["quantity"],
|
||||
"set_img_url": x["set_img_url"],
|
||||
"parts": []
|
||||
}
|
||||
|
||||
res = requests.get(x["set_img_url"], stream = True)
|
||||
|
||||
if res.status_code == 200:
|
||||
with open("./static/figs/"+x["set_num"]+".jpg",'wb') as f:
|
||||
shutil.copyfileobj(res.raw, f)
|
||||
else:
|
||||
print('Image Couldn\'t be retrieved for set ' + set_num)
|
||||
logging.error('set_img_url: ' + set_num)
|
||||
|
||||
response = json.loads(rebrick.lego.get_minifig_elements(x["set_num"]).read())
|
||||
|
||||
for i in response["results"]:
|
||||
part = {
|
||||
"name": i["part"]["name"],
|
||||
"quantity": i["quantity"],
|
||||
"color_name": i["color"]["name"],
|
||||
"part_num": i["part"]["part_num"],
|
||||
"part_img_url": i["part"]["part_img_url"]
|
||||
|
||||
}
|
||||
fig["parts"].append(part)
|
||||
|
||||
try:
|
||||
if i['element_id'] == None:
|
||||
if not Path("./static/figs/p_"+i["part"]["part_num"]+".jpg").is_file():
|
||||
res = requests.get(i["part"]["part_img_url"], stream = True)
|
||||
|
||||
if res.status_code == 200:
|
||||
with open("./static/figs/p_"+i["part"]["part_num"]+".jpg",'wb') as f:
|
||||
shutil.copyfileobj(res.raw, f)
|
||||
else:
|
||||
if not Path("./static/figs/"+i["part"]["part_num"]+".jpg").is_file():
|
||||
res = requests.get(i["part"]["part_img_url"], stream = True)
|
||||
|
||||
if res.status_code == 200:
|
||||
with open("./static/figs/"+i["part"]["part_num"]+".jpg",'wb') as f:
|
||||
shutil.copyfileobj(res.raw, f)
|
||||
if not Path("./static/figs/"+i["part"]["part_num"]+".jpg").is_file():
|
||||
res = requests.get(i["part"]["part_img_url"], stream = True)
|
||||
|
||||
if res.status_code == 200:
|
||||
with open("./static/figs/"+i["part"]["part_num"]+".jpg",'wb') as f:
|
||||
shutil.copyfileobj(res.raw, f)
|
||||
except Exception as e:
|
||||
print(e)
|
||||
logging.error(set_num + ": " + str(e))
|
||||
|
||||
figures["figs"].append(fig)
|
||||
part = {}
|
||||
fig = {}
|
||||
|
||||
with open(set_path+'minifigs.json', 'w', encoding='utf-8') as f:
|
||||
json.dump(figures, f, ensure_ascii=False, indent=4)
|
||||
####
|
||||
|
||||
print('Done!')
|
12
archive/set_template.json
Normal file
12
archive/set_template.json
Normal file
@ -0,0 +1,12 @@
|
||||
{
|
||||
"count": 1,
|
||||
"unit": [
|
||||
{
|
||||
"location": "",
|
||||
"minifigs": "",
|
||||
"bricks": {
|
||||
"missing": []
|
||||
}
|
||||
}
|
||||
]
|
||||
}
|
@ -1 +0,0 @@
|
||||
VERSION = '0.1.0'
|
@ -1,127 +0,0 @@
|
||||
import logging
|
||||
import sys
|
||||
import time
|
||||
from zoneinfo import ZoneInfo
|
||||
|
||||
from flask import current_app, Flask, g
|
||||
from werkzeug.middleware.proxy_fix import ProxyFix
|
||||
|
||||
from bricktracker.configuration_list import BrickConfigurationList
|
||||
from bricktracker.login import LoginManager
|
||||
from bricktracker.navbar import Navbar
|
||||
from bricktracker.sql import close
|
||||
from bricktracker.version import __version__
|
||||
from bricktracker.views.add import add_page
|
||||
from bricktracker.views.admin.admin import admin_page
|
||||
from bricktracker.views.admin.database import admin_database_page
|
||||
from bricktracker.views.admin.image import admin_image_page
|
||||
from bricktracker.views.admin.instructions import admin_instructions_page
|
||||
from bricktracker.views.admin.owner import admin_owner_page
|
||||
from bricktracker.views.admin.purchase_location import admin_purchase_location_page # noqa: E501
|
||||
from bricktracker.views.admin.retired import admin_retired_page
|
||||
from bricktracker.views.admin.set import admin_set_page
|
||||
from bricktracker.views.admin.status import admin_status_page
|
||||
from bricktracker.views.admin.storage import admin_storage_page
|
||||
from bricktracker.views.admin.tag import admin_tag_page
|
||||
from bricktracker.views.admin.theme import admin_theme_page
|
||||
from bricktracker.views.error import error_404
|
||||
from bricktracker.views.index import index_page
|
||||
from bricktracker.views.instructions import instructions_page
|
||||
from bricktracker.views.login import login_page
|
||||
from bricktracker.views.minifigure import minifigure_page
|
||||
from bricktracker.views.part import part_page
|
||||
from bricktracker.views.set import set_page
|
||||
from bricktracker.views.storage import storage_page
|
||||
from bricktracker.views.wish import wish_page
|
||||
|
||||
|
||||
def setup_app(app: Flask) -> None:
|
||||
# Load the configuration
|
||||
BrickConfigurationList(app)
|
||||
|
||||
# Set the logging level
|
||||
if app.config['DEBUG']:
|
||||
logging.basicConfig(
|
||||
stream=sys.stdout,
|
||||
level=logging.DEBUG,
|
||||
format='[%(asctime)s] {%(filename)s:%(lineno)d} %(levelname)s - %(message)s', # noqa: E501
|
||||
)
|
||||
else:
|
||||
logging.basicConfig(
|
||||
stream=sys.stdout,
|
||||
level=logging.INFO,
|
||||
format='[%(asctime)s] %(levelname)s - %(message)s',
|
||||
)
|
||||
|
||||
# Load the navbar
|
||||
Navbar(app)
|
||||
|
||||
# Setup the login manager
|
||||
LoginManager(app)
|
||||
|
||||
# I don't know :-)
|
||||
app.wsgi_app = ProxyFix(
|
||||
app.wsgi_app,
|
||||
x_for=1,
|
||||
x_proto=1,
|
||||
x_host=1,
|
||||
x_port=1,
|
||||
x_prefix=1,
|
||||
)
|
||||
|
||||
# Register errors
|
||||
app.register_error_handler(404, error_404)
|
||||
|
||||
# Register app routes
|
||||
app.register_blueprint(add_page)
|
||||
app.register_blueprint(index_page)
|
||||
app.register_blueprint(instructions_page)
|
||||
app.register_blueprint(login_page)
|
||||
app.register_blueprint(minifigure_page)
|
||||
app.register_blueprint(part_page)
|
||||
app.register_blueprint(set_page)
|
||||
app.register_blueprint(storage_page)
|
||||
app.register_blueprint(wish_page)
|
||||
|
||||
# Register admin routes
|
||||
app.register_blueprint(admin_page)
|
||||
app.register_blueprint(admin_database_page)
|
||||
app.register_blueprint(admin_image_page)
|
||||
app.register_blueprint(admin_instructions_page)
|
||||
app.register_blueprint(admin_retired_page)
|
||||
app.register_blueprint(admin_owner_page)
|
||||
app.register_blueprint(admin_purchase_location_page)
|
||||
app.register_blueprint(admin_set_page)
|
||||
app.register_blueprint(admin_status_page)
|
||||
app.register_blueprint(admin_storage_page)
|
||||
app.register_blueprint(admin_tag_page)
|
||||
app.register_blueprint(admin_theme_page)
|
||||
|
||||
# An helper to make global variables available to the
|
||||
# request
|
||||
@app.before_request
|
||||
def before_request() -> None:
|
||||
def request_time() -> str:
|
||||
elapsed = time.time() - g.request_start_time
|
||||
if elapsed < 1:
|
||||
return '{elapsed:.0f}ms'.format(elapsed=elapsed*1000)
|
||||
else:
|
||||
return '{elapsed:.2f}s'.format(elapsed=elapsed)
|
||||
|
||||
# Login manager
|
||||
g.login = LoginManager
|
||||
|
||||
# Execution time
|
||||
g.request_start_time = time.time()
|
||||
g.request_time = request_time
|
||||
|
||||
# Register the timezone
|
||||
g.timezone = ZoneInfo(current_app.config['TIMEZONE'])
|
||||
|
||||
# Version
|
||||
g.version = __version__
|
||||
|
||||
# Make sure all connections are closed at the end
|
||||
@app.teardown_request
|
||||
def teardown_request(_: BaseException | None) -> None:
|
||||
close()
|
@ -1,72 +0,0 @@
|
||||
from typing import Any, Final
|
||||
|
||||
# Configuration map:
|
||||
# - n: internal name (str)
|
||||
# - e: extra environment name (str, optional=None)
|
||||
# - d: default value (Any, optional=None)
|
||||
# - c: cast to type (Type, optional=None)
|
||||
# - s: interpret as a path within static (bool, optional=False)
|
||||
# Easy to change an environment variable name without changing all the code
|
||||
CONFIG: Final[list[dict[str, Any]]] = [
|
||||
{'n': 'AUTHENTICATION_PASSWORD', 'd': ''},
|
||||
{'n': 'AUTHENTICATION_KEY', 'd': ''},
|
||||
{'n': 'BRICKLINK_LINK_PART_PATTERN', 'd': 'https://www.bricklink.com/v2/catalog/catalogitem.page?P={part}'}, # noqa: E501
|
||||
{'n': 'BRICKLINK_LINKS', 'c': bool},
|
||||
{'n': 'DATABASE_PATH', 'd': './app.db'},
|
||||
{'n': 'DATABASE_TIMESTAMP_FORMAT', 'd': '%Y-%m-%d-%H-%M-%S'},
|
||||
{'n': 'DEBUG', 'c': bool},
|
||||
{'n': 'DEFAULT_TABLE_PER_PAGE', 'd': 25, 'c': int},
|
||||
{'n': 'DOMAIN_NAME', 'e': 'DOMAIN_NAME', 'd': ''},
|
||||
{'n': 'FILE_DATETIME_FORMAT', 'd': '%d/%m/%Y, %H:%M:%S'},
|
||||
{'n': 'HOST', 'd': '0.0.0.0'},
|
||||
{'n': 'INDEPENDENT_ACCORDIONS', 'c': bool},
|
||||
{'n': 'INSTRUCTIONS_ALLOWED_EXTENSIONS', 'd': ['.pdf'], 'c': list}, # noqa: E501
|
||||
{'n': 'INSTRUCTIONS_FOLDER', 'd': 'instructions', 's': True},
|
||||
{'n': 'HIDE_ADD_SET', 'c': bool},
|
||||
{'n': 'HIDE_ADD_BULK_SET', 'c': bool},
|
||||
{'n': 'HIDE_ADMIN', 'c': bool},
|
||||
{'n': 'HIDE_ALL_INSTRUCTIONS', 'c': bool},
|
||||
{'n': 'HIDE_ALL_MINIFIGURES', 'c': bool},
|
||||
{'n': 'HIDE_ALL_PARTS', 'c': bool},
|
||||
{'n': 'HIDE_ALL_PROBLEMS_PARTS', 'e': 'BK_HIDE_MISSING_PARTS', 'c': bool},
|
||||
{'n': 'HIDE_ALL_SETS', 'c': bool},
|
||||
{'n': 'HIDE_ALL_STORAGES', 'c': bool},
|
||||
{'n': 'HIDE_SET_INSTRUCTIONS', 'c': bool},
|
||||
{'n': 'HIDE_TABLE_DAMAGED_PARTS', 'c': bool},
|
||||
{'n': 'HIDE_TABLE_MISSING_PARTS', 'c': bool},
|
||||
{'n': 'HIDE_WISHES', 'c': bool},
|
||||
{'n': 'MINIFIGURES_DEFAULT_ORDER', 'd': '"rebrickable_minifigures"."name" ASC'}, # noqa: E501
|
||||
{'n': 'MINIFIGURES_FOLDER', 'd': 'minifigs', 's': True},
|
||||
{'n': 'NO_THREADED_SOCKET', 'c': bool},
|
||||
{'n': 'PARTS_DEFAULT_ORDER', 'd': '"rebrickable_parts"."name" ASC, "rebrickable_parts"."color_name" ASC, "bricktracker_parts"."spare" ASC'}, # noqa: E501
|
||||
{'n': 'PARTS_FOLDER', 'd': 'parts', 's': True},
|
||||
{'n': 'PORT', 'd': 3333, 'c': int},
|
||||
{'n': 'PURCHASE_DATE_FORMAT', 'd': '%d/%m/%Y'},
|
||||
{'n': 'PURCHASE_CURRENCY', 'd': '€'},
|
||||
{'n': 'PURCHASE_LOCATION_DEFAULT_ORDER', 'd': '"bricktracker_metadata_purchase_locations"."name" ASC'}, # noqa: E501
|
||||
{'n': 'RANDOM', 'e': 'RANDOM', 'c': bool},
|
||||
{'n': 'REBRICKABLE_API_KEY', 'e': 'REBRICKABLE_API_KEY', 'd': ''},
|
||||
{'n': 'REBRICKABLE_IMAGE_NIL', 'd': 'https://rebrickable.com/static/img/nil.png'}, # noqa: E501
|
||||
{'n': 'REBRICKABLE_IMAGE_NIL_MINIFIGURE', 'd': 'https://rebrickable.com/static/img/nil_mf.jpg'}, # noqa: E501
|
||||
{'n': 'REBRICKABLE_LINK_MINIFIGURE_PATTERN', 'd': 'https://rebrickable.com/minifigs/{figure}'}, # noqa: E501
|
||||
{'n': 'REBRICKABLE_LINK_PART_PATTERN', 'd': 'https://rebrickable.com/parts/{part}/_/{color}'}, # noqa: E501
|
||||
{'n': 'REBRICKABLE_LINK_INSTRUCTIONS_PATTERN', 'd': 'https://rebrickable.com/instructions/{path}'}, # noqa: E501
|
||||
{'n': 'REBRICKABLE_USER_AGENT', 'd': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'}, # noqa: E501
|
||||
{'n': 'REBRICKABLE_LINKS', 'e': 'LINKS', 'c': bool},
|
||||
{'n': 'REBRICKABLE_PAGE_SIZE', 'd': 100, 'c': int},
|
||||
{'n': 'RETIRED_SETS_FILE_URL', 'd': 'https://docs.google.com/spreadsheets/d/1rlYfEXtNKxUOZt2Mfv0H17DvK7bj6Pe0CuYwq6ay8WA/gviz/tq?tqx=out:csv&sheet=Sorted%20by%20Retirement%20Date'}, # noqa: E501
|
||||
{'n': 'RETIRED_SETS_PATH', 'd': './retired_sets.csv'},
|
||||
{'n': 'SETS_DEFAULT_ORDER', 'd': '"rebrickable_sets"."number" DESC, "rebrickable_sets"."version" ASC'}, # noqa: E501
|
||||
{'n': 'SETS_FOLDER', 'd': 'sets', 's': True},
|
||||
{'n': 'SHOW_GRID_FILTERS', 'c': bool},
|
||||
{'n': 'SHOW_GRID_SORT', 'c': bool},
|
||||
{'n': 'SKIP_SPARE_PARTS', 'c': bool},
|
||||
{'n': 'SOCKET_NAMESPACE', 'd': 'bricksocket'},
|
||||
{'n': 'SOCKET_PATH', 'd': '/bricksocket/'},
|
||||
{'n': 'STORAGE_DEFAULT_ORDER', 'd': '"bricktracker_metadata_storages"."name" ASC'}, # noqa: E501
|
||||
{'n': 'THEMES_FILE_URL', 'd': 'https://cdn.rebrickable.com/media/downloads/themes.csv.gz'}, # noqa: E501
|
||||
{'n': 'THEMES_PATH', 'd': './themes.csv'},
|
||||
{'n': 'TIMEZONE', 'd': 'Etc/UTC'},
|
||||
{'n': 'USE_REMOTE_IMAGES', 'c': bool},
|
||||
{'n': 'WISHES_DEFAULT_ORDER', 'd': '"bricktracker_wishes"."rowid" DESC'},
|
||||
]
|
@ -1,89 +0,0 @@
|
||||
import os
|
||||
from typing import Any, Type
|
||||
|
||||
|
||||
# Configuration item
|
||||
class BrickConfiguration(object):
|
||||
name: str
|
||||
cast: Type | None
|
||||
default: Any
|
||||
env_name: str
|
||||
extra_name: str | None
|
||||
mandatory: bool
|
||||
static_path: bool
|
||||
value: Any
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
n: str,
|
||||
e: str | None = None,
|
||||
d: Any = None,
|
||||
c: Type | None = None,
|
||||
m: bool = False,
|
||||
s: bool = False,
|
||||
):
|
||||
# We prefix all default variable name with 'BK_' for the
|
||||
# environment name to avoid interfering
|
||||
self.name = n
|
||||
self.env_name = 'BK_{name}'.format(name=n)
|
||||
self.extra_name = e
|
||||
self.default = d
|
||||
self.cast = c
|
||||
self.mandatory = m
|
||||
self.static_path = s
|
||||
|
||||
# Default for our booleans is False
|
||||
if self.cast == bool:
|
||||
self.default = False
|
||||
|
||||
# Try default environment name
|
||||
value = os.getenv(self.env_name)
|
||||
if value is None:
|
||||
# Try the extra name
|
||||
if self.extra_name is not None:
|
||||
value = os.getenv(self.extra_name)
|
||||
|
||||
# Set the default
|
||||
if value is None:
|
||||
value = self.default
|
||||
|
||||
# Special treatment
|
||||
if value is not None:
|
||||
# Comma seperated list
|
||||
if self.cast == list and isinstance(value, str):
|
||||
value = [item.strip() for item in value.split(',')]
|
||||
self.cast = None
|
||||
|
||||
# Boolean string
|
||||
if self.cast == bool and isinstance(value, str):
|
||||
value = value.lower() in ('true', 'yes', '1')
|
||||
|
||||
# Static path fixup
|
||||
if self.static_path and isinstance(value, str):
|
||||
value = os.path.normpath(value)
|
||||
|
||||
# Remove any leading slash or dots
|
||||
value = value.lstrip('/.')
|
||||
|
||||
# Remove static prefix
|
||||
value = value.removeprefix('static/')
|
||||
|
||||
# Type casting
|
||||
if self.cast is not None:
|
||||
self.value = self.cast(value)
|
||||
else:
|
||||
self.value = value
|
||||
|
||||
# Tells whether the value is changed from its default
|
||||
def is_changed(self, /) -> bool:
|
||||
return self.value != self.default
|
||||
|
||||
# Tells whether the value is secret
|
||||
def is_secret(self, /) -> bool:
|
||||
return self.name in [
|
||||
'REBRICKABLE_API_KEY',
|
||||
'AUTHENTICATION_PASSWORD',
|
||||
'AUTHENTICATION_KEY'
|
||||
]
|
@ -1,60 +0,0 @@
|
||||
import logging
|
||||
from typing import Generator
|
||||
|
||||
from flask import Flask
|
||||
|
||||
from .config import CONFIG
|
||||
from .configuration import BrickConfiguration
|
||||
from .exceptions import ConfigurationMissingException
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
# Application configuration
|
||||
class BrickConfigurationList(object):
|
||||
app: Flask
|
||||
configurations: dict[str, BrickConfiguration]
|
||||
|
||||
# Load configuration
|
||||
def __init__(self, app: Flask, /):
|
||||
self.app = app
|
||||
|
||||
# Load the configurations only there is none already loaded
|
||||
configurations = getattr(self, 'configurations', None)
|
||||
|
||||
if configurations is None:
|
||||
logger.info('Loading configuration variables')
|
||||
|
||||
BrickConfigurationList.configurations = {}
|
||||
|
||||
# Process all configuration items
|
||||
for config in CONFIG:
|
||||
item = BrickConfiguration(**config)
|
||||
|
||||
# Store in the list
|
||||
BrickConfigurationList.configurations[item.name] = item
|
||||
|
||||
# Only store the value in the app to avoid breaking any
|
||||
# existing variables
|
||||
self.app.config[item.name] = item.value
|
||||
|
||||
# Check whether a str configuration is set
|
||||
@staticmethod
|
||||
def error_unless_is_set(name: str):
|
||||
configuration = BrickConfigurationList.configurations[name]
|
||||
|
||||
if configuration.value is None or configuration.value == '':
|
||||
raise ConfigurationMissingException(
|
||||
'{name} must be defined (using the {environ} environment variable)'.format( # noqa: E501
|
||||
name=name,
|
||||
environ=configuration.env_name
|
||||
),
|
||||
)
|
||||
|
||||
# Get all the configuration items from the app config
|
||||
@staticmethod
|
||||
def list() -> Generator[BrickConfiguration, None, None]:
|
||||
keys = sorted(BrickConfigurationList.configurations.keys())
|
||||
|
||||
for name in keys:
|
||||
yield BrickConfigurationList.configurations[name]
|
@ -1,23 +0,0 @@
|
||||
# Something was not found
|
||||
class NotFoundException(Exception):
|
||||
...
|
||||
|
||||
|
||||
# Generic error exception
|
||||
class ErrorException(Exception):
|
||||
title: str = 'Error'
|
||||
|
||||
|
||||
# Configuration error
|
||||
class ConfigurationMissingException(ErrorException):
|
||||
title: str = 'Configuration missing'
|
||||
|
||||
|
||||
# Database error
|
||||
class DatabaseException(ErrorException):
|
||||
title: str = 'Database error'
|
||||
|
||||
|
||||
# Download error
|
||||
class DownloadException(ErrorException):
|
||||
title: str = 'Download error'
|
@ -1,13 +0,0 @@
|
||||
from typing import Any
|
||||
|
||||
|
||||
# SQLite record fields
|
||||
class BrickRecordFields(object):
|
||||
def __getattr__(self, name: str, /) -> Any:
|
||||
if name not in self.__dict__:
|
||||
raise AttributeError(name)
|
||||
|
||||
return self.__dict__[name]
|
||||
|
||||
def __setattr__(self, name: str, value: Any, /) -> None:
|
||||
self.__dict__[name] = value
|
@ -1,289 +0,0 @@
|
||||
from datetime import datetime, timezone
|
||||
import logging
|
||||
import os
|
||||
from shutil import copyfileobj
|
||||
import traceback
|
||||
from typing import Tuple, TYPE_CHECKING
|
||||
|
||||
from bs4 import BeautifulSoup
|
||||
from flask import current_app, g, url_for
|
||||
import humanize
|
||||
import requests
|
||||
from werkzeug.datastructures import FileStorage
|
||||
from werkzeug.utils import secure_filename
|
||||
|
||||
from .exceptions import ErrorException, DownloadException
|
||||
if TYPE_CHECKING:
|
||||
from .rebrickable_set import RebrickableSet
|
||||
from .socket import BrickSocket
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
class BrickInstructions(object):
|
||||
socket: 'BrickSocket'
|
||||
|
||||
allowed: bool
|
||||
rebrickable: 'RebrickableSet | None'
|
||||
extension: str
|
||||
filename: str
|
||||
mtime: datetime
|
||||
set: 'str | None'
|
||||
name: str
|
||||
size: int
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
file: os.DirEntry | str,
|
||||
/,
|
||||
*,
|
||||
socket: 'BrickSocket | None' = None,
|
||||
):
|
||||
# Save the socket
|
||||
if socket is not None:
|
||||
self.socket = socket
|
||||
|
||||
if isinstance(file, str):
|
||||
self.filename = file
|
||||
|
||||
if self.filename == '':
|
||||
raise ErrorException('An instruction filename cannot be empty')
|
||||
else:
|
||||
self.filename = file.name
|
||||
|
||||
# Store the file stats
|
||||
stat = file.stat()
|
||||
self.size = stat.st_size
|
||||
self.mtime = datetime.fromtimestamp(stat.st_mtime, tz=timezone.utc)
|
||||
|
||||
# Store the name and extension, check if extension is allowed
|
||||
self.name, self.extension = os.path.splitext(self.filename)
|
||||
self.extension = self.extension.lower()
|
||||
self.allowed = self.extension in current_app.config['INSTRUCTIONS_ALLOWED_EXTENSIONS'] # noqa: E501
|
||||
|
||||
# Placeholder
|
||||
self.rebrickable = None
|
||||
self.set = None
|
||||
|
||||
# Extract the set number
|
||||
if self.allowed:
|
||||
# Normalize special chars to improve set detection
|
||||
normalized = self.name.replace('_', '-')
|
||||
normalized = normalized.replace(' ', '-')
|
||||
|
||||
splits = normalized.split('-', 2)
|
||||
|
||||
if len(splits) >= 2:
|
||||
try:
|
||||
# Trying to make sense of each part as integers
|
||||
int(splits[0])
|
||||
int(splits[1])
|
||||
|
||||
self.set = '-'.join(splits[:2])
|
||||
except Exception:
|
||||
pass
|
||||
|
||||
# Delete an instruction file
|
||||
def delete(self, /) -> None:
|
||||
os.remove(self.path())
|
||||
|
||||
# Download an instruction file
|
||||
def download(self, path: str, /) -> None:
|
||||
try:
|
||||
# Just to make sure that the progress is initiated
|
||||
self.socket.progress(
|
||||
message='Downloading {file}'.format(
|
||||
file=self.filename,
|
||||
)
|
||||
)
|
||||
|
||||
target = self.path(filename=secure_filename(self.filename))
|
||||
|
||||
# Skipping rather than failing here
|
||||
if os.path.isfile(target):
|
||||
self.socket.complete(
|
||||
message='File {file} already exists, skipped'.format(
|
||||
file=self.filename,
|
||||
)
|
||||
)
|
||||
|
||||
else:
|
||||
url = current_app.config['REBRICKABLE_LINK_INSTRUCTIONS_PATTERN'].format( # noqa: E501
|
||||
path=path
|
||||
)
|
||||
trimmed_url = current_app.config['REBRICKABLE_LINK_INSTRUCTIONS_PATTERN'].format( # noqa: E501
|
||||
path=path.partition('/')[0]
|
||||
)
|
||||
|
||||
# Request the file
|
||||
self.socket.progress(
|
||||
message='Requesting {url}'.format(
|
||||
url=trimmed_url,
|
||||
)
|
||||
)
|
||||
|
||||
response = requests.get(url, stream=True)
|
||||
if response.ok:
|
||||
|
||||
# Store the content header as size
|
||||
try:
|
||||
self.size = int(
|
||||
response.headers.get('Content-length', 0)
|
||||
)
|
||||
except Exception:
|
||||
self.size = 0
|
||||
|
||||
# Downloading the file
|
||||
self.socket.progress(
|
||||
message='Downloading {url} ({size})'.format(
|
||||
url=trimmed_url,
|
||||
size=self.human_size(),
|
||||
)
|
||||
)
|
||||
|
||||
with open(target, 'wb') as f:
|
||||
copyfileobj(response.raw, f)
|
||||
else:
|
||||
raise DownloadException('failed to download: {code}'.format( # noqa: E501
|
||||
code=response.status_code
|
||||
))
|
||||
|
||||
# Info
|
||||
logger.info('The instruction file {file} has been downloaded'.format( # noqa: E501
|
||||
file=self.filename
|
||||
))
|
||||
|
||||
# Complete
|
||||
self.socket.complete(
|
||||
message='File {file} downloaded ({size})'.format( # noqa: E501
|
||||
file=self.filename,
|
||||
size=self.human_size()
|
||||
)
|
||||
)
|
||||
|
||||
except Exception as e:
|
||||
self.socket.fail(
|
||||
message='Error while downloading instruction {file}: {error}'.format( # noqa: E501
|
||||
file=self.filename,
|
||||
error=e,
|
||||
)
|
||||
)
|
||||
|
||||
logger.debug(traceback.format_exc())
|
||||
|
||||
# Display the size in a human format
|
||||
def human_size(self) -> str:
|
||||
return humanize.naturalsize(self.size)
|
||||
|
||||
# Display the time in a human format
|
||||
def human_time(self) -> str:
|
||||
return self.mtime.astimezone(g.timezone).strftime(
|
||||
current_app.config['FILE_DATETIME_FORMAT']
|
||||
)
|
||||
|
||||
# Compute the path of an instruction file
|
||||
def path(self, /, *, filename=None) -> str:
|
||||
if filename is None:
|
||||
filename = self.filename
|
||||
|
||||
return os.path.join(
|
||||
current_app.static_folder, # type: ignore
|
||||
current_app.config['INSTRUCTIONS_FOLDER'],
|
||||
filename
|
||||
)
|
||||
|
||||
# Rename an instructions file
|
||||
def rename(self, filename: str, /) -> None:
|
||||
# Add the extension
|
||||
filename = '{name}{ext}'.format(name=filename, ext=self.extension)
|
||||
|
||||
if filename != self.filename:
|
||||
# Check if it already exists
|
||||
target = self.path(filename=filename)
|
||||
if os.path.isfile(target):
|
||||
raise ErrorException('Cannot rename {source} to {target} as it already exists'.format( # noqa: E501
|
||||
source=self.filename,
|
||||
target=filename
|
||||
))
|
||||
|
||||
os.rename(self.path(), target)
|
||||
|
||||
# Upload a new instructions file
|
||||
def upload(self, file: FileStorage, /) -> None:
|
||||
target = self.path(filename=secure_filename(self.filename))
|
||||
|
||||
if os.path.isfile(target):
|
||||
raise ErrorException('Cannot upload {target} as it already exists'.format( # noqa: E501
|
||||
target=self.filename
|
||||
))
|
||||
|
||||
file.save(target)
|
||||
|
||||
# Info
|
||||
logger.info('The instruction file {file} has been imported'.format(
|
||||
file=self.filename
|
||||
))
|
||||
|
||||
# Compute the url for a set instructions file
|
||||
def url(self, /) -> str:
|
||||
if not self.allowed:
|
||||
return ''
|
||||
|
||||
folder: str = current_app.config['INSTRUCTIONS_FOLDER']
|
||||
|
||||
# Compute the path
|
||||
path = os.path.join(folder, self.filename)
|
||||
|
||||
return url_for('static', filename=path)
|
||||
|
||||
# Return the icon depending on the extension
|
||||
def icon(self, /) -> str:
|
||||
if self.extension == '.pdf':
|
||||
return 'file-pdf-2-line'
|
||||
elif self.extension in ['.doc', '.docx']:
|
||||
return 'file-word-line'
|
||||
elif self.extension in ['.png', '.jpg', '.jpeg']:
|
||||
return 'file-image-line'
|
||||
else:
|
||||
return 'file-line'
|
||||
|
||||
# Find the instructions for a set
|
||||
@staticmethod
|
||||
def find_instructions(set: str, /) -> list[Tuple[str, str]]:
|
||||
response = requests.get(
|
||||
current_app.config['REBRICKABLE_LINK_INSTRUCTIONS_PATTERN'].format(
|
||||
path=set,
|
||||
),
|
||||
headers={
|
||||
'User-Agent': current_app.config['REBRICKABLE_USER_AGENT']
|
||||
}
|
||||
)
|
||||
|
||||
if not response.ok:
|
||||
raise ErrorException('Failed to load the Rebrickable instructions page. Status code: {code}'.format( # noqa: E501
|
||||
code=response.status_code
|
||||
))
|
||||
|
||||
# Parse the HTML content
|
||||
soup = BeautifulSoup(response.content, 'html.parser')
|
||||
|
||||
# Collect all <img> tags with "LEGO Building Instructions" in the
|
||||
# alt attribute
|
||||
found_tags: list[Tuple[str, str]] = []
|
||||
for a_tag in soup.find_all('a', href=True):
|
||||
img_tag = a_tag.find('img', alt=True)
|
||||
if img_tag and "LEGO Building Instructions" in img_tag['alt']:
|
||||
found_tags.append(
|
||||
(
|
||||
img_tag['alt'].removeprefix('LEGO Building Instructions for '), # noqa: E501
|
||||
a_tag['href']
|
||||
)
|
||||
) # Save alt and href
|
||||
|
||||
# Raise an error if nothing found
|
||||
if not len(found_tags):
|
||||
raise ErrorException('No instruction found for set {set}'.format(
|
||||
set=set
|
||||
))
|
||||
|
||||
return found_tags
|
@ -1,106 +0,0 @@
|
||||
import logging
|
||||
import os
|
||||
from typing import Generator, TYPE_CHECKING
|
||||
|
||||
from flask import current_app
|
||||
|
||||
from .exceptions import NotFoundException
|
||||
from .instructions import BrickInstructions
|
||||
from .rebrickable_set_list import RebrickableSetList
|
||||
if TYPE_CHECKING:
|
||||
from .rebrickable_set import RebrickableSet
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
# Lego sets instruction list
|
||||
class BrickInstructionsList(object):
|
||||
all: dict[str, BrickInstructions]
|
||||
rejected_total: int
|
||||
sets: dict[str, list[BrickInstructions]]
|
||||
sets_total: int
|
||||
unknown_total: int
|
||||
|
||||
def __init__(self, /, *, force=False):
|
||||
# Load instructions only if there is none already loaded
|
||||
all = getattr(self, 'all', None)
|
||||
|
||||
if all is None or force:
|
||||
logger.info('Loading instructions file list')
|
||||
|
||||
BrickInstructionsList.all = {}
|
||||
BrickInstructionsList.rejected_total = 0
|
||||
BrickInstructionsList.sets = {}
|
||||
BrickInstructionsList.sets_total = 0
|
||||
BrickInstructionsList.unknown_total = 0
|
||||
|
||||
# Try to list the files in the instruction folder
|
||||
try:
|
||||
# Make a folder relative to static
|
||||
folder: str = os.path.join(
|
||||
current_app.static_folder, # type: ignore
|
||||
current_app.config['INSTRUCTIONS_FOLDER'],
|
||||
)
|
||||
|
||||
for file in os.scandir(folder):
|
||||
instruction = BrickInstructions(file)
|
||||
|
||||
# Unconditionnally add to the list
|
||||
BrickInstructionsList.all[instruction.filename] = instruction # noqa: E501
|
||||
|
||||
if instruction.allowed:
|
||||
if instruction.set:
|
||||
# Instantiate the list if not existing yet
|
||||
if instruction.set not in BrickInstructionsList.sets: # noqa: E501
|
||||
BrickInstructionsList.sets[instruction.set] = [] # noqa: E501
|
||||
|
||||
BrickInstructionsList.sets[instruction.set].append(instruction) # noqa: E501
|
||||
BrickInstructionsList.sets_total += 1
|
||||
else:
|
||||
BrickInstructionsList.unknown_total += 1
|
||||
else:
|
||||
BrickInstructionsList.rejected_total += 1
|
||||
|
||||
# List of Rebrickable sets
|
||||
rebrickable_sets: dict[str, RebrickableSet] = {}
|
||||
for rebrickable_set in RebrickableSetList().all():
|
||||
rebrickable_sets[rebrickable_set.fields.set] = rebrickable_set # noqa: E501
|
||||
|
||||
# Inject the brickset if it exists
|
||||
for instruction in self.all.values():
|
||||
if (
|
||||
instruction.allowed and
|
||||
instruction.set is not None and
|
||||
instruction.rebrickable is None and
|
||||
instruction.set in rebrickable_sets
|
||||
):
|
||||
instruction.rebrickable = rebrickable_sets[instruction.set] # noqa: E501
|
||||
# Ignore errors
|
||||
except Exception:
|
||||
pass
|
||||
|
||||
# Grab instructions for a set
|
||||
def get(self, set: str) -> list[BrickInstructions]:
|
||||
if set in self.sets:
|
||||
return self.sets[set]
|
||||
else:
|
||||
return []
|
||||
|
||||
# Grab instructions for a file
|
||||
def get_file(self, name: str) -> BrickInstructions:
|
||||
if name not in self.all:
|
||||
raise NotFoundException('Instruction file {name} does not exist'.format( # noqa: E501
|
||||
name=name
|
||||
))
|
||||
|
||||
return self.all[name]
|
||||
|
||||
# List of all instruction files
|
||||
def list(self, /) -> Generator[BrickInstructions, None, None]:
|
||||
# Get the filenames and sort them
|
||||
filenames = list(self.all.keys())
|
||||
filenames.sort()
|
||||
|
||||
# Return the files
|
||||
for filename in filenames:
|
||||
yield self.all[filename]
|
@ -1,55 +0,0 @@
|
||||
from flask import current_app, Flask
|
||||
from flask_login import current_user, login_manager, UserMixin
|
||||
|
||||
|
||||
# Login manager wrapper
|
||||
class LoginManager(object):
|
||||
# Login user
|
||||
class User(UserMixin):
|
||||
def __init__(self, name: str, password: str, /):
|
||||
self.id = name
|
||||
self.password = password
|
||||
|
||||
def __init__(self, app: Flask, /):
|
||||
# Setup basic authentication
|
||||
app.secret_key = app.config['AUTHENTICATION_KEY']
|
||||
|
||||
manager = login_manager.LoginManager()
|
||||
manager.login_view = 'login.login' # type: ignore
|
||||
manager.init_app(app)
|
||||
|
||||
# User loader with only one user
|
||||
@manager.user_loader
|
||||
def user_loader(*arg) -> LoginManager.User:
|
||||
return self.User(
|
||||
'admin',
|
||||
app.config['AUTHENTICATION_PASSWORD']
|
||||
)
|
||||
|
||||
# If the password is unset, globally disable
|
||||
app.config['LOGIN_DISABLED'] = app.config['AUTHENTICATION_PASSWORD'] == '' # noqa: E501
|
||||
|
||||
# Tells whether the user is authenticated, meaning:
|
||||
# - Authentication disabled
|
||||
# - or User is authenticated
|
||||
@staticmethod
|
||||
def is_authenticated() -> bool:
|
||||
return (
|
||||
current_app.config['LOGIN_DISABLED'] or
|
||||
current_user.is_authenticated
|
||||
)
|
||||
|
||||
# Tells whether authentication is enabled
|
||||
@staticmethod
|
||||
def is_enabled() -> bool:
|
||||
return not current_app.config['LOGIN_DISABLED']
|
||||
|
||||
# Tells whether we need the user authenticated, meaning:
|
||||
# - Authentication enabled
|
||||
# - and User not authenticated
|
||||
@staticmethod
|
||||
def is_not_authenticated() -> bool:
|
||||
return (
|
||||
not current_app.config['LOGIN_DISABLED'] and
|
||||
not current_user.is_authenticated
|
||||
)
|
@ -1,263 +0,0 @@
|
||||
import logging
|
||||
from sqlite3 import Row
|
||||
from typing import Any, Self, TYPE_CHECKING
|
||||
from uuid import uuid4
|
||||
|
||||
from flask import url_for
|
||||
|
||||
from .exceptions import DatabaseException, ErrorException, NotFoundException
|
||||
from .record import BrickRecord
|
||||
from .sql import BrickSQL
|
||||
if TYPE_CHECKING:
|
||||
from .set import BrickSet
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
# Lego set metadata (customizable list of entries that can be checked)
|
||||
class BrickMetadata(BrickRecord):
|
||||
kind: str
|
||||
|
||||
# Set state endpoint
|
||||
set_state_endpoint: str
|
||||
|
||||
# Queries
|
||||
delete_query: str
|
||||
insert_query: str
|
||||
select_query: str
|
||||
update_field_query: str
|
||||
update_set_state_query: str
|
||||
update_set_value_query: str
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
record: Row | dict[str, Any] | None = None,
|
||||
):
|
||||
super().__init__()
|
||||
|
||||
# Defined an empty ID
|
||||
self.fields.id = None
|
||||
|
||||
# Ingest the record if it has one
|
||||
if record is not None:
|
||||
self.ingest(record)
|
||||
|
||||
# SQL column name
|
||||
def as_column(self, /) -> str:
|
||||
return '{kind}_{id}'.format(
|
||||
id=self.fields.id,
|
||||
kind=self.kind.lower().replace(' ', '-')
|
||||
)
|
||||
|
||||
# HTML dataset name
|
||||
def as_dataset(self, /) -> str:
|
||||
return self.as_column().replace('_', '-')
|
||||
|
||||
# Delete from database
|
||||
def delete(self, /) -> None:
|
||||
BrickSQL().executescript(
|
||||
self.delete_query,
|
||||
id=self.fields.id,
|
||||
)
|
||||
|
||||
# Grab data from a form
|
||||
def from_form(self, form: dict[str, str], /) -> Self:
|
||||
name = form.get('name', None)
|
||||
|
||||
if name is None or name == '':
|
||||
raise ErrorException('Status name cannot be empty')
|
||||
|
||||
self.fields.name = name
|
||||
|
||||
return self
|
||||
|
||||
# Insert into database
|
||||
def insert(self, /, **context) -> None:
|
||||
self.safe()
|
||||
|
||||
# Generate an ID for the metadata (with underscores to make it
|
||||
# column name friendly)
|
||||
self.fields.id = str(uuid4()).replace('-', '_')
|
||||
|
||||
BrickSQL().executescript(
|
||||
self.insert_query,
|
||||
id=self.fields.id,
|
||||
name=self.fields.safe_name,
|
||||
**context
|
||||
)
|
||||
|
||||
# Rename the entry
|
||||
def rename(self, /) -> None:
|
||||
self.update_field('name', value=self.fields.name)
|
||||
|
||||
# Make the name "safe"
|
||||
# Security: eh.
|
||||
def safe(self, /) -> None:
|
||||
# Prevent self-ownage with accidental quote escape
|
||||
self.fields.safe_name = self.fields.name.replace("'", "''")
|
||||
|
||||
# URL to change the selected state of this metadata item for a set
|
||||
def url_for_set_state(self, id: str, /) -> str:
|
||||
return url_for(
|
||||
self.set_state_endpoint,
|
||||
id=id,
|
||||
metadata_id=self.fields.id
|
||||
)
|
||||
|
||||
# Select a specific metadata (with an id)
|
||||
def select_specific(self, id: str, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.id = id
|
||||
|
||||
# Load from database
|
||||
if not self.select():
|
||||
raise NotFoundException(
|
||||
'{kind} with ID {id} was not found in the database'.format(
|
||||
kind=self.kind.capitalize(),
|
||||
id=self.fields.id,
|
||||
),
|
||||
)
|
||||
|
||||
return self
|
||||
|
||||
# Update a field
|
||||
def update_field(
|
||||
self,
|
||||
field: str,
|
||||
/,
|
||||
*,
|
||||
json: Any | None = None,
|
||||
value: Any | None = None
|
||||
) -> Any:
|
||||
if value is None and json is not None:
|
||||
value = json.get('value', None)
|
||||
|
||||
if value is None:
|
||||
raise ErrorException('"{field}" of a {kind} cannot be set to an empty value'.format( # noqa: E501
|
||||
field=field,
|
||||
kind=self.kind
|
||||
))
|
||||
|
||||
if field == 'id' or not hasattr(self.fields, field):
|
||||
raise NotFoundException('"{field}" is not a field of a {kind}'.format( # noqa: E501
|
||||
kind=self.kind,
|
||||
field=field
|
||||
))
|
||||
|
||||
parameters = self.sql_parameters()
|
||||
parameters['value'] = value
|
||||
|
||||
# Update the status
|
||||
rows, _ = BrickSQL().execute_and_commit(
|
||||
self.update_field_query,
|
||||
parameters=parameters,
|
||||
field=field,
|
||||
)
|
||||
|
||||
if rows != 1:
|
||||
raise DatabaseException('Could not update the field "{field}" for {kind} "{name}" ({id})'.format( # noqa: E501
|
||||
field=field,
|
||||
kind=self.kind,
|
||||
name=self.fields.name,
|
||||
id=self.fields.id,
|
||||
))
|
||||
|
||||
# Info
|
||||
logger.info('{kind} "{name}" ({id}): field "{field}" changed to "{value}"'.format( # noqa: E501
|
||||
kind=self.kind.capitalize(),
|
||||
name=self.fields.name,
|
||||
id=self.fields.id,
|
||||
field=field,
|
||||
value=value,
|
||||
))
|
||||
|
||||
return value
|
||||
|
||||
# Update the selected state of this metadata item for a set
|
||||
def update_set_state(
|
||||
self,
|
||||
brickset: 'BrickSet',
|
||||
/,
|
||||
*,
|
||||
json: Any | None = None,
|
||||
state: Any | None = None
|
||||
) -> Any:
|
||||
if state is None and json is not None:
|
||||
state = json.get('value', False)
|
||||
|
||||
parameters = self.sql_parameters()
|
||||
parameters['set_id'] = brickset.fields.id
|
||||
parameters['state'] = state
|
||||
|
||||
rows, _ = BrickSQL().execute_and_commit(
|
||||
self.update_set_state_query,
|
||||
parameters=parameters,
|
||||
name=self.as_column(),
|
||||
)
|
||||
|
||||
if rows != 1:
|
||||
raise DatabaseException('Could not update the {kind} "{name}" state for set {set} ({id})'.format( # noqa: E501
|
||||
kind=self.kind,
|
||||
name=self.fields.name,
|
||||
set=brickset.fields.set,
|
||||
id=brickset.fields.id,
|
||||
))
|
||||
|
||||
# Info
|
||||
logger.info('{kind} "{name}" state changed to "{state}" for set {set} ({id})'.format( # noqa: E501
|
||||
kind=self.kind,
|
||||
name=self.fields.name,
|
||||
state=state,
|
||||
set=brickset.fields.set,
|
||||
id=brickset.fields.id,
|
||||
))
|
||||
|
||||
return state
|
||||
|
||||
# Update the selected value of this metadata item for a set
|
||||
def update_set_value(
|
||||
self,
|
||||
brickset: 'BrickSet',
|
||||
/,
|
||||
*,
|
||||
json: Any | None = None,
|
||||
value: Any | None = None,
|
||||
) -> Any:
|
||||
if value is None and json is not None:
|
||||
value = json.get('value', '')
|
||||
|
||||
if value == '':
|
||||
value = None
|
||||
|
||||
parameters = self.sql_parameters()
|
||||
parameters['set_id'] = brickset.fields.id
|
||||
parameters['value'] = value
|
||||
|
||||
rows, _ = BrickSQL().execute_and_commit(
|
||||
self.update_set_value_query,
|
||||
parameters=parameters,
|
||||
)
|
||||
|
||||
# Update the status
|
||||
if value is None and not hasattr(self.fields, 'name'):
|
||||
self.fields.name = 'None'
|
||||
|
||||
if rows != 1:
|
||||
raise DatabaseException('Could not update the {kind} value for set {set} ({id})'.format( # noqa: E501
|
||||
kind=self.kind,
|
||||
set=brickset.fields.set,
|
||||
id=brickset.fields.id,
|
||||
))
|
||||
|
||||
# Info
|
||||
logger.info('{kind} value changed to "{name}" ({value}) for set {set} ({id})'.format( # noqa: E501
|
||||
kind=self.kind,
|
||||
name=self.fields.name,
|
||||
value=value,
|
||||
set=brickset.fields.set,
|
||||
id=brickset.fields.id,
|
||||
))
|
||||
|
||||
return value
|
@ -1,176 +0,0 @@
|
||||
import logging
|
||||
from typing import List, overload, Self, Type, TypeVar
|
||||
|
||||
from flask import url_for
|
||||
|
||||
from .exceptions import ErrorException, NotFoundException
|
||||
from .fields import BrickRecordFields
|
||||
from .record_list import BrickRecordList
|
||||
from .set_owner import BrickSetOwner
|
||||
from .set_purchase_location import BrickSetPurchaseLocation
|
||||
from .set_status import BrickSetStatus
|
||||
from .set_storage import BrickSetStorage
|
||||
from .set_tag import BrickSetTag
|
||||
from .wish_owner import BrickWishOwner
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
T = TypeVar(
|
||||
'T',
|
||||
BrickSetOwner,
|
||||
BrickSetPurchaseLocation,
|
||||
BrickSetStatus,
|
||||
BrickSetStorage,
|
||||
BrickSetTag,
|
||||
BrickWishOwner
|
||||
)
|
||||
|
||||
|
||||
# Lego sets metadata list
|
||||
class BrickMetadataList(BrickRecordList[T]):
|
||||
kind: str
|
||||
mapping: dict[str, T]
|
||||
model: Type[T]
|
||||
|
||||
# Database
|
||||
table: str
|
||||
order: str
|
||||
|
||||
# Queries
|
||||
select_query: str
|
||||
|
||||
# Set endpoints
|
||||
set_state_endpoint: str
|
||||
set_value_endpoint: str
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
model: Type[T],
|
||||
/,
|
||||
*,
|
||||
force: bool = False,
|
||||
records: list[T] | None = None
|
||||
):
|
||||
self.model = model
|
||||
|
||||
# Records override (masking the class variables with instance ones)
|
||||
if records is not None:
|
||||
self.override()
|
||||
|
||||
for metadata in records:
|
||||
self.records.append(metadata)
|
||||
self.mapping[metadata.fields.id] = metadata
|
||||
else:
|
||||
# Load metadata only if there is none already loaded
|
||||
records = getattr(self, 'records', None)
|
||||
|
||||
if records is None or force:
|
||||
# Don't use super()__init__ as it would mask class variables
|
||||
self.fields = BrickRecordFields()
|
||||
|
||||
logger.info('Loading {kind} list'.format(
|
||||
kind=self.kind
|
||||
))
|
||||
|
||||
self.__class__.records = []
|
||||
self.__class__.mapping = {}
|
||||
|
||||
# Load the metadata from the database
|
||||
for record in self.select(order=self.order):
|
||||
metadata = model(record=record)
|
||||
|
||||
self.__class__.records.append(metadata)
|
||||
self.__class__.mapping[metadata.fields.id] = metadata
|
||||
|
||||
# HTML prefix name
|
||||
def as_prefix(self, /) -> str:
|
||||
return self.kind.replace(' ', '-')
|
||||
|
||||
# Filter the list of records (this one does nothing)
|
||||
def filter(self) -> list[T]:
|
||||
return self.records
|
||||
|
||||
# Add a layer of override data
|
||||
def override(self) -> None:
|
||||
self.fields = BrickRecordFields()
|
||||
|
||||
self.records = []
|
||||
self.mapping = {}
|
||||
|
||||
# Return the items as columns for a select
|
||||
@classmethod
|
||||
def as_columns(cls, /, **kwargs) -> str:
|
||||
new = cls.new()
|
||||
|
||||
return ', '.join([
|
||||
'"{table}"."{column}"'.format(
|
||||
table=cls.table,
|
||||
column=record.as_column(),
|
||||
)
|
||||
for record
|
||||
in new.filter(**kwargs)
|
||||
])
|
||||
|
||||
# Grab a specific status
|
||||
@classmethod
|
||||
def get(cls, id: str | None, /, *, allow_none: bool = False) -> T:
|
||||
new = cls.new()
|
||||
|
||||
if allow_none and (id == '' or id is None):
|
||||
return new.model()
|
||||
|
||||
if id is None:
|
||||
raise ErrorException('Cannot get {kind} with no ID'.format(
|
||||
kind=new.kind.capitalize()
|
||||
))
|
||||
|
||||
if id not in new.mapping:
|
||||
raise NotFoundException(
|
||||
'{kind} with ID {id} was not found in the database'.format(
|
||||
kind=new.kind.capitalize(),
|
||||
id=id,
|
||||
),
|
||||
)
|
||||
|
||||
return new.mapping[id]
|
||||
|
||||
# Get the list of statuses depending on the context
|
||||
@overload
|
||||
@classmethod
|
||||
def list(cls, /, **kwargs) -> List[T]: ...
|
||||
|
||||
@overload
|
||||
@classmethod
|
||||
def list(cls, /, as_class: bool = False, **kwargs) -> Self: ...
|
||||
|
||||
@classmethod
|
||||
def list(cls, /, as_class: bool = False, **kwargs) -> List[T] | Self:
|
||||
new = cls.new()
|
||||
list = new.filter(**kwargs)
|
||||
|
||||
if as_class:
|
||||
# Return a copy of the metadata list with overriden records
|
||||
return cls(new.model, records=list)
|
||||
else:
|
||||
return list
|
||||
|
||||
# Instantiate the list with the proper class
|
||||
@classmethod
|
||||
def new(cls, /, *, force: bool = False) -> Self:
|
||||
raise Exception('new() is not implemented for BrickMetadataList')
|
||||
|
||||
# URL to change the selected state of this metadata item for a set
|
||||
@classmethod
|
||||
def url_for_set_state(cls, id: str, /) -> str:
|
||||
return url_for(
|
||||
cls.set_state_endpoint,
|
||||
id=id,
|
||||
)
|
||||
|
||||
# URL to change the selected value of this metadata item for a set
|
||||
@classmethod
|
||||
def url_for_set_value(cls, id: str, /) -> str:
|
||||
return url_for(
|
||||
cls.set_value_endpoint,
|
||||
id=id,
|
||||
)
|
@ -1,29 +0,0 @@
|
||||
from typing import Any, TYPE_CHECKING
|
||||
|
||||
if TYPE_CHECKING:
|
||||
from ..sql import BrickSQL
|
||||
|
||||
|
||||
# Grab the list of checkboxes to create a list of SQL columns
|
||||
def migration_0007(sql: 'BrickSQL', /) -> dict[str, Any]:
|
||||
# Don't realy on sql files as they could be removed in the future
|
||||
sql.cursor.execute('SELECT "bricktracker_set_checkboxes"."id" FROM "bricktracker_set_checkboxes"') # noqa: E501
|
||||
records = sql.cursor.fetchall()
|
||||
|
||||
return {
|
||||
'sources': ', '.join([
|
||||
'"bricktracker_set_statuses_old"."status_{id}"'.format(id=record['id']) # noqa: E501
|
||||
for record
|
||||
in records
|
||||
]),
|
||||
'targets': ', '.join([
|
||||
'"status_{id}"'.format(id=record['id'])
|
||||
for record
|
||||
in records
|
||||
]),
|
||||
'structure': ', '.join([
|
||||
'"status_{id}" BOOLEAN NOT NULL DEFAULT 0'.format(id=record['id'])
|
||||
for record
|
||||
in records
|
||||
])
|
||||
}
|
@ -1,108 +0,0 @@
|
||||
import logging
|
||||
import traceback
|
||||
from typing import Self, TYPE_CHECKING
|
||||
|
||||
from .exceptions import ErrorException, NotFoundException
|
||||
from .part_list import BrickPartList
|
||||
from .rebrickable_minifigure import RebrickableMinifigure
|
||||
if TYPE_CHECKING:
|
||||
from .set import BrickSet
|
||||
from .socket import BrickSocket
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
# Lego minifigure
|
||||
class BrickMinifigure(RebrickableMinifigure):
|
||||
# Queries
|
||||
insert_query: str = 'minifigure/insert'
|
||||
generic_query: str = 'minifigure/select/generic'
|
||||
select_query: str = 'minifigure/select/specific'
|
||||
|
||||
# Import a minifigure into the database
|
||||
def download(self, socket: 'BrickSocket', refresh: bool = False) -> bool:
|
||||
if self.brickset is None:
|
||||
raise ErrorException('Importing a minifigure from Rebrickable outside of a set is not supported') # noqa: E501
|
||||
|
||||
try:
|
||||
# Insert into the database
|
||||
socket.auto_progress(
|
||||
message='Set {set}: inserting minifigure {figure} into database'.format( # noqa: E501
|
||||
set=self.brickset.fields.set,
|
||||
figure=self.fields.figure
|
||||
)
|
||||
)
|
||||
|
||||
if not refresh:
|
||||
# Insert into database
|
||||
self.insert(commit=False)
|
||||
|
||||
# Load the inventory
|
||||
if not BrickPartList.download(
|
||||
socket,
|
||||
self.brickset,
|
||||
minifigure=self,
|
||||
refresh=refresh
|
||||
):
|
||||
return False
|
||||
|
||||
# Insert the rebrickable set into database (after counting parts)
|
||||
self.insert_rebrickable()
|
||||
|
||||
except Exception as e:
|
||||
socket.fail(
|
||||
message='Error while importing minifigure {figure} from {set}: {error}'.format( # noqa: E501
|
||||
figure=self.fields.figure,
|
||||
set=self.brickset.fields.set,
|
||||
error=e,
|
||||
)
|
||||
)
|
||||
|
||||
logger.debug(traceback.format_exc())
|
||||
|
||||
return False
|
||||
|
||||
return True
|
||||
|
||||
# Parts
|
||||
def generic_parts(self, /) -> BrickPartList:
|
||||
return BrickPartList().from_minifigure(self)
|
||||
|
||||
# Parts
|
||||
def parts(self, /) -> BrickPartList:
|
||||
if self.brickset is None:
|
||||
raise ErrorException('Part list for minifigure {figure} requires a brickset'.format( # noqa: E501
|
||||
figure=self.fields.figure,
|
||||
))
|
||||
|
||||
return BrickPartList().list_specific(self.brickset, minifigure=self)
|
||||
|
||||
# Select a generic minifigure
|
||||
def select_generic(self, figure: str, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.figure = figure
|
||||
|
||||
if not self.select(override_query=self.generic_query):
|
||||
raise NotFoundException(
|
||||
'Minifigure with figure {figure} was not found in the database'.format( # noqa: E501
|
||||
figure=self.fields.figure,
|
||||
),
|
||||
)
|
||||
|
||||
return self
|
||||
|
||||
# Select a specific minifigure (with a set and a figure)
|
||||
def select_specific(self, brickset: 'BrickSet', figure: str, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.brickset = brickset
|
||||
self.fields.figure = figure
|
||||
|
||||
if not self.select():
|
||||
raise NotFoundException(
|
||||
'Minifigure with figure {figure} from set {set} was not found in the database'.format( # noqa: E501
|
||||
figure=self.fields.figure,
|
||||
set=self.brickset.fields.set,
|
||||
),
|
||||
)
|
||||
|
||||
return self
|
@ -1,183 +0,0 @@
|
||||
import logging
|
||||
import traceback
|
||||
from typing import Any, Self, TYPE_CHECKING
|
||||
|
||||
from flask import current_app
|
||||
|
||||
from .minifigure import BrickMinifigure
|
||||
from .rebrickable import Rebrickable
|
||||
from .record_list import BrickRecordList
|
||||
if TYPE_CHECKING:
|
||||
from .set import BrickSet
|
||||
from .socket import BrickSocket
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
# Lego minifigures
|
||||
class BrickMinifigureList(BrickRecordList[BrickMinifigure]):
|
||||
brickset: 'BrickSet | None'
|
||||
order: str
|
||||
|
||||
# Queries
|
||||
all_query: str = 'minifigure/list/all'
|
||||
damaged_part_query: str = 'minifigure/list/damaged_part'
|
||||
last_query: str = 'minifigure/list/last'
|
||||
missing_part_query: str = 'minifigure/list/missing_part'
|
||||
select_query: str = 'minifigure/list/from_set'
|
||||
using_part_query: str = 'minifigure/list/using_part'
|
||||
|
||||
def __init__(self, /):
|
||||
super().__init__()
|
||||
|
||||
# Placeholders
|
||||
self.brickset = None
|
||||
|
||||
# Store the order for this list
|
||||
self.order = current_app.config['MINIFIGURES_DEFAULT_ORDER']
|
||||
|
||||
# Load all minifigures
|
||||
def all(self, /) -> Self:
|
||||
self.list(override_query=self.all_query)
|
||||
|
||||
return self
|
||||
|
||||
# Minifigures with a part damaged part
|
||||
def damaged_part(self, part: str, color: int, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.part = part
|
||||
self.fields.color = color
|
||||
|
||||
# Load the minifigures from the database
|
||||
self.list(override_query=self.damaged_part_query)
|
||||
|
||||
return self
|
||||
|
||||
# Last added minifigure
|
||||
def last(self, /, *, limit: int = 6) -> Self:
|
||||
# Randomize
|
||||
if current_app.config['RANDOM']:
|
||||
order = 'RANDOM()'
|
||||
else:
|
||||
order = '"bricktracker_minifigures"."rowid" DESC'
|
||||
|
||||
self.list(override_query=self.last_query, order=order, limit=limit)
|
||||
|
||||
return self
|
||||
|
||||
# Base minifigure list
|
||||
def list(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
override_query: str | None = None,
|
||||
order: str | None = None,
|
||||
limit: int | None = None,
|
||||
**context: Any,
|
||||
) -> None:
|
||||
if order is None:
|
||||
order = self.order
|
||||
|
||||
if hasattr(self, 'brickset'):
|
||||
brickset = self.brickset
|
||||
else:
|
||||
brickset = None
|
||||
|
||||
# Load the sets from the database
|
||||
for record in super().select(
|
||||
override_query=override_query,
|
||||
order=order,
|
||||
limit=limit,
|
||||
):
|
||||
minifigure = BrickMinifigure(brickset=brickset, record=record)
|
||||
|
||||
self.records.append(minifigure)
|
||||
|
||||
# Load minifigures from a brickset
|
||||
def from_set(self, brickset: 'BrickSet', /) -> Self:
|
||||
# Save the brickset
|
||||
self.brickset = brickset
|
||||
|
||||
# Load the minifigures from the database
|
||||
self.list()
|
||||
|
||||
return self
|
||||
|
||||
# Minifigures missing a part
|
||||
def missing_part(self, part: str, color: int, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.part = part
|
||||
self.fields.color = color
|
||||
|
||||
# Load the minifigures from the database
|
||||
self.list(override_query=self.missing_part_query)
|
||||
|
||||
return self
|
||||
|
||||
# Minifigure using a part
|
||||
def using_part(self, part: str, color: int, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.part = part
|
||||
self.fields.color = color
|
||||
|
||||
# Load the minifigures from the database
|
||||
self.list(override_query=self.using_part_query)
|
||||
|
||||
return self
|
||||
|
||||
# Return a dict with common SQL parameters for a minifigures list
|
||||
def sql_parameters(self, /) -> dict[str, Any]:
|
||||
parameters: dict[str, Any] = super().sql_parameters()
|
||||
|
||||
if self.brickset is not None:
|
||||
parameters['id'] = self.brickset.fields.id
|
||||
|
||||
return parameters
|
||||
|
||||
# Import the minifigures from Rebrickable
|
||||
@staticmethod
|
||||
def download(
|
||||
socket: 'BrickSocket',
|
||||
brickset: 'BrickSet',
|
||||
/,
|
||||
*,
|
||||
refresh: bool = False
|
||||
) -> bool:
|
||||
try:
|
||||
socket.auto_progress(
|
||||
message='Set {set}: loading minifigures from Rebrickable'.format( # noqa: E501
|
||||
set=brickset.fields.set,
|
||||
),
|
||||
increment_total=True,
|
||||
)
|
||||
|
||||
logger.debug('rebrick.lego.get_set_minifigs("{set}")'.format(
|
||||
set=brickset.fields.set,
|
||||
))
|
||||
|
||||
minifigures = Rebrickable[BrickMinifigure](
|
||||
'get_set_minifigs',
|
||||
brickset.fields.set,
|
||||
BrickMinifigure,
|
||||
socket=socket,
|
||||
brickset=brickset,
|
||||
).list()
|
||||
|
||||
# Process each minifigure
|
||||
for minifigure in minifigures:
|
||||
if not minifigure.download(socket, refresh=refresh):
|
||||
return False
|
||||
|
||||
return True
|
||||
|
||||
except Exception as e:
|
||||
socket.fail(
|
||||
message='Error while importing set {set} minifigure list: {error}'.format( # noqa: E501
|
||||
set=brickset.fields.set,
|
||||
error=e,
|
||||
)
|
||||
)
|
||||
|
||||
logger.debug(traceback.format_exc())
|
||||
|
||||
return False
|
@ -1,52 +0,0 @@
|
||||
from typing import Any, Final
|
||||
|
||||
from flask import Flask
|
||||
|
||||
# Navbar map:
|
||||
# - e: url endpoint (str)
|
||||
# - t: title (str)
|
||||
# - i: icon (str, optional=None)
|
||||
# - f: flag name (str, optional=None)
|
||||
NAVBAR: Final[list[dict[str, Any]]] = [
|
||||
{'e': 'set.list', 't': 'Sets', 'i': 'grid-line', 'f': 'HIDE_ALL_SETS'}, # noqa: E501
|
||||
{'e': 'add.add', 't': 'Add', 'i': 'add-circle-line', 'f': 'HIDE_ADD_SET'}, # noqa: E501
|
||||
{'e': 'part.list', 't': 'Parts', 'i': 'shapes-line', 'f': 'HIDE_ALL_PARTS'}, # noqa: E501
|
||||
{'e': 'part.problem', 't': 'Problems', 'i': 'error-warning-line', 'f': 'HIDE_ALL_PROBLEMS_PARTS'}, # noqa: E501
|
||||
{'e': 'minifigure.list', 't': 'Minifigures', 'i': 'group-line', 'f': 'HIDE_ALL_MINIFIGURES'}, # noqa: E501
|
||||
{'e': 'instructions.list', 't': 'Instructions', 'i': 'file-line', 'f': 'HIDE_ALL_INSTRUCTIONS'}, # noqa: E501
|
||||
{'e': 'storage.list', 't': 'Storages', 'i': 'archive-2-line', 'f': 'HIDE_ALL_STORAGES'}, # noqa: E501
|
||||
{'e': 'wish.list', 't': 'Wishlist', 'i': 'gift-line', 'f': 'HIDE_WISHES'},
|
||||
{'e': 'admin.admin', 't': 'Admin', 'i': 'settings-4-line', 'f': 'HIDE_ADMIN'}, # noqa: E501
|
||||
]
|
||||
|
||||
|
||||
# Navbar configuration
|
||||
class Navbar(object):
|
||||
# Navbar item
|
||||
class NavbarItem(object):
|
||||
endpoint: str
|
||||
title: str
|
||||
icon: str | None
|
||||
flag: str | None
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
*,
|
||||
e: str,
|
||||
t: str,
|
||||
i: str | None = None,
|
||||
f: str | None = None,
|
||||
):
|
||||
self.endpoint = e
|
||||
self.title = t
|
||||
self.icon = i
|
||||
self.flag = f
|
||||
|
||||
# Load configuration
|
||||
def __init__(self, app: Flask, /):
|
||||
# Navbar storage
|
||||
app.config['_NAVBAR'] = []
|
||||
|
||||
# Process all configuration items
|
||||
for item in NAVBAR:
|
||||
app.config['_NAVBAR'].append(self.NavbarItem(**item))
|
@ -1,37 +0,0 @@
|
||||
from .exceptions import ErrorException
|
||||
|
||||
|
||||
# Make sense of string supposed to contain a set ID
|
||||
def parse_set(set: str, /) -> str:
|
||||
number, _, version = set.partition('-')
|
||||
|
||||
# Making sure both are integers
|
||||
if version == '':
|
||||
version = 1
|
||||
|
||||
try:
|
||||
number = int(number)
|
||||
except Exception:
|
||||
raise ErrorException('Number "{number}" is not a number'.format(
|
||||
number=number,
|
||||
))
|
||||
|
||||
try:
|
||||
version = int(version)
|
||||
except Exception:
|
||||
raise ErrorException('Version "{version}" is not a number'.format(
|
||||
version=version,
|
||||
))
|
||||
|
||||
# Make sure both are positive
|
||||
if number < 0:
|
||||
raise ErrorException('Number "{number}" should be positive'.format(
|
||||
number=number,
|
||||
))
|
||||
|
||||
if version < 0:
|
||||
raise ErrorException('Version "{version}" should be positive'.format( # noqa: E501
|
||||
version=version,
|
||||
))
|
||||
|
||||
return '{number}-{version}'.format(number=number, version=version)
|
@ -1,208 +0,0 @@
|
||||
import logging
|
||||
from sqlite3 import Row
|
||||
from typing import Any, Self, TYPE_CHECKING
|
||||
import traceback
|
||||
|
||||
from flask import url_for
|
||||
|
||||
from .exceptions import ErrorException, NotFoundException
|
||||
from .rebrickable_part import RebrickablePart
|
||||
from .sql import BrickSQL
|
||||
if TYPE_CHECKING:
|
||||
from .minifigure import BrickMinifigure
|
||||
from .set import BrickSet
|
||||
from .socket import BrickSocket
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
# Lego set or minifig part
|
||||
class BrickPart(RebrickablePart):
|
||||
identifier: str
|
||||
kind: str
|
||||
|
||||
# Queries
|
||||
insert_query: str = 'part/insert'
|
||||
generic_query: str = 'part/select/generic'
|
||||
select_query: str = 'part/select/specific'
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
brickset: 'BrickSet | None' = None,
|
||||
minifigure: 'BrickMinifigure | None' = None,
|
||||
record: Row | dict[str, Any] | None = None
|
||||
):
|
||||
super().__init__(
|
||||
brickset=brickset,
|
||||
minifigure=minifigure,
|
||||
record=record
|
||||
)
|
||||
|
||||
if self.minifigure is not None:
|
||||
self.identifier = self.minifigure.fields.figure
|
||||
self.kind = 'Minifigure'
|
||||
elif self.brickset is not None:
|
||||
self.identifier = self.brickset.fields.set
|
||||
self.kind = 'Set'
|
||||
|
||||
# Import a part into the database
|
||||
def download(self, socket: 'BrickSocket', refresh: bool = False) -> bool:
|
||||
if self.brickset is None:
|
||||
raise ErrorException('Importing a part from Rebrickable outside of a set is not supported') # noqa: E501
|
||||
|
||||
try:
|
||||
# Insert into the database
|
||||
socket.auto_progress(
|
||||
message='{kind} {identifier}: inserting part {part} into database'.format( # noqa: E501
|
||||
kind=self.kind,
|
||||
identifier=self.identifier,
|
||||
part=self.fields.part
|
||||
)
|
||||
)
|
||||
|
||||
if not refresh:
|
||||
# Insert into database
|
||||
self.insert(commit=False)
|
||||
|
||||
# Insert the rebrickable set into database
|
||||
self.insert_rebrickable()
|
||||
|
||||
except Exception as e:
|
||||
socket.fail(
|
||||
message='Error while importing part {part} from {kind} {identifier}: {error}'.format( # noqa: E501
|
||||
part=self.fields.part,
|
||||
kind=self.kind,
|
||||
identifier=self.identifier,
|
||||
error=e,
|
||||
)
|
||||
)
|
||||
|
||||
logger.debug(traceback.format_exc())
|
||||
|
||||
return False
|
||||
|
||||
return True
|
||||
|
||||
# A identifier for HTML component
|
||||
def html_id(self, prefix: str | None = None, /) -> str:
|
||||
components: list[str] = ['part']
|
||||
|
||||
if prefix is not None:
|
||||
components.append(prefix)
|
||||
|
||||
if self.fields.figure is not None:
|
||||
components.append(self.fields.figure)
|
||||
|
||||
components.append(self.fields.part)
|
||||
components.append(str(self.fields.color))
|
||||
components.append(str(self.fields.spare))
|
||||
|
||||
return '-'.join(components)
|
||||
|
||||
# Select a generic part
|
||||
def select_generic(
|
||||
self,
|
||||
part: str,
|
||||
color: int,
|
||||
/,
|
||||
) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.part = part
|
||||
self.fields.color = color
|
||||
|
||||
if not self.select(override_query=self.generic_query):
|
||||
raise NotFoundException(
|
||||
'Part with number {number}, color ID {color} was not found in the database'.format( # noqa: E501
|
||||
number=self.fields.part,
|
||||
color=self.fields.color,
|
||||
),
|
||||
)
|
||||
|
||||
return self
|
||||
|
||||
# Select a specific part (with a set and an id, and option. a minifigure)
|
||||
def select_specific(
|
||||
self,
|
||||
brickset: 'BrickSet',
|
||||
part: str,
|
||||
color: int,
|
||||
spare: int,
|
||||
/,
|
||||
*,
|
||||
minifigure: 'BrickMinifigure | None' = None,
|
||||
) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.brickset = brickset
|
||||
self.minifigure = minifigure
|
||||
self.fields.part = part
|
||||
self.fields.color = color
|
||||
self.fields.spare = spare
|
||||
|
||||
if not self.select():
|
||||
if self.minifigure is not None:
|
||||
figure = self.minifigure.fields.figure
|
||||
else:
|
||||
figure = None
|
||||
|
||||
raise NotFoundException(
|
||||
'Part {part} with color {color} (spare: {spare}) from set {set} ({id}) (minifigure: {figure}) was not found in the database'.format( # noqa: E501
|
||||
part=self.fields.part,
|
||||
color=self.fields.color,
|
||||
spare=self.fields.spare,
|
||||
id=self.fields.id,
|
||||
set=self.brickset.fields.set,
|
||||
figure=figure,
|
||||
),
|
||||
)
|
||||
|
||||
return self
|
||||
|
||||
# Update a problematic part
|
||||
def update_problem(self, problem: str, json: Any | None, /) -> int:
|
||||
amount: str | int = json.get('value', '') # type: ignore
|
||||
|
||||
# We need a positive integer
|
||||
try:
|
||||
if amount == '':
|
||||
amount = 0
|
||||
|
||||
amount = int(amount)
|
||||
|
||||
if amount < 0:
|
||||
amount = 0
|
||||
except Exception:
|
||||
raise ErrorException('"{amount}" is not a valid integer'.format(
|
||||
amount=amount
|
||||
))
|
||||
|
||||
if amount < 0:
|
||||
raise ErrorException('Cannot set a negative amount')
|
||||
|
||||
setattr(self.fields, problem, amount)
|
||||
|
||||
BrickSQL().execute_and_commit(
|
||||
'part/update/{problem}'.format(problem=problem),
|
||||
parameters=self.sql_parameters()
|
||||
)
|
||||
|
||||
return amount
|
||||
|
||||
# Compute the url for problematic part
|
||||
def url_for_problem(self, problem: str, /) -> str:
|
||||
# Different URL for a minifigure part
|
||||
if self.minifigure is not None:
|
||||
figure = self.minifigure.fields.figure
|
||||
else:
|
||||
figure = None
|
||||
|
||||
return url_for(
|
||||
'set.problem_part',
|
||||
id=self.fields.id,
|
||||
figure=figure,
|
||||
part=self.fields.part,
|
||||
color=self.fields.color,
|
||||
spare=self.fields.spare,
|
||||
problem=problem,
|
||||
)
|
@ -1,245 +0,0 @@
|
||||
import logging
|
||||
from typing import Any, Self, TYPE_CHECKING
|
||||
import traceback
|
||||
|
||||
from flask import current_app
|
||||
|
||||
from .part import BrickPart
|
||||
from .rebrickable import Rebrickable
|
||||
from .record_list import BrickRecordList
|
||||
if TYPE_CHECKING:
|
||||
from .minifigure import BrickMinifigure
|
||||
from .set import BrickSet
|
||||
from .socket import BrickSocket
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
# Lego set or minifig parts
|
||||
class BrickPartList(BrickRecordList[BrickPart]):
|
||||
brickset: 'BrickSet | None'
|
||||
minifigure: 'BrickMinifigure | None'
|
||||
order: str
|
||||
|
||||
# Queries
|
||||
all_query: str = 'part/list/all'
|
||||
different_color_query = 'part/list/with_different_color'
|
||||
last_query: str = 'part/list/last'
|
||||
minifigure_query: str = 'part/list/from_minifigure'
|
||||
problem_query: str = 'part/list/problem'
|
||||
print_query: str = 'part/list/from_print'
|
||||
select_query: str = 'part/list/specific'
|
||||
|
||||
def __init__(self, /):
|
||||
super().__init__()
|
||||
|
||||
# Placeholders
|
||||
self.brickset = None
|
||||
self.minifigure = None
|
||||
|
||||
# Store the order for this list
|
||||
self.order = current_app.config['PARTS_DEFAULT_ORDER']
|
||||
|
||||
# Load all parts
|
||||
def all(self, /) -> Self:
|
||||
self.list(override_query=self.all_query)
|
||||
|
||||
return self
|
||||
|
||||
# Base part list
|
||||
def list(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
override_query: str | None = None,
|
||||
order: str | None = None,
|
||||
limit: int | None = None,
|
||||
**context: Any,
|
||||
) -> None:
|
||||
if order is None:
|
||||
order = self.order
|
||||
|
||||
if hasattr(self, 'brickset'):
|
||||
brickset = self.brickset
|
||||
else:
|
||||
brickset = None
|
||||
|
||||
if hasattr(self, 'minifigure'):
|
||||
minifigure = self.minifigure
|
||||
else:
|
||||
minifigure = None
|
||||
|
||||
# Load the sets from the database
|
||||
for record in super().select(
|
||||
override_query=override_query,
|
||||
order=order,
|
||||
limit=limit,
|
||||
):
|
||||
part = BrickPart(
|
||||
brickset=brickset,
|
||||
minifigure=minifigure,
|
||||
record=record,
|
||||
)
|
||||
|
||||
if current_app.config['SKIP_SPARE_PARTS'] and part.fields.spare:
|
||||
continue
|
||||
|
||||
self.records.append(part)
|
||||
|
||||
# List specific parts from a brickset or minifigure
|
||||
def list_specific(
|
||||
self,
|
||||
brickset: 'BrickSet',
|
||||
/,
|
||||
*,
|
||||
minifigure: 'BrickMinifigure | None' = None,
|
||||
) -> Self:
|
||||
# Save the brickset and minifigure
|
||||
self.brickset = brickset
|
||||
self.minifigure = minifigure
|
||||
|
||||
# Load the parts from the database
|
||||
self.list()
|
||||
|
||||
return self
|
||||
|
||||
# Load generic parts from a minifigure
|
||||
def from_minifigure(
|
||||
self,
|
||||
minifigure: 'BrickMinifigure',
|
||||
/,
|
||||
) -> Self:
|
||||
# Save the minifigure
|
||||
self.minifigure = minifigure
|
||||
|
||||
# Load the parts from the database
|
||||
self.list(override_query=self.minifigure_query)
|
||||
|
||||
return self
|
||||
|
||||
# Load generic parts from a print
|
||||
def from_print(
|
||||
self,
|
||||
brickpart: BrickPart,
|
||||
/,
|
||||
) -> Self:
|
||||
# Save the part and print
|
||||
if brickpart.fields.print is not None:
|
||||
self.fields.print = brickpart.fields.print
|
||||
else:
|
||||
self.fields.print = brickpart.fields.part
|
||||
|
||||
self.fields.part = brickpart.fields.part
|
||||
self.fields.color = brickpart.fields.color
|
||||
|
||||
# Load the parts from the database
|
||||
self.list(override_query=self.print_query)
|
||||
|
||||
return self
|
||||
|
||||
# Load problematic parts
|
||||
def problem(self, /) -> Self:
|
||||
self.list(override_query=self.problem_query)
|
||||
|
||||
return self
|
||||
|
||||
# Return a dict with common SQL parameters for a parts list
|
||||
def sql_parameters(self, /) -> dict[str, Any]:
|
||||
parameters: dict[str, Any] = super().sql_parameters()
|
||||
|
||||
# Set id
|
||||
if self.brickset is not None:
|
||||
parameters['id'] = self.brickset.fields.id
|
||||
|
||||
# Use the minifigure number if present,
|
||||
if self.minifigure is not None:
|
||||
parameters['figure'] = self.minifigure.fields.figure
|
||||
else:
|
||||
parameters['figure'] = None
|
||||
|
||||
return parameters
|
||||
|
||||
# Load generic parts with same base but different color
|
||||
def with_different_color(
|
||||
self,
|
||||
brickpart: BrickPart,
|
||||
/,
|
||||
) -> Self:
|
||||
# Save the part
|
||||
self.fields.part = brickpart.fields.part
|
||||
self.fields.color = brickpart.fields.color
|
||||
|
||||
# Load the parts from the database
|
||||
self.list(override_query=self.different_color_query)
|
||||
|
||||
return self
|
||||
|
||||
# Import the parts from Rebrickable
|
||||
@staticmethod
|
||||
def download(
|
||||
socket: 'BrickSocket',
|
||||
brickset: 'BrickSet',
|
||||
/,
|
||||
*,
|
||||
minifigure: 'BrickMinifigure | None' = None,
|
||||
refresh: bool = False
|
||||
) -> bool:
|
||||
if minifigure is not None:
|
||||
identifier = minifigure.fields.figure
|
||||
kind = 'Minifigure'
|
||||
method = 'get_minifig_elements'
|
||||
else:
|
||||
identifier = brickset.fields.set
|
||||
kind = 'Set'
|
||||
method = 'get_set_elements'
|
||||
|
||||
try:
|
||||
socket.auto_progress(
|
||||
message='{kind} {identifier}: loading parts inventory from Rebrickable'.format( # noqa: E501
|
||||
kind=kind,
|
||||
identifier=identifier,
|
||||
),
|
||||
increment_total=True,
|
||||
)
|
||||
|
||||
logger.debug('rebrick.lego.{method}("{identifier}")'.format(
|
||||
method=method,
|
||||
identifier=identifier,
|
||||
))
|
||||
|
||||
inventory = Rebrickable[BrickPart](
|
||||
method,
|
||||
identifier,
|
||||
BrickPart,
|
||||
socket=socket,
|
||||
brickset=brickset,
|
||||
minifigure=minifigure,
|
||||
).list()
|
||||
|
||||
# Process each part
|
||||
number_of_parts: int = 0
|
||||
for part in inventory:
|
||||
# Count the number of parts for minifigures
|
||||
if minifigure is not None:
|
||||
number_of_parts += part.fields.quantity
|
||||
|
||||
if not part.download(socket, refresh=refresh):
|
||||
return False
|
||||
|
||||
if minifigure is not None:
|
||||
minifigure.fields.number_of_parts = number_of_parts
|
||||
|
||||
except Exception as e:
|
||||
socket.fail(
|
||||
message='Error while importing {kind} {identifier} parts list: {error}'.format( # noqa: E501
|
||||
kind=kind,
|
||||
identifier=identifier,
|
||||
error=e,
|
||||
)
|
||||
)
|
||||
|
||||
logger.debug(traceback.format_exc())
|
||||
|
||||
return False
|
||||
|
||||
return True
|
@ -1,163 +0,0 @@
|
||||
import json
|
||||
from typing import Any, Callable, Generic, Type, TypeVar, TYPE_CHECKING
|
||||
from urllib.error import HTTPError
|
||||
|
||||
from flask import current_app
|
||||
from rebrick import lego
|
||||
|
||||
from .exceptions import NotFoundException, ErrorException
|
||||
if TYPE_CHECKING:
|
||||
from .minifigure import BrickMinifigure
|
||||
from .part import BrickPart
|
||||
from .rebrickable_set import RebrickableSet
|
||||
from .set import BrickSet
|
||||
from .socket import BrickSocket
|
||||
from .wish import BrickWish
|
||||
|
||||
T = TypeVar('T', 'RebrickableSet', 'BrickPart', 'BrickMinifigure', 'BrickWish')
|
||||
|
||||
|
||||
# An helper around the rebrick library, autoconverting
|
||||
class Rebrickable(Generic[T]):
|
||||
method: Callable
|
||||
method_name: str
|
||||
number: str
|
||||
model: Type[T]
|
||||
|
||||
brickset: 'BrickSet | None'
|
||||
instance: T | None
|
||||
kind: str
|
||||
minifigure: 'BrickMinifigure | None'
|
||||
socket: 'BrickSocket | None'
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
method: str,
|
||||
number: str,
|
||||
model: Type[T],
|
||||
/,
|
||||
*,
|
||||
brickset: 'BrickSet | None' = None,
|
||||
instance: T | None = None,
|
||||
minifigure: 'BrickMinifigure | None' = None,
|
||||
socket: 'BrickSocket | None' = None,
|
||||
):
|
||||
if not hasattr(lego, method):
|
||||
raise ErrorException('{method} is not a valid method for the rebrick.lego module'.format( # noqa: E501
|
||||
method=method,
|
||||
))
|
||||
|
||||
self.method = getattr(lego, method)
|
||||
self.method_name = method
|
||||
self.number = number
|
||||
self.model = model
|
||||
|
||||
self.brickset = brickset
|
||||
self.instance = instance
|
||||
self.minifigure = minifigure
|
||||
self.socket = socket
|
||||
|
||||
if self.minifigure is not None:
|
||||
self.kind = 'Minifigure'
|
||||
else:
|
||||
self.kind = 'Set'
|
||||
|
||||
# Get one element from the Rebrickable API
|
||||
def get(self, /) -> T:
|
||||
model_parameters = self.model_parameters()
|
||||
|
||||
if self.instance is None:
|
||||
self.instance = self.model(**model_parameters)
|
||||
|
||||
self.instance.ingest(self.model.from_rebrickable(
|
||||
self.load(),
|
||||
brickset=self.brickset,
|
||||
))
|
||||
|
||||
return self.instance
|
||||
|
||||
# Get paginated elements from the Rebrickable API
|
||||
def list(self, /) -> list[T]:
|
||||
model_parameters = self.model_parameters()
|
||||
|
||||
results: list[T] = []
|
||||
|
||||
# Bootstrap a first set of parameters
|
||||
parameters: dict[str, Any] | None = {
|
||||
'page_size': current_app.config['REBRICKABLE_PAGE_SIZE'],
|
||||
}
|
||||
|
||||
# Read all pages
|
||||
while parameters is not None:
|
||||
response = self.load(parameters=parameters)
|
||||
|
||||
# Grab the results
|
||||
if 'results' not in response:
|
||||
raise ErrorException('Missing "results" field from {method} for {number}'.format( # noqa: E501
|
||||
method=self.method_name,
|
||||
number=self.number,
|
||||
))
|
||||
|
||||
# Update the total
|
||||
if self.socket is not None:
|
||||
self.socket.total_progress(len(response['results']), add=True)
|
||||
|
||||
# Convert to object
|
||||
for result in response['results']:
|
||||
results.append(
|
||||
self.model(
|
||||
**model_parameters,
|
||||
record=self.model.from_rebrickable(result),
|
||||
)
|
||||
)
|
||||
|
||||
# Check for a next page
|
||||
if 'next' in response and response['next'] is not None:
|
||||
parameters['page'] = response['next']
|
||||
else:
|
||||
parameters = None
|
||||
|
||||
return results
|
||||
|
||||
# Load from the API
|
||||
def load(self, /, *, parameters: dict[str, Any] = {}) -> dict[str, Any]:
|
||||
# Inject the API key
|
||||
parameters['api_key'] = current_app.config['REBRICKABLE_API_KEY']
|
||||
|
||||
try:
|
||||
return json.loads(
|
||||
self.method(
|
||||
self.number,
|
||||
**parameters,
|
||||
).read()
|
||||
)
|
||||
|
||||
# HTTP errors
|
||||
except HTTPError as e:
|
||||
# Not found
|
||||
if e.code == 404:
|
||||
raise NotFoundException('{kind} {number} was not found on Rebrickable'.format( # noqa: E501
|
||||
kind=self.kind,
|
||||
number=self.number,
|
||||
))
|
||||
else:
|
||||
# Re-raise as ErrorException
|
||||
raise ErrorException(e)
|
||||
|
||||
# Other errors
|
||||
except Exception as e:
|
||||
# Re-raise as ErrorException
|
||||
raise ErrorException(e)
|
||||
|
||||
# Get the model parameters
|
||||
def model_parameters(self, /) -> dict[str, Any]:
|
||||
parameters: dict[str, Any] = {}
|
||||
|
||||
# Overload with objects
|
||||
if self.brickset is not None:
|
||||
parameters['brickset'] = self.brickset
|
||||
|
||||
if self.minifigure is not None:
|
||||
parameters['minifigure'] = self.minifigure
|
||||
|
||||
return parameters
|
@ -1,161 +0,0 @@
|
||||
import os
|
||||
from typing import TYPE_CHECKING
|
||||
from urllib.parse import urlparse
|
||||
|
||||
from flask import current_app, url_for
|
||||
import requests
|
||||
from shutil import copyfileobj
|
||||
|
||||
from .exceptions import DownloadException
|
||||
if TYPE_CHECKING:
|
||||
from .rebrickable_minifigure import RebrickableMinifigure
|
||||
from .rebrickable_part import RebrickablePart
|
||||
from .rebrickable_set import RebrickableSet
|
||||
|
||||
|
||||
# A set, part or minifigure image from Rebrickable
|
||||
class RebrickableImage(object):
|
||||
set: 'RebrickableSet'
|
||||
minifigure: 'RebrickableMinifigure | None'
|
||||
part: 'RebrickablePart | None'
|
||||
|
||||
extension: str | None
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
set: 'RebrickableSet',
|
||||
/,
|
||||
*,
|
||||
minifigure: 'RebrickableMinifigure | None' = None,
|
||||
part: 'RebrickablePart | None' = None,
|
||||
):
|
||||
# Save all objects
|
||||
self.set = set
|
||||
self.minifigure = minifigure
|
||||
self.part = part
|
||||
|
||||
# Currently everything is saved as 'jpg'
|
||||
self.extension = 'jpg'
|
||||
|
||||
# Guess the extension
|
||||
# url = self.url()
|
||||
# if url is not None:
|
||||
# _, extension = os.path.splitext(url)
|
||||
# # TODO: Add allowed extensions
|
||||
# if extension != '':
|
||||
# self.extension = extension
|
||||
|
||||
# Import the image from Rebrickable
|
||||
def download(self, /) -> None:
|
||||
path = self.path()
|
||||
|
||||
# Avoid doing anything if the file exists
|
||||
if os.path.exists(path):
|
||||
return
|
||||
|
||||
url = self.url()
|
||||
if url is None:
|
||||
return
|
||||
|
||||
# Grab the image
|
||||
response = requests.get(url, stream=True)
|
||||
if response.ok:
|
||||
with open(path, 'wb') as f:
|
||||
copyfileobj(response.raw, f)
|
||||
else:
|
||||
raise DownloadException('could not get image {id} at {url}'.format(
|
||||
id=self.id(),
|
||||
url=url,
|
||||
))
|
||||
|
||||
# Return the folder depending on the objects provided
|
||||
def folder(self, /) -> str:
|
||||
if self.part is not None:
|
||||
return current_app.config['PARTS_FOLDER']
|
||||
|
||||
if self.minifigure is not None:
|
||||
return current_app.config['MINIFIGURES_FOLDER']
|
||||
|
||||
return current_app.config['SETS_FOLDER']
|
||||
|
||||
# Return the id depending on the objects provided
|
||||
def id(self, /) -> str:
|
||||
if self.part is not None:
|
||||
if self.part.fields.image_id is None:
|
||||
return RebrickableImage.nil_name()
|
||||
else:
|
||||
return self.part.fields.image_id
|
||||
|
||||
if self.minifigure is not None:
|
||||
if self.minifigure.fields.image is None:
|
||||
return RebrickableImage.nil_minifigure_name()
|
||||
else:
|
||||
return self.minifigure.fields.figure
|
||||
|
||||
return self.set.fields.set
|
||||
|
||||
# Return the path depending on the objects provided
|
||||
def path(self, /) -> str:
|
||||
return os.path.join(
|
||||
current_app.static_folder, # type: ignore
|
||||
self.folder(),
|
||||
'{id}.{ext}'.format(id=self.id(), ext=self.extension),
|
||||
)
|
||||
|
||||
# Return the url depending on the objects provided
|
||||
def url(self, /) -> str:
|
||||
if self.part is not None:
|
||||
if self.part.fields.image is None:
|
||||
return current_app.config['REBRICKABLE_IMAGE_NIL']
|
||||
else:
|
||||
return self.part.fields.image
|
||||
|
||||
if self.minifigure is not None:
|
||||
if self.minifigure.fields.image is None:
|
||||
return current_app.config['REBRICKABLE_IMAGE_NIL_MINIFIGURE']
|
||||
else:
|
||||
return self.minifigure.fields.image
|
||||
|
||||
return self.set.fields.image
|
||||
|
||||
# Return the name of the nil image file
|
||||
@staticmethod
|
||||
def nil_name() -> str:
|
||||
filename, _ = os.path.splitext(
|
||||
os.path.basename(
|
||||
urlparse(current_app.config['REBRICKABLE_IMAGE_NIL']).path
|
||||
)
|
||||
)
|
||||
|
||||
return filename
|
||||
|
||||
# Return the name of the nil minifigure image file
|
||||
@staticmethod
|
||||
def nil_minifigure_name() -> str:
|
||||
filename, _ = os.path.splitext(
|
||||
os.path.basename(
|
||||
urlparse(current_app.config['REBRICKABLE_IMAGE_NIL_MINIFIGURE']).path # noqa: E501
|
||||
)
|
||||
)
|
||||
|
||||
return filename
|
||||
|
||||
# Return the static URL for an image given a name and folder
|
||||
@staticmethod
|
||||
def static_url(name: str, folder_name: str) -> str:
|
||||
folder: str = current_app.config[folder_name]
|
||||
|
||||
# /!\ Everything is saved as .jpg, even if it came from a .png
|
||||
# not changing this behaviour.
|
||||
|
||||
# Grab the extension
|
||||
# _, extension = os.path.splitext(self.part_img_url)
|
||||
extension = '.jpg'
|
||||
|
||||
# Compute the path
|
||||
path = os.path.join(folder, '{name}{ext}'.format(
|
||||
name=name,
|
||||
ext=extension,
|
||||
))
|
||||
|
||||
return url_for('static', filename=path)
|
@ -1,111 +0,0 @@
|
||||
from sqlite3 import Row
|
||||
from typing import Any, TYPE_CHECKING
|
||||
|
||||
from flask import current_app, url_for
|
||||
|
||||
from .exceptions import ErrorException
|
||||
from .rebrickable_image import RebrickableImage
|
||||
from .record import BrickRecord
|
||||
if TYPE_CHECKING:
|
||||
from .set import BrickSet
|
||||
|
||||
|
||||
# A minifigure from Rebrickable
|
||||
class RebrickableMinifigure(BrickRecord):
|
||||
brickset: 'BrickSet | None'
|
||||
|
||||
# Queries
|
||||
select_query: str = 'rebrickable/minifigure/select'
|
||||
insert_query: str = 'rebrickable/minifigure/insert'
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
brickset: 'BrickSet | None' = None,
|
||||
record: Row | dict[str, Any] | None = None
|
||||
):
|
||||
super().__init__()
|
||||
|
||||
# Save the brickset
|
||||
self.brickset = brickset
|
||||
|
||||
# Ingest the record if it has one
|
||||
if record is not None:
|
||||
self.ingest(record)
|
||||
|
||||
# Insert the minifigure from Rebrickable
|
||||
def insert_rebrickable(self, /) -> None:
|
||||
if self.brickset is None:
|
||||
raise ErrorException('Importing a minifigure from Rebrickable outside of a set is not supported') # noqa: E501
|
||||
|
||||
# Insert the Rebrickable minifigure to the database
|
||||
self.insert(
|
||||
commit=False,
|
||||
no_defer=True,
|
||||
override_query=RebrickableMinifigure.insert_query
|
||||
)
|
||||
|
||||
if not current_app.config['USE_REMOTE_IMAGES']:
|
||||
RebrickableImage(
|
||||
self.brickset,
|
||||
minifigure=self,
|
||||
).download()
|
||||
|
||||
# Return a dict with common SQL parameters for a minifigure
|
||||
def sql_parameters(self, /) -> dict[str, Any]:
|
||||
parameters = super().sql_parameters()
|
||||
|
||||
# Supplement from the brickset
|
||||
if self.brickset is not None and 'id' not in parameters:
|
||||
parameters['id'] = self.brickset.fields.id
|
||||
|
||||
return parameters
|
||||
|
||||
# Self url
|
||||
def url(self, /) -> str:
|
||||
return url_for(
|
||||
'minifigure.details',
|
||||
figure=self.fields.figure,
|
||||
)
|
||||
|
||||
# Compute the url for minifigure image
|
||||
def url_for_image(self, /) -> str:
|
||||
if not current_app.config['USE_REMOTE_IMAGES']:
|
||||
if self.fields.image is None:
|
||||
file = RebrickableImage.nil_minifigure_name()
|
||||
else:
|
||||
file = self.fields.figure
|
||||
|
||||
return RebrickableImage.static_url(file, 'MINIFIGURES_FOLDER')
|
||||
else:
|
||||
if self.fields.image is None:
|
||||
return current_app.config['REBRICKABLE_IMAGE_NIL_MINIFIGURE']
|
||||
else:
|
||||
return self.fields.image
|
||||
|
||||
# Compute the url for the rebrickable page
|
||||
def url_for_rebrickable(self, /) -> str:
|
||||
if current_app.config['REBRICKABLE_LINKS']:
|
||||
try:
|
||||
return current_app.config['REBRICKABLE_LINK_MINIFIGURE_PATTERN'].format( # noqa: E501
|
||||
number=self.fields.figure,
|
||||
)
|
||||
except Exception:
|
||||
pass
|
||||
|
||||
return ''
|
||||
|
||||
# Normalize from Rebrickable
|
||||
@staticmethod
|
||||
def from_rebrickable(data: dict[str, Any], /, **_) -> dict[str, Any]:
|
||||
# Extracting number
|
||||
number = int(str(data['set_num'])[5:])
|
||||
|
||||
return {
|
||||
'figure': str(data['set_num']),
|
||||
'number': int(number),
|
||||
'name': str(data['set_name']),
|
||||
'quantity': int(data['quantity']),
|
||||
'image': data['set_img_url'],
|
||||
}
|
@ -1,196 +0,0 @@
|
||||
import os
|
||||
from sqlite3 import Row
|
||||
from typing import Any, TYPE_CHECKING
|
||||
from urllib.parse import urlparse
|
||||
|
||||
from flask import current_app, url_for
|
||||
|
||||
from .exceptions import ErrorException
|
||||
from .rebrickable_image import RebrickableImage
|
||||
from .record import BrickRecord
|
||||
if TYPE_CHECKING:
|
||||
from .minifigure import BrickMinifigure
|
||||
from .set import BrickSet
|
||||
from .socket import BrickSocket
|
||||
|
||||
|
||||
# A part from Rebrickable
|
||||
class RebrickablePart(BrickRecord):
|
||||
socket: 'BrickSocket'
|
||||
brickset: 'BrickSet | None'
|
||||
minifigure: 'BrickMinifigure | None'
|
||||
|
||||
# Queries
|
||||
select_query: str = 'rebrickable/part/select'
|
||||
insert_query: str = 'rebrickable/part/insert'
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
brickset: 'BrickSet | None' = None,
|
||||
minifigure: 'BrickMinifigure | None' = None,
|
||||
record: Row | dict[str, Any] | None = None
|
||||
):
|
||||
super().__init__()
|
||||
|
||||
# Save the brickset
|
||||
self.brickset = brickset
|
||||
|
||||
# Save the minifigure
|
||||
self.minifigure = minifigure
|
||||
|
||||
# Ingest the record if it has one
|
||||
if record is not None:
|
||||
self.ingest(record)
|
||||
|
||||
# Insert the part from Rebrickable
|
||||
def insert_rebrickable(self, /) -> None:
|
||||
if self.brickset is None:
|
||||
raise ErrorException('Importing a part from Rebrickable outside of a set is not supported') # noqa: E501
|
||||
|
||||
# Insert the Rebrickable part to the database
|
||||
self.insert(
|
||||
commit=False,
|
||||
no_defer=True,
|
||||
override_query=RebrickablePart.insert_query
|
||||
)
|
||||
|
||||
if not current_app.config['USE_REMOTE_IMAGES']:
|
||||
RebrickableImage(
|
||||
self.brickset,
|
||||
minifigure=self.minifigure,
|
||||
part=self,
|
||||
).download()
|
||||
|
||||
# Return a dict with common SQL parameters for a part
|
||||
def sql_parameters(self, /) -> dict[str, Any]:
|
||||
parameters = super().sql_parameters()
|
||||
|
||||
# Set id
|
||||
if self.brickset is not None:
|
||||
parameters['id'] = self.brickset.fields.id
|
||||
|
||||
# Use the minifigure number if present,
|
||||
if self.minifigure is not None:
|
||||
parameters['figure'] = self.minifigure.fields.figure
|
||||
else:
|
||||
parameters['figure'] = None
|
||||
|
||||
return parameters
|
||||
|
||||
# Self url
|
||||
def url(self, /) -> str:
|
||||
return url_for(
|
||||
'part.details',
|
||||
part=self.fields.part,
|
||||
color=self.fields.color,
|
||||
)
|
||||
|
||||
# Compute the url for the bricklink page
|
||||
def url_for_bricklink(self, /) -> str:
|
||||
if current_app.config['BRICKLINK_LINKS']:
|
||||
try:
|
||||
return current_app.config['BRICKLINK_LINK_PART_PATTERN'].format( # noqa: E501
|
||||
part=self.fields.part,
|
||||
)
|
||||
except Exception:
|
||||
pass
|
||||
|
||||
return ''
|
||||
|
||||
# Compute the url for the part image
|
||||
def url_for_image(self, /) -> str:
|
||||
if not current_app.config['USE_REMOTE_IMAGES']:
|
||||
if self.fields.image is None:
|
||||
file = RebrickableImage.nil_name()
|
||||
else:
|
||||
file = self.fields.image_id
|
||||
|
||||
return RebrickableImage.static_url(file, 'PARTS_FOLDER')
|
||||
else:
|
||||
if self.fields.image is None:
|
||||
return current_app.config['REBRICKABLE_IMAGE_NIL']
|
||||
else:
|
||||
return self.fields.image
|
||||
|
||||
# Compute the url for the original of the printed part
|
||||
def url_for_print(self, /) -> str:
|
||||
if self.fields.print is not None:
|
||||
return url_for(
|
||||
'part.details',
|
||||
part=self.fields.print,
|
||||
color=self.fields.color,
|
||||
)
|
||||
else:
|
||||
return ''
|
||||
|
||||
# Compute the url for the rebrickable page
|
||||
def url_for_rebrickable(self, /) -> str:
|
||||
if current_app.config['REBRICKABLE_LINKS']:
|
||||
try:
|
||||
if self.fields.url is not None:
|
||||
# The URL does not contain color info...
|
||||
return '{url}{color}'.format(
|
||||
url=self.fields.url,
|
||||
color=self.fields.color
|
||||
)
|
||||
else:
|
||||
return current_app.config['REBRICKABLE_LINK_PART_PATTERN'].format( # noqa: E501
|
||||
part=self.fields.part,
|
||||
color=self.fields.color,
|
||||
)
|
||||
except Exception:
|
||||
pass
|
||||
|
||||
return ''
|
||||
|
||||
# Normalize from Rebrickable
|
||||
@staticmethod
|
||||
def from_rebrickable(
|
||||
data: dict[str, Any],
|
||||
/,
|
||||
*,
|
||||
brickset: 'BrickSet | None' = None,
|
||||
minifigure: 'BrickMinifigure | None' = None,
|
||||
**_,
|
||||
) -> dict[str, Any]:
|
||||
record = {
|
||||
'id': None,
|
||||
'figure': None,
|
||||
'part': data['part']['part_num'],
|
||||
'color': data['color']['id'],
|
||||
'spare': data['is_spare'],
|
||||
'quantity': data['quantity'],
|
||||
'rebrickable_inventory': data['id'],
|
||||
'element': data['element_id'],
|
||||
'color_id': data['color']['id'],
|
||||
'color_name': data['color']['name'],
|
||||
'color_rgb': data['color']['rgb'],
|
||||
'color_transparent': data['color']['is_trans'],
|
||||
'name': data['part']['name'],
|
||||
'category': data['part']['part_cat_id'],
|
||||
'image': data['part']['part_img_url'],
|
||||
'image_id': None,
|
||||
'url': data['part']['part_url'],
|
||||
'print': data['part']['print_of']
|
||||
}
|
||||
|
||||
if brickset is not None:
|
||||
record['id'] = brickset.fields.id
|
||||
|
||||
if minifigure is not None:
|
||||
record['figure'] = minifigure.fields.figure
|
||||
|
||||
# Extract the file name
|
||||
if record['image'] is not None:
|
||||
image_id, _ = os.path.splitext(
|
||||
os.path.basename(
|
||||
urlparse(record['image']).path
|
||||
)
|
||||
)
|
||||
|
||||
if image_id is not None or image_id != '':
|
||||
record['image_id'] = image_id
|
||||
|
||||
return record
|
@ -1,203 +0,0 @@
|
||||
import logging
|
||||
from sqlite3 import Row
|
||||
import traceback
|
||||
from typing import Any, Self, TYPE_CHECKING
|
||||
|
||||
from flask import current_app, url_for
|
||||
|
||||
from .exceptions import ErrorException, NotFoundException
|
||||
from .instructions import BrickInstructions
|
||||
from .parser import parse_set
|
||||
from .rebrickable import Rebrickable
|
||||
from .rebrickable_image import RebrickableImage
|
||||
from .record import BrickRecord
|
||||
from .theme_list import BrickThemeList
|
||||
if TYPE_CHECKING:
|
||||
from .socket import BrickSocket
|
||||
from .theme import BrickTheme
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
# A set from Rebrickable
|
||||
class RebrickableSet(BrickRecord):
|
||||
theme: 'BrickTheme'
|
||||
instructions: list[BrickInstructions]
|
||||
|
||||
# Flags
|
||||
resolve_instructions: bool = True
|
||||
|
||||
# Queries
|
||||
select_query: str = 'rebrickable/set/select'
|
||||
insert_query: str = 'rebrickable/set/insert'
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
record: Row | dict[str, Any] | None = None
|
||||
):
|
||||
super().__init__()
|
||||
|
||||
# Placeholders
|
||||
self.instructions = []
|
||||
|
||||
# Ingest the record if it has one
|
||||
if record is not None:
|
||||
self.ingest(record)
|
||||
|
||||
# Insert the set from Rebrickable
|
||||
def insert_rebrickable(self, /) -> None:
|
||||
# Insert the Rebrickable set to the database
|
||||
self.insert(
|
||||
commit=False,
|
||||
no_defer=True,
|
||||
override_query=RebrickableSet.insert_query
|
||||
)
|
||||
|
||||
if not current_app.config['USE_REMOTE_IMAGES']:
|
||||
RebrickableImage(self).download()
|
||||
|
||||
# Ingest a set
|
||||
def ingest(self, record: Row | dict[str, Any], /):
|
||||
super().ingest(record)
|
||||
|
||||
# Resolve theme
|
||||
if not hasattr(self.fields, 'theme_id'):
|
||||
self.fields.theme_id = 0
|
||||
|
||||
self.theme = BrickThemeList().get(self.fields.theme_id)
|
||||
|
||||
# Resolve instructions
|
||||
if self.resolve_instructions:
|
||||
# Not idead, avoiding cyclic import
|
||||
from .instructions_list import BrickInstructionsList
|
||||
|
||||
if self.fields.set is not None:
|
||||
self.instructions = BrickInstructionsList().get(
|
||||
self.fields.set
|
||||
)
|
||||
|
||||
# Load the set from Rebrickable
|
||||
def load(
|
||||
self,
|
||||
socket: 'BrickSocket',
|
||||
data: dict[str, Any],
|
||||
/,
|
||||
*,
|
||||
from_download=False,
|
||||
) -> bool:
|
||||
# Reset the progress
|
||||
socket.progress_count = 0
|
||||
socket.progress_total = 2
|
||||
|
||||
try:
|
||||
socket.auto_progress(message='Parsing set number')
|
||||
set = parse_set(str(data['set']))
|
||||
|
||||
socket.auto_progress(
|
||||
message='Set {set}: loading from Rebrickable'.format(
|
||||
set=set,
|
||||
),
|
||||
)
|
||||
|
||||
logger.debug('rebrick.lego.get_set("{set}")'.format(
|
||||
set=set,
|
||||
))
|
||||
|
||||
Rebrickable[RebrickableSet](
|
||||
'get_set',
|
||||
set,
|
||||
RebrickableSet,
|
||||
instance=self,
|
||||
).get()
|
||||
|
||||
socket.emit('SET_LOADED', self.short(
|
||||
from_download=from_download
|
||||
))
|
||||
|
||||
if not from_download:
|
||||
socket.complete(
|
||||
message='Set {set}: loaded from Rebrickable'.format(
|
||||
set=self.fields.set
|
||||
)
|
||||
)
|
||||
|
||||
return True
|
||||
|
||||
except Exception as e:
|
||||
socket.fail(
|
||||
message='Could not load the set from Rebrickable: {error}. Data: {data}'.format( # noqa: E501
|
||||
error=str(e),
|
||||
data=data,
|
||||
)
|
||||
)
|
||||
|
||||
if not isinstance(e, (NotFoundException, ErrorException)):
|
||||
logger.debug(traceback.format_exc())
|
||||
|
||||
return False
|
||||
|
||||
# Select a specific set (with a set)
|
||||
def select_specific(self, set: str, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.set = set
|
||||
|
||||
# Load from database
|
||||
if not self.select():
|
||||
raise NotFoundException(
|
||||
'Set with set {set} was not found in the database'.format(
|
||||
set=self.fields.set,
|
||||
),
|
||||
)
|
||||
|
||||
return self
|
||||
|
||||
# Return a short form of the Rebrickable set
|
||||
def short(self, /, *, from_download: bool = False) -> dict[str, Any]:
|
||||
return {
|
||||
'download': from_download,
|
||||
'image': self.fields.image,
|
||||
'name': self.fields.name,
|
||||
'set': self.fields.set,
|
||||
}
|
||||
|
||||
# Compute the url for the set image
|
||||
def url_for_image(self, /) -> str:
|
||||
if not current_app.config['USE_REMOTE_IMAGES']:
|
||||
return RebrickableImage.static_url(
|
||||
self.fields.set,
|
||||
'SETS_FOLDER'
|
||||
)
|
||||
else:
|
||||
return self.fields.image
|
||||
|
||||
# Compute the url for the rebrickable page
|
||||
def url_for_rebrickable(self, /) -> str:
|
||||
if current_app.config['REBRICKABLE_LINKS']:
|
||||
return self.fields.url
|
||||
|
||||
return ''
|
||||
|
||||
# Compute the url for the refresh button
|
||||
def url_for_refresh(self, /) -> str:
|
||||
return url_for('set.refresh', set=self.fields.set)
|
||||
|
||||
# Normalize from Rebrickable
|
||||
@staticmethod
|
||||
def from_rebrickable(data: dict[str, Any], /, **_) -> dict[str, Any]:
|
||||
# Extracting version and number
|
||||
number, _, version = str(data['set_num']).partition('-')
|
||||
|
||||
return {
|
||||
'set': str(data['set_num']),
|
||||
'number': int(number),
|
||||
'version': int(version),
|
||||
'name': str(data['name']),
|
||||
'year': int(data['year']),
|
||||
'theme_id': int(data['theme_id']),
|
||||
'number_of_parts': int(data['num_parts']),
|
||||
'image': str(data['set_img_url']),
|
||||
'url': str(data['set_url']),
|
||||
'last_modified': str(data['last_modified_dt']),
|
||||
}
|
@ -1,34 +0,0 @@
|
||||
from typing import Self
|
||||
|
||||
from .rebrickable_set import RebrickableSet
|
||||
from .record_list import BrickRecordList
|
||||
|
||||
|
||||
# All the rebrickable sets from the database
|
||||
class RebrickableSetList(BrickRecordList[RebrickableSet]):
|
||||
|
||||
# Queries
|
||||
select_query: str = 'rebrickable/set/list'
|
||||
refresh_query: str = 'rebrickable/set/need_refresh'
|
||||
|
||||
# All the sets
|
||||
def all(self, /) -> Self:
|
||||
# Load the sets from the database
|
||||
for record in self.select():
|
||||
rebrickable_set = RebrickableSet(record=record)
|
||||
|
||||
self.records.append(rebrickable_set)
|
||||
|
||||
return self
|
||||
|
||||
# Sets needing refresh
|
||||
def need_refresh(self, /) -> Self:
|
||||
# Load the sets from the database
|
||||
for record in self.select(
|
||||
override_query=self.refresh_query
|
||||
):
|
||||
rebrickable_set = RebrickableSet(record=record)
|
||||
|
||||
self.records.append(rebrickable_set)
|
||||
|
||||
return self
|
@ -1,88 +0,0 @@
|
||||
from sqlite3 import Row
|
||||
from typing import Any, ItemsView
|
||||
|
||||
from .fields import BrickRecordFields
|
||||
from .sql import BrickSQL
|
||||
|
||||
|
||||
# SQLite record
|
||||
class BrickRecord(object):
|
||||
select_query: str
|
||||
insert_query: str
|
||||
|
||||
# Fields
|
||||
fields: BrickRecordFields
|
||||
|
||||
def __init__(self, /):
|
||||
self.fields = BrickRecordFields()
|
||||
|
||||
# Load from a record
|
||||
def ingest(self, record: Row | dict[str, Any], /) -> None:
|
||||
# Brutally ingest the record
|
||||
for key in record.keys():
|
||||
setattr(self.fields, key, record[key])
|
||||
|
||||
# Insert into the database
|
||||
# If we do not commit immediately, we defer the execute() call
|
||||
def insert(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
commit=True,
|
||||
no_defer=False,
|
||||
override_query: str | None = None
|
||||
) -> None:
|
||||
if override_query:
|
||||
query = override_query
|
||||
else:
|
||||
query = self.insert_query
|
||||
|
||||
database = BrickSQL()
|
||||
database.execute(
|
||||
query,
|
||||
parameters=self.sql_parameters(),
|
||||
defer=not commit and not no_defer,
|
||||
)
|
||||
|
||||
if commit:
|
||||
database.commit()
|
||||
|
||||
# Shorthand to field items
|
||||
def items(self, /) -> ItemsView[str, Any]:
|
||||
return self.fields.__dict__.items()
|
||||
|
||||
# Get from the database using the query
|
||||
def select(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
override_query: str | None = None,
|
||||
**context: Any
|
||||
) -> bool:
|
||||
if override_query:
|
||||
query = override_query
|
||||
else:
|
||||
query = self.select_query
|
||||
|
||||
record = BrickSQL().fetchone(
|
||||
query,
|
||||
parameters=self.sql_parameters(),
|
||||
**context
|
||||
)
|
||||
|
||||
# Ingest the record
|
||||
if record is not None:
|
||||
self.ingest(record)
|
||||
|
||||
return True
|
||||
|
||||
else:
|
||||
return False
|
||||
|
||||
# Generic SQL parameters from fields
|
||||
def sql_parameters(self, /) -> dict[str, Any]:
|
||||
parameters: dict[str, Any] = {}
|
||||
for name, value in self.items():
|
||||
parameters[name] = value
|
||||
|
||||
return parameters
|
@ -1,90 +0,0 @@
|
||||
from sqlite3 import Row
|
||||
from typing import Any, Generator, Generic, ItemsView, TypeVar, TYPE_CHECKING
|
||||
|
||||
from .fields import BrickRecordFields
|
||||
from .sql import BrickSQL
|
||||
if TYPE_CHECKING:
|
||||
from .minifigure import BrickMinifigure
|
||||
from .part import BrickPart
|
||||
from .rebrickable_set import RebrickableSet
|
||||
from .set import BrickSet
|
||||
from .set_owner import BrickSetOwner
|
||||
from .set_purchase_location import BrickSetPurchaseLocation
|
||||
from .set_status import BrickSetStatus
|
||||
from .set_storage import BrickSetStorage
|
||||
from .set_tag import BrickSetTag
|
||||
from .wish import BrickWish
|
||||
from .wish_owner import BrickWishOwner
|
||||
|
||||
T = TypeVar(
|
||||
'T',
|
||||
'BrickMinifigure',
|
||||
'BrickPart',
|
||||
'BrickSet',
|
||||
'BrickSetOwner',
|
||||
'BrickSetPurchaseLocation',
|
||||
'BrickSetStatus',
|
||||
'BrickSetStorage',
|
||||
'BrickSetTag',
|
||||
'BrickWish',
|
||||
'BrickWishOwner',
|
||||
'RebrickableSet'
|
||||
)
|
||||
|
||||
|
||||
# SQLite records
|
||||
class BrickRecordList(Generic[T]):
|
||||
select_query: str
|
||||
records: list[T]
|
||||
|
||||
# Fields
|
||||
fields: BrickRecordFields
|
||||
|
||||
def __init__(self, /):
|
||||
self.fields = BrickRecordFields()
|
||||
self.records = []
|
||||
|
||||
# Shorthand to field items
|
||||
def items(self, /) -> ItemsView[str, Any]:
|
||||
return self.fields.__dict__.items()
|
||||
|
||||
# Get all from the database
|
||||
def select(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
override_query: str | None = None,
|
||||
order: str | None = None,
|
||||
limit: int | None = None,
|
||||
**context: Any,
|
||||
) -> list[Row]:
|
||||
# Select the query
|
||||
if override_query:
|
||||
query = override_query
|
||||
else:
|
||||
query = self.select_query
|
||||
|
||||
return BrickSQL().fetchall(
|
||||
query,
|
||||
parameters=self.sql_parameters(),
|
||||
order=order,
|
||||
limit=limit,
|
||||
**context
|
||||
)
|
||||
|
||||
# Generic SQL parameters from fields
|
||||
def sql_parameters(self, /) -> dict[str, Any]:
|
||||
parameters: dict[str, Any] = {}
|
||||
for name, value in self.items():
|
||||
parameters[name] = value
|
||||
|
||||
return parameters
|
||||
|
||||
# Make the list iterable
|
||||
def __iter__(self, /) -> Generator[T, Any, Any]:
|
||||
for record in self.records:
|
||||
yield record
|
||||
|
||||
# Make the list measurable
|
||||
def __len__(self, /) -> int:
|
||||
return len(self.records)
|
@ -1,43 +0,0 @@
|
||||
from .instructions_list import BrickInstructionsList
|
||||
from .retired_list import BrickRetiredList
|
||||
from .set_owner_list import BrickSetOwnerList
|
||||
from .set_purchase_location_list import BrickSetPurchaseLocationList
|
||||
from .set_status_list import BrickSetStatusList
|
||||
from .set_storage_list import BrickSetStorageList
|
||||
from .set_tag_list import BrickSetTagList
|
||||
from .theme_list import BrickThemeList
|
||||
from .wish_owner_list import BrickWishOwnerList
|
||||
|
||||
|
||||
# Reload everything related to a database after an operation
|
||||
def reload() -> None:
|
||||
# Failsafe
|
||||
try:
|
||||
# Reload the instructions
|
||||
BrickInstructionsList(force=True)
|
||||
|
||||
# Reload the set owners
|
||||
BrickSetOwnerList.new(force=True)
|
||||
|
||||
# Reload the set purchase locations
|
||||
BrickSetPurchaseLocationList.new(force=True)
|
||||
|
||||
# Reload the set statuses
|
||||
BrickSetStatusList.new(force=True)
|
||||
|
||||
# Reload the set storages
|
||||
BrickSetStorageList.new(force=True)
|
||||
|
||||
# Reload the set tags
|
||||
BrickSetTagList.new(force=True)
|
||||
|
||||
# Reload retired sets
|
||||
BrickRetiredList(force=True)
|
||||
|
||||
# Reload themes
|
||||
BrickThemeList(force=True)
|
||||
|
||||
# Reload the wish owners
|
||||
BrickWishOwnerList.new(force=True)
|
||||
except Exception:
|
||||
pass
|
@ -1,28 +0,0 @@
|
||||
# Lego retired set
|
||||
class BrickRetired(object):
|
||||
theme: str
|
||||
subtheme: str
|
||||
number: str
|
||||
name: str
|
||||
age: str
|
||||
piece_count: str
|
||||
retirement_date: str
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
theme: str,
|
||||
subtheme: str,
|
||||
number: str,
|
||||
name: str,
|
||||
age: str,
|
||||
piece_count: str,
|
||||
retirement_date: str,
|
||||
*_,
|
||||
):
|
||||
self.theme = theme
|
||||
self.subtheme = subtheme
|
||||
self.number = number
|
||||
self.name = name
|
||||
self.age = age
|
||||
self.piece_count = piece_count
|
||||
self.retirement_date = retirement_date
|
@ -1,105 +0,0 @@
|
||||
from datetime import datetime, timezone
|
||||
import csv
|
||||
import gzip
|
||||
import logging
|
||||
import os
|
||||
from shutil import copyfileobj
|
||||
|
||||
from flask import current_app, g
|
||||
import humanize
|
||||
import requests
|
||||
|
||||
from .exceptions import ErrorException
|
||||
from .retired import BrickRetired
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
# Lego retired sets
|
||||
class BrickRetiredList(object):
|
||||
retired: dict[str, BrickRetired]
|
||||
mtime: datetime | None
|
||||
size: int | None
|
||||
exception: Exception | None
|
||||
|
||||
def __init__(self, /, *, force: bool = False):
|
||||
# Load sets only if there is none already loaded
|
||||
retired = getattr(self, 'retired', None)
|
||||
|
||||
if retired is None or force:
|
||||
logger.info('Loading retired sets list')
|
||||
|
||||
BrickRetiredList.retired = {}
|
||||
|
||||
# Try to read the themes from a CSV file
|
||||
try:
|
||||
with open(current_app.config['RETIRED_SETS_PATH'], newline='') as themes_file: # noqa: E501
|
||||
themes_reader = csv.reader(themes_file)
|
||||
|
||||
# Ignore the header
|
||||
next(themes_reader, None)
|
||||
|
||||
for row in themes_reader:
|
||||
retired = BrickRetired(*row)
|
||||
BrickRetiredList.retired[retired.number] = retired
|
||||
|
||||
# File stats
|
||||
stat = os.stat(current_app.config['RETIRED_SETS_PATH'])
|
||||
BrickRetiredList.size = stat.st_size
|
||||
BrickRetiredList.mtime = datetime.fromtimestamp(stat.st_mtime, tz=timezone.utc) # noqa: E501
|
||||
|
||||
BrickRetiredList.exception = None
|
||||
|
||||
# Ignore errors
|
||||
except Exception as e:
|
||||
BrickRetiredList.exception = e
|
||||
BrickRetiredList.size = None
|
||||
BrickRetiredList.mtime = None
|
||||
|
||||
# Get a retirement date for a set
|
||||
def get(self, number: str, /) -> str:
|
||||
if number in self.retired:
|
||||
return self.retired[number].retirement_date
|
||||
else:
|
||||
number, _, _ = number.partition('-')
|
||||
|
||||
if number in self.retired:
|
||||
return self.retired[number].retirement_date
|
||||
else:
|
||||
return ''
|
||||
|
||||
# Display the size in a human format
|
||||
def human_size(self) -> str:
|
||||
if self.size is not None:
|
||||
return humanize.naturalsize(self.size)
|
||||
else:
|
||||
return ''
|
||||
|
||||
# Display the time in a human format
|
||||
def human_time(self) -> str:
|
||||
if self.mtime is not None:
|
||||
return self.mtime.astimezone(g.timezone).strftime(
|
||||
current_app.config['FILE_DATETIME_FORMAT']
|
||||
)
|
||||
else:
|
||||
return ''
|
||||
|
||||
# Update the file
|
||||
@staticmethod
|
||||
def update() -> None:
|
||||
response = requests.get(
|
||||
current_app.config['RETIRED_SETS_FILE_URL'],
|
||||
stream=True,
|
||||
)
|
||||
|
||||
if not response.ok:
|
||||
raise ErrorException('An error occured while downloading the retired sets file ({code})'.format( # noqa: E501
|
||||
code=response.status_code
|
||||
))
|
||||
|
||||
content = gzip.GzipFile(fileobj=response.raw)
|
||||
|
||||
with open(current_app.config['RETIRED_SETS_PATH'], 'wb') as f:
|
||||
copyfileobj(content, f)
|
||||
|
||||
logger.info('Retired sets list updated')
|
@ -1,341 +0,0 @@
|
||||
from datetime import datetime
|
||||
import logging
|
||||
import traceback
|
||||
from typing import Any, Self, TYPE_CHECKING
|
||||
from uuid import uuid4
|
||||
|
||||
from flask import current_app, url_for
|
||||
|
||||
from .exceptions import NotFoundException, DatabaseException, ErrorException
|
||||
from .minifigure_list import BrickMinifigureList
|
||||
from .part_list import BrickPartList
|
||||
from .rebrickable_set import RebrickableSet
|
||||
from .set_owner_list import BrickSetOwnerList
|
||||
from .set_purchase_location_list import BrickSetPurchaseLocationList
|
||||
from .set_status_list import BrickSetStatusList
|
||||
from .set_storage_list import BrickSetStorageList
|
||||
from .set_tag_list import BrickSetTagList
|
||||
from .sql import BrickSQL
|
||||
if TYPE_CHECKING:
|
||||
from .socket import BrickSocket
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
|
||||
# Lego brick set
|
||||
class BrickSet(RebrickableSet):
|
||||
# Queries
|
||||
select_query: str = 'set/select/full'
|
||||
light_query: str = 'set/select/light'
|
||||
insert_query: str = 'set/insert'
|
||||
update_purchase_date_query: str = 'set/update/purchase_date'
|
||||
update_purchase_price_query: str = 'set/update/purchase_price'
|
||||
|
||||
# Delete a set
|
||||
def delete(self, /) -> None:
|
||||
BrickSQL().executescript(
|
||||
'set/delete/set',
|
||||
id=self.fields.id
|
||||
)
|
||||
|
||||
# Import a set into the database
|
||||
def download(self, socket: 'BrickSocket', data: dict[str, Any], /) -> bool:
|
||||
# Load the set
|
||||
if not self.load(socket, data, from_download=True):
|
||||
return False
|
||||
|
||||
try:
|
||||
# Insert into the database
|
||||
socket.auto_progress(
|
||||
message='Set {set}: inserting into database'.format(
|
||||
set=self.fields.set
|
||||
),
|
||||
increment_total=True,
|
||||
)
|
||||
|
||||
# Grabbing the refresh flag
|
||||
refresh: bool = bool(data.get('refresh', False))
|
||||
|
||||
# Generate an UUID for self
|
||||
self.fields.id = str(uuid4())
|
||||
|
||||
if not refresh:
|
||||
# Save the storage
|
||||
storage = BrickSetStorageList.get(
|
||||
data.get('storage', ''),
|
||||
allow_none=True
|
||||
)
|
||||
self.fields.storage = storage.fields.id
|
||||
|
||||
# Save the purchase location
|
||||
purchase_location = BrickSetPurchaseLocationList.get(
|
||||
data.get('purchase_location', ''),
|
||||
allow_none=True
|
||||
)
|
||||
self.fields.purchase_location = purchase_location.fields.id
|
||||
|
||||
# Insert into database
|
||||
self.insert(commit=False)
|
||||
|
||||
# Save the owners
|
||||
owners: list[str] = list(data.get('owners', []))
|
||||
|
||||
for id in owners:
|
||||
owner = BrickSetOwnerList.get(id)
|
||||
owner.update_set_state(self, state=True)
|
||||
|
||||
# Save the tags
|
||||
tags: list[str] = list(data.get('tags', []))
|
||||
|
||||
for id in tags:
|
||||
tag = BrickSetTagList.get(id)
|
||||
tag.update_set_state(self, state=True)
|
||||
|
||||
# Insert the rebrickable set into database
|
||||
self.insert_rebrickable()
|
||||
|
||||
# Load the inventory
|
||||
if not BrickPartList.download(socket, self, refresh=refresh):
|
||||
return False
|
||||
|
||||
# Load the minifigures
|
||||
if not BrickMinifigureList.download(socket, self, refresh=refresh):
|
||||
return False
|
||||
|
||||
# Commit the transaction to the database
|
||||
socket.auto_progress(
|
||||
message='Set {set}: writing to the database'.format(
|
||||
set=self.fields.set
|
||||
),
|
||||
increment_total=True,
|
||||
)
|
||||
|
||||
BrickSQL().commit()
|
||||
|
||||
if refresh:
|
||||
# Info
|
||||
logger.info('Set {set}: imported (id: {id})'.format(
|
||||
set=self.fields.set,
|
||||
id=self.fields.id,
|
||||
))
|
||||
|
||||
# Complete
|
||||
socket.complete(
|
||||
message='Set {set}: refreshed'.format( # noqa: E501
|
||||
set=self.fields.set,
|
||||
),
|
||||
download=True
|
||||
)
|
||||
else:
|
||||
# Info
|
||||
logger.info('Set {set}: refreshed'.format(
|
||||
set=self.fields.set,
|
||||
))
|
||||
|
||||
# Complete
|
||||
socket.complete(
|
||||
message='Set {set}: imported (<a href="{url}">Go to the set</a>)'.format( # noqa: E501
|
||||
set=self.fields.set,
|
||||
url=self.url()
|
||||
),
|
||||
download=True
|
||||
)
|
||||
|
||||
except Exception as e:
|
||||
socket.fail(
|
||||
message='Error while importing set {set}: {error}'.format(
|
||||
set=self.fields.set,
|
||||
error=e,
|
||||
)
|
||||
)
|
||||
|
||||
logger.debug(traceback.format_exc())
|
||||
|
||||
return False
|
||||
|
||||
return True
|
||||
|
||||
# Purchase date
|
||||
def purchase_date(self, /, *, standard: bool = False) -> str:
|
||||
if self.fields.purchase_date is not None:
|
||||
time = datetime.fromtimestamp(self.fields.purchase_date)
|
||||
|
||||
if standard:
|
||||
return time.strftime('%Y/%m/%d')
|
||||
else:
|
||||
return time.strftime(
|
||||
current_app.config['PURCHASE_DATE_FORMAT']
|
||||
)
|
||||
else:
|
||||
return ''
|
||||
|
||||
# Purchase price with currency
|
||||
def purchase_price(self, /) -> str:
|
||||
if self.fields.purchase_price is not None:
|
||||
return '{price}{currency}'.format(
|
||||
price=self.fields.purchase_price,
|
||||
currency=current_app.config['PURCHASE_CURRENCY']
|
||||
)
|
||||
else:
|
||||
return ''
|
||||
|
||||
# Minifigures
|
||||
def minifigures(self, /) -> BrickMinifigureList:
|
||||
return BrickMinifigureList().from_set(self)
|
||||
|
||||
# Parts
|
||||
def parts(self, /) -> BrickPartList:
|
||||
return BrickPartList().list_specific(self)
|
||||
|
||||
# Select a light set (with an id)
|
||||
def select_light(self, id: str, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.id = id
|
||||
|
||||
# Load from database
|
||||
if not self.select(override_query=self.light_query):
|
||||
raise NotFoundException(
|
||||
'Set with ID {id} was not found in the database'.format(
|
||||
id=self.fields.id,
|
||||
),
|
||||
)
|
||||
|
||||
return self
|
||||
|
||||
# Select a specific set (with an id)
|
||||
def select_specific(self, id: str, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.id = id
|
||||
|
||||
# Load from database
|
||||
if not self.select(
|
||||
owners=BrickSetOwnerList.as_columns(),
|
||||
statuses=BrickSetStatusList.as_columns(all=True),
|
||||
tags=BrickSetTagList.as_columns(),
|
||||
):
|
||||
raise NotFoundException(
|
||||
'Set with ID {id} was not found in the database'.format(
|
||||
id=self.fields.id,
|
||||
),
|
||||
)
|
||||
|
||||
return self
|
||||
|
||||
# Update the purchase date
|
||||
def update_purchase_date(self, json: Any | None, /) -> Any:
|
||||
value = json.get('value', None) # type: ignore
|
||||
|
||||
try:
|
||||
if value == '':
|
||||
value = None
|
||||
|
||||
if value is not None:
|
||||
value = datetime.strptime(value, '%Y/%m/%d').timestamp()
|
||||
except Exception:
|
||||
raise ErrorException('{value} is not a date'.format(
|
||||
value=value,
|
||||
))
|
||||
|
||||
self.fields.purchase_date = value
|
||||
|
||||
rows, _ = BrickSQL().execute_and_commit(
|
||||
self.update_purchase_date_query,
|
||||
parameters=self.sql_parameters()
|
||||
)
|
||||
|
||||
if rows != 1:
|
||||
raise DatabaseException('Could not update the purchase date for set {set} ({id})'.format( # noqa: E501
|
||||
set=self.fields.set,
|
||||
id=self.fields.id,
|
||||
))
|
||||
|
||||
# Info
|
||||
logger.info('Purchase date changed to "{value}" for set {set} ({id})'.format( # noqa: E501
|
||||
value=value,
|
||||
set=self.fields.set,
|
||||
id=self.fields.id,
|
||||
))
|
||||
|
||||
return value
|
||||
|
||||
# Update the purchase price
|
||||
def update_purchase_price(self, json: Any | None, /) -> Any:
|
||||
value = json.get('value', None) # type: ignore
|
||||
|
||||
try:
|
||||
if value == '':
|
||||
value = None
|
||||
|
||||
if value is not None:
|
||||
value = float(value)
|
||||
except Exception:
|
||||
raise ErrorException('{value} is not a number or empty'.format(
|
||||
value=value,
|
||||
))
|
||||
|
||||
self.fields.purchase_price = value
|
||||
|
||||
rows, _ = BrickSQL().execute_and_commit(
|
||||
self.update_purchase_price_query,
|
||||
parameters=self.sql_parameters()
|
||||
)
|
||||
|
||||
if rows != 1:
|
||||
raise DatabaseException('Could not update the purchase price for set {set} ({id})'.format( # noqa: E501
|
||||
set=self.fields.set,
|
||||
id=self.fields.id,
|
||||
))
|
||||
|
||||
# Info
|
||||
logger.info('Purchase price changed to "{value}" for set {set} ({id})'.format( # noqa: E501
|
||||
value=value,
|
||||
set=self.fields.set,
|
||||
id=self.fields.id,
|
||||
))
|
||||
|
||||
return value
|
||||
|
||||
# Self url
|
||||
def url(self, /) -> str:
|
||||
return url_for('set.details', id=self.fields.id)
|
||||
|
||||
# Deletion url
|
||||
def url_for_delete(self, /) -> str:
|
||||
return url_for('set.delete', id=self.fields.id)
|
||||
|
||||
# Actual deletion url
|
||||
def url_for_do_delete(self, /) -> str:
|
||||
return url_for('set.do_delete', id=self.fields.id)
|
||||
|
||||
# Compute the url for the set instructions
|
||||
def url_for_instructions(self, /) -> str:
|
||||
if (
|
||||
not current_app.config['HIDE_SET_INSTRUCTIONS'] and
|
||||
len(self.instructions)
|
||||
):
|
||||
return url_for(
|
||||
'set.details',
|
||||
id=self.fields.id,
|
||||
open_instructions=True
|
||||
)
|
||||
else:
|
||||
return ''
|
||||
|
||||
# Compute the url for the refresh button
|
||||
def url_for_refresh(self, /) -> str:
|
||||
return url_for('set.refresh', id=self.fields.id)
|
||||
|
||||
# Compute the url for the set storage
|
||||
def url_for_storage(self, /) -> str:
|
||||
if self.fields.storage is not None:
|
||||
return url_for('storage.details', id=self.fields.storage)
|
||||
else:
|
||||
return ''
|
||||
|
||||
# Update purchase date url
|
||||
def url_for_purchase_date(self, /) -> str:
|
||||
return url_for('set.update_purchase_date', id=self.fields.id)
|
||||
|
||||
# Update purchase price url
|
||||
def url_for_purchase_price(self, /) -> str:
|
||||
return url_for('set.update_purchase_price', id=self.fields.id)
|
@ -1,192 +0,0 @@
|
||||
from typing import Any, Self, Union
|
||||
|
||||
from flask import current_app
|
||||
|
||||
from .record_list import BrickRecordList
|
||||
from .set_owner import BrickSetOwner
|
||||
from .set_owner_list import BrickSetOwnerList
|
||||
from .set_purchase_location import BrickSetPurchaseLocation
|
||||
from .set_purchase_location_list import BrickSetPurchaseLocationList
|
||||
from .set_status_list import BrickSetStatusList
|
||||
from .set_storage import BrickSetStorage
|
||||
from .set_storage_list import BrickSetStorageList
|
||||
from .set_tag import BrickSetTag
|
||||
from .set_tag_list import BrickSetTagList
|
||||
from .set import BrickSet
|
||||
|
||||
|
||||
# All the sets from the database
|
||||
class BrickSetList(BrickRecordList[BrickSet]):
|
||||
themes: list[str]
|
||||
order: str
|
||||
|
||||
# Queries
|
||||
damaged_minifigure_query: str = 'set/list/damaged_minifigure'
|
||||
damaged_part_query: str = 'set/list/damaged_part'
|
||||
generic_query: str = 'set/list/generic'
|
||||
light_query: str = 'set/list/light'
|
||||
missing_minifigure_query: str = 'set/list/missing_minifigure'
|
||||
missing_part_query: str = 'set/list/missing_part'
|
||||
select_query: str = 'set/list/all'
|
||||
using_minifigure_query: str = 'set/list/using_minifigure'
|
||||
using_part_query: str = 'set/list/using_part'
|
||||
using_storage_query: str = 'set/list/using_storage'
|
||||
|
||||
def __init__(self, /):
|
||||
super().__init__()
|
||||
|
||||
# Placeholders
|
||||
self.themes = []
|
||||
|
||||
# Store the order for this list
|
||||
self.order = current_app.config['SETS_DEFAULT_ORDER']
|
||||
|
||||
# All the sets
|
||||
def all(self, /) -> Self:
|
||||
# Load the sets from the database
|
||||
self.list(do_theme=True)
|
||||
|
||||
return self
|
||||
|
||||
# Sets with a minifigure part damaged
|
||||
def damaged_minifigure(self, figure: str, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.figure = figure
|
||||
|
||||
# Load the sets from the database
|
||||
self.list(override_query=self.damaged_minifigure_query)
|
||||
|
||||
return self
|
||||
|
||||
# Sets with a part damaged
|
||||
def damaged_part(self, part: str, color: int, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.part = part
|
||||
self.fields.color = color
|
||||
|
||||
# Load the sets from the database
|
||||
self.list(override_query=self.damaged_part_query)
|
||||
|
||||
return self
|
||||
|
||||
# Last added sets
|
||||
def last(self, /, *, limit: int = 6) -> Self:
|
||||
# Randomize
|
||||
if current_app.config['RANDOM']:
|
||||
order = 'RANDOM()'
|
||||
else:
|
||||
order = '"bricktracker_sets"."rowid" DESC'
|
||||
|
||||
self.list(order=order, limit=limit)
|
||||
|
||||
return self
|
||||
|
||||
# Base set list
|
||||
def list(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
override_query: str | None = None,
|
||||
order: str | None = None,
|
||||
limit: int | None = None,
|
||||
do_theme: bool = False,
|
||||
**context: Any,
|
||||
) -> None:
|
||||
themes = set()
|
||||
|
||||
if order is None:
|
||||
order = self.order
|
||||
|
||||
# Load the sets from the database
|
||||
for record in super().select(
|
||||
override_query=override_query,
|
||||
order=order,
|
||||
limit=limit,
|
||||
owners=BrickSetOwnerList.as_columns(),
|
||||
statuses=BrickSetStatusList.as_columns(),
|
||||
tags=BrickSetTagList.as_columns(),
|
||||
):
|
||||
brickset = BrickSet(record=record)
|
||||
|
||||
self.records.append(brickset)
|
||||
if do_theme:
|
||||
themes.add(brickset.theme.name)
|
||||
|
||||
# Convert the set into a list and sort it
|
||||
if do_theme:
|
||||
self.themes = list(themes)
|
||||
self.themes.sort()
|
||||
|
||||
# Sets missing a minifigure part
|
||||
def missing_minifigure(self, figure: str, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.figure = figure
|
||||
|
||||
# Load the sets from the database
|
||||
self.list(override_query=self.missing_minifigure_query)
|
||||
|
||||
return self
|
||||
|
||||
# Sets missing a part
|
||||
def missing_part(self, part: str, color: int, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.part = part
|
||||
self.fields.color = color
|
||||
|
||||
# Load the sets from the database
|
||||
self.list(override_query=self.missing_part_query)
|
||||
|
||||
return self
|
||||
|
||||
# Sets using a minifigure
|
||||
def using_minifigure(self, figure: str, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.figure = figure
|
||||
|
||||
# Load the sets from the database
|
||||
self.list(override_query=self.using_minifigure_query)
|
||||
|
||||
return self
|
||||
|
||||
# Sets using a part
|
||||
def using_part(self, part: str, color: int, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.part = part
|
||||
self.fields.color = color
|
||||
|
||||
# Load the sets from the database
|
||||
self.list(override_query=self.using_part_query)
|
||||
|
||||
return self
|
||||
|
||||
# Sets using a storage
|
||||
def using_storage(self, storage: BrickSetStorage, /) -> Self:
|
||||
# Save the parameters to the fields
|
||||
self.fields.storage = storage.fields.id
|
||||
|
||||
# Load the sets from the database
|
||||
self.list(override_query=self.using_storage_query)
|
||||
|
||||
return self
|
||||
|
||||
|
||||
# Helper to build the metadata lists
|
||||
def set_metadata_lists(
|
||||
as_class: bool = False
|
||||
) -> dict[
|
||||
str,
|
||||
Union[
|
||||
list[BrickSetOwner],
|
||||
list[BrickSetPurchaseLocation],
|
||||
BrickSetPurchaseLocation,
|
||||
list[BrickSetStorage],
|
||||
BrickSetStorageList,
|
||||
list[BrickSetTag]
|
||||
]
|
||||
]:
|
||||
return {
|
||||
'brickset_owners': BrickSetOwnerList.list(),
|
||||
'brickset_purchase_locations': BrickSetPurchaseLocationList.list(as_class=as_class), # noqa: E501
|
||||
'brickset_storages': BrickSetStorageList.list(as_class=as_class),
|
||||
'brickset_tags': BrickSetTagList.list(),
|
||||
}
|
@ -1,16 +0,0 @@
|
||||
from .metadata import BrickMetadata
|
||||
|
||||
|
||||
# Lego set owner metadata
|
||||
class BrickSetOwner(BrickMetadata):
|
||||
kind: str = 'owner'
|
||||
|
||||
# Set state endpoint
|
||||
set_state_endpoint: str = 'set.update_owner'
|
||||
|
||||
# Queries
|
||||
delete_query: str = 'set/metadata/owner/delete'
|
||||
insert_query: str = 'set/metadata/owner/insert'
|
||||
select_query: str = 'set/metadata/owner/select'
|
||||
update_field_query: str = 'set/metadata/owner/update/field'
|
||||
update_set_state_query: str = 'set/metadata/owner/update/state'
|
@ -1,21 +0,0 @@
|
||||
from typing import Self
|
||||
|
||||
from .metadata_list import BrickMetadataList
|
||||
from .set_owner import BrickSetOwner
|
||||
|
||||
|
||||
# Lego sets owner list
|
||||
class BrickSetOwnerList(BrickMetadataList[BrickSetOwner]):
|
||||
kind: str = 'set owners'
|
||||
|
||||
# Database
|
||||
table: str = 'bricktracker_set_owners'
|
||||
order: str = '"bricktracker_metadata_owners"."name"'
|
||||
|
||||
# Queries
|
||||
select_query = 'set/metadata/owner/list'
|
||||
|
||||
# Instantiate the list with the proper class
|
||||
@classmethod
|
||||
def new(cls, /, *, force: bool = False) -> Self:
|
||||
return cls(BrickSetOwner, force=force)
|
@ -1,13 +0,0 @@
|
||||
from .metadata import BrickMetadata
|
||||
|
||||
|
||||
# Lego set purchase location metadata
|
||||
class BrickSetPurchaseLocation(BrickMetadata):
|
||||
kind: str = 'purchase location'
|
||||
|
||||
# Queries
|
||||
delete_query: str = 'set/metadata/purchase_location/delete'
|
||||
insert_query: str = 'set/metadata/purchase_location/insert'
|
||||
select_query: str = 'set/metadata/purchase_location/select'
|
||||
update_field_query: str = 'set/metadata/purchase_location/update/field'
|
||||
update_set_value_query: str = 'set/metadata/purchase_location/update/value'
|
@ -1,42 +0,0 @@
|
||||
from typing import Self
|
||||
|
||||
from flask import current_app
|
||||
|
||||
from .metadata_list import BrickMetadataList
|
||||
from .set_purchase_location import BrickSetPurchaseLocation
|
||||
|
||||
|
||||
# Lego sets purchase location list
|
||||
class BrickSetPurchaseLocationList(
|
||||
BrickMetadataList[BrickSetPurchaseLocation]
|
||||
):
|
||||
kind: str = 'set purchase locations'
|
||||
|
||||
# Order
|
||||
order: str = '"bricktracker_metadata_purchase_locations"."name"'
|
||||
|
||||
# Queries
|
||||
select_query: str = 'set/metadata/purchase_location/list'
|
||||
all_query: str = 'set/metadata/purchase_location/all'
|
||||
|
||||
# Set value endpoint
|
||||
set_value_endpoint: str = 'set.update_purchase_location'
|
||||
|
||||
# Load all purchase locations
|
||||
@classmethod
|
||||
def all(cls, /) -> Self:
|
||||
new = cls.new()
|
||||
new.override()
|
||||
|
||||
for record in new.select(
|
||||
override_query=cls.all_query,
|
||||
order=current_app.config['PURCHASE_LOCATION_DEFAULT_ORDER']
|
||||
):
|
||||
new.records.append(new.model(record=record))
|
||||
|
||||
return new
|
||||
|
||||
# Instantiate the list with the proper class
|
||||
@classmethod
|
||||
def new(cls, /, *, force: bool = False) -> Self:
|
||||
return cls(BrickSetPurchaseLocation, force=force)
|
@ -1,34 +0,0 @@
|
||||
from typing import Self
|
||||
|
||||
from .metadata import BrickMetadata
|
||||
|
||||
|
||||
# Lego set status metadata
|
||||
class BrickSetStatus(BrickMetadata):
|
||||
kind: str = 'status'
|
||||
|
||||
# Set state endpoint
|
||||
set_state_endpoint: str = 'set.update_status'
|
||||
|
||||
# Queries
|
||||
delete_query: str = 'set/metadata/status/delete'
|
||||
insert_query: str = 'set/metadata/status/insert'
|
||||
select_query: str = 'set/metadata/status/select'
|
||||
update_field_query: str = 'set/metadata/status/update/field'
|
||||
update_set_state_query: str = 'set/metadata/status/update/state'
|
||||
|
||||
# Grab data from a form
|
||||
def from_form(self, form: dict[str, str], /) -> Self:
|
||||
super().from_form(form)
|
||||
|
||||
grid = form.get('grid', None)
|
||||
|
||||
self.fields.displayed_on_grid = grid == 'on'
|
||||
|
||||
return self
|
||||
|
||||
# Insert into database
|
||||
def insert(self, /, **_) -> None:
|
||||
super().insert(
|
||||
displayed_on_grid=self.fields.displayed_on_grid
|
||||
)
|
@ -1,30 +0,0 @@
|
||||
from typing import Self
|
||||
|
||||
from .metadata_list import BrickMetadataList
|
||||
from .set_status import BrickSetStatus
|
||||
|
||||
|
||||
# Lego sets status list
|
||||
class BrickSetStatusList(BrickMetadataList[BrickSetStatus]):
|
||||
kind: str = 'set statuses'
|
||||
|
||||
# Database
|
||||
table: str = 'bricktracker_set_statuses'
|
||||
order: str = '"bricktracker_metadata_statuses"."name"'
|
||||
|
||||
# Queries
|
||||
select_query = 'set/metadata/status/list'
|
||||
|
||||
# Filter the list of set status
|
||||
def filter(self, all: bool = False) -> list[BrickSetStatus]:
|
||||
return [
|
||||
record
|
||||
for record
|
||||
in self.records
|
||||
if all or record.fields.displayed_on_grid
|
||||
]
|
||||
|
||||
# Instantiate the list with the proper class
|
||||
@classmethod
|
||||
def new(cls, /, *, force: bool = False) -> Self:
|
||||
return cls(BrickSetStatus, force=force)
|
@ -1,22 +0,0 @@
|
||||
from .metadata import BrickMetadata
|
||||
|
||||
from flask import url_for
|
||||
|
||||
|
||||
# Lego set storage metadata
|
||||
class BrickSetStorage(BrickMetadata):
|
||||
kind: str = 'storage'
|
||||
|
||||
# Queries
|
||||
delete_query: str = 'set/metadata/storage/delete'
|
||||
insert_query: str = 'set/metadata/storage/insert'
|
||||
select_query: str = 'set/metadata/storage/select'
|
||||
update_field_query: str = 'set/metadata/storage/update/field'
|
||||
update_set_value_query: str = 'set/metadata/storage/update/value'
|
||||
|
||||
# Self url
|
||||
def url(self, /) -> str:
|
||||
return url_for(
|
||||
'storage.details',
|
||||
id=self.fields.id,
|
||||
)
|
@ -1,40 +0,0 @@
|
||||
from typing import Self
|
||||
|
||||
from flask import current_app
|
||||
|
||||
from .metadata_list import BrickMetadataList
|
||||
from .set_storage import BrickSetStorage
|
||||
|
||||
|
||||
# Lego sets storage list
|
||||
class BrickSetStorageList(BrickMetadataList[BrickSetStorage]):
|
||||
kind: str = 'set storages'
|
||||
|
||||
# Order
|
||||
order: str = '"bricktracker_metadata_storages"."name"'
|
||||
|
||||
# Queries
|
||||
select_query: str = 'set/metadata/storage/list'
|
||||
all_query: str = 'set/metadata/storage/all'
|
||||
|
||||
# Set value endpoint
|
||||
set_value_endpoint: str = 'set.update_storage'
|
||||
|
||||
# Load all storages
|
||||
@classmethod
|
||||
def all(cls, /) -> Self:
|
||||
new = cls.new()
|
||||
new.override()
|
||||
|
||||
for record in new.select(
|
||||
override_query=cls.all_query,
|
||||
order=current_app.config['STORAGE_DEFAULT_ORDER']
|
||||
):
|
||||
new.records.append(new.model(record=record))
|
||||
|
||||
return new
|
||||
|
||||
# Instantiate the list with the proper class
|
||||
@classmethod
|
||||
def new(cls, /, *, force: bool = False) -> Self:
|
||||
return cls(BrickSetStorage, force=force)
|
@ -1,16 +0,0 @@
|
||||
from .metadata import BrickMetadata
|
||||
|
||||
|
||||
# Lego set tag metadata
|
||||
class BrickSetTag(BrickMetadata):
|
||||
kind: str = 'tag'
|
||||
|
||||
# Set state endpoint
|
||||
set_state_endpoint: str = 'set.update_tag'
|
||||
|
||||
# Queries
|
||||
delete_query: str = 'set/metadata/tag/delete'
|
||||
insert_query: str = 'set/metadata/tag/insert'
|
||||
select_query: str = 'set/metadata/tag/select'
|
||||
update_field_query: str = 'set/metadata/tag/update/field'
|
||||
update_set_state_query: str = 'set/metadata/tag/update/state'
|
@ -1,21 +0,0 @@
|
||||
from typing import Self
|
||||
|
||||
from .metadata_list import BrickMetadataList
|
||||
from .set_tag import BrickSetTag
|
||||
|
||||
|
||||
# Lego sets tag list
|
||||
class BrickSetTagList(BrickMetadataList[BrickSetTag]):
|
||||
kind: str = 'set tags'
|
||||
|
||||
# Database
|
||||
table: str = 'bricktracker_set_tags'
|
||||
order: str = '"bricktracker_metadata_tags"."name"'
|
||||
|
||||
# Queries
|
||||
select_query: str = 'set/metadata/tag/list'
|
||||
|
||||
# Instantiate the list with the proper class
|
||||
@classmethod
|
||||
def new(cls, /, *, force: bool = False) -> Self:
|
||||
return cls(BrickSetTag, force=force)
|
@ -1,221 +0,0 @@
|
||||
import logging
|
||||
from typing import Any, Final, Tuple
|
||||
|
||||
from flask import Flask, request
|
||||
from flask_socketio import SocketIO
|
||||
|
||||
from .instructions import BrickInstructions
|
||||
from .instructions_list import BrickInstructionsList
|
||||
from .set import BrickSet
|
||||
from .socket_decorator import authenticated_socket, rebrickable_socket
|
||||
from .sql import close as sql_close
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
# Messages valid through the socket
|
||||
MESSAGES: Final[dict[str, str]] = {
|
||||
'COMPLETE': 'complete',
|
||||
'CONNECT': 'connect',
|
||||
'DISCONNECT': 'disconnect',
|
||||
'DOWNLOAD_INSTRUCTIONS': 'download_instructions',
|
||||
'FAIL': 'fail',
|
||||
'IMPORT_SET': 'import_set',
|
||||
'LOAD_SET': 'load_set',
|
||||
'PROGRESS': 'progress',
|
||||
'SET_LOADED': 'set_loaded',
|
||||
}
|
||||
|
||||
|
||||
# Flask socket.io with our extra features
|
||||
class BrickSocket(object):
|
||||
app: Flask
|
||||
socket: SocketIO
|
||||
threaded: bool
|
||||
|
||||
# Progress
|
||||
progress_message: str
|
||||
progress_total: int
|
||||
progress_count: int
|
||||
|
||||
def __init__(
|
||||
self,
|
||||
app: Flask,
|
||||
*args,
|
||||
threaded: bool = True,
|
||||
**kwargs
|
||||
):
|
||||
# Save the app
|
||||
self.app = app
|
||||
|
||||
# Progress
|
||||
self.progress_message = ''
|
||||
self.progress_count = 0
|
||||
self.progress_total = 0
|
||||
|
||||
# Save the threaded flag
|
||||
self.threaded = threaded
|
||||
|
||||
# Compute the namespace
|
||||
self.namespace = '/{namespace}'.format(
|
||||
namespace=app.config['SOCKET_NAMESPACE']
|
||||
)
|
||||
|
||||
# Inject CORS if a domain is defined
|
||||
if app.config['DOMAIN_NAME'] != '':
|
||||
kwargs['cors_allowed_origins'] = app.config['DOMAIN_NAME']
|
||||
|
||||
# Instantiate the socket
|
||||
self.socket = SocketIO(
|
||||
self.app,
|
||||
*args,
|
||||
**kwargs,
|
||||
path=app.config['SOCKET_PATH'],
|
||||
async_mode='eventlet',
|
||||
)
|
||||
|
||||
# Store the socket in the app config
|
||||
self.app.config['_SOCKET'] = self
|
||||
|
||||
# Setup the socket
|
||||
@self.socket.on(MESSAGES['CONNECT'], namespace=self.namespace)
|
||||
def connect() -> None:
|
||||
self.connected()
|
||||
|
||||
@self.socket.on(MESSAGES['DISCONNECT'], namespace=self.namespace)
|
||||
def disconnect() -> None:
|
||||
self.disconnected()
|
||||
|
||||
@self.socket.on(MESSAGES['DOWNLOAD_INSTRUCTIONS'], namespace=self.namespace) # noqa: E501
|
||||
@authenticated_socket(self)
|
||||
def download_instructions(data: dict[str, Any], /) -> None:
|
||||
instructions = BrickInstructions(
|
||||
'{name}.pdf'.format(name=data.get('alt', '')),
|
||||
socket=self
|
||||
)
|
||||
|
||||
path = data.get('href', '').removeprefix('/instructions/')
|
||||
|
||||
# Update the progress
|
||||
try:
|
||||
self.progress_total = int(data.get('total', 0))
|
||||
self.progress_count = int(data.get('current', 0))
|
||||
except Exception:
|
||||
pass
|
||||
|
||||
instructions.download(path)
|
||||
|
||||
BrickInstructionsList(force=True)
|
||||
|
||||
@self.socket.on(MESSAGES['IMPORT_SET'], namespace=self.namespace)
|
||||
@rebrickable_socket(self)
|
||||
def import_set(data: dict[str, Any], /) -> None:
|
||||
logger.debug('Socket: IMPORT_SET={data} (from: {fr})'.format(
|
||||
data=data,
|
||||
fr=request.sid, # type: ignore
|
||||
))
|
||||
|
||||
BrickSet().download(self, data)
|
||||
|
||||
@self.socket.on(MESSAGES['LOAD_SET'], namespace=self.namespace)
|
||||
def load_set(data: dict[str, Any], /) -> None:
|
||||
logger.debug('Socket: LOAD_SET={data} (from: {fr})'.format(
|
||||
data=data,
|
||||
fr=request.sid, # type: ignore
|
||||
))
|
||||
|
||||
BrickSet().load(self, data)
|
||||
|
||||
# Update the progress auto-incrementing
|
||||
def auto_progress(
|
||||
self,
|
||||
/,
|
||||
*,
|
||||
message: str | None = None,
|
||||
increment_total=False,
|
||||
) -> None:
|
||||
# Auto-increment
|
||||
self.progress_count += 1
|
||||
|
||||
if increment_total:
|
||||
self.progress_total += 1
|
||||
|
||||
self.progress(message=message)
|
||||
|
||||
# Send a complete
|
||||
def complete(self, /, **data: Any) -> None:
|
||||
self.emit('COMPLETE', data)
|
||||
|
||||
# Close any dangling connection
|
||||
sql_close()
|
||||
|
||||
# Socket is connected
|
||||
def connected(self, /) -> Tuple[str, int]:
|
||||
logger.debug('Socket: client connected')
|
||||
|
||||
return '', 301
|
||||
|
||||
# Socket is disconnected
|
||||
def disconnected(self, /) -> None:
|
||||
logger.debug('Socket: client disconnected')
|
||||
|
||||
# Emit a message through the socket
|
||||
def emit(self, name: str, *arg, all=False) -> None:
|
||||
# Emit to all sockets
|
||||
if all:
|
||||
to = None
|
||||
else:
|
||||
# Grab the request SID
|
||||
# This keeps message isolated between clients (and tabs!)
|
||||
try:
|
||||
to = request.sid # type: ignore
|
||||
except Exception:
|
||||
logger.debug('Unable to load request.sid')
|
||||
to = None
|
||||
|
||||
logger.debug('Socket: {name}={args} (to: {to})'.format(
|
||||
name=name,
|
||||
args=arg,
|
||||
to=to,
|
||||
))
|
||||
|
||||
self.socket.emit(
|
||||
MESSAGES[name],
|
||||
*arg,
|
||||
namespace=self.namespace,
|
||||
to=to,
|
||||
)
|
||||
|
||||
# Send a failed
|
||||
def fail(self, /, **data: Any) -> None:
|
||||
self.emit('FAIL', data)
|
||||
|
||||
# Close any dangling connection
|
||||
sql_close()
|
||||
|
||||
# Update the progress
|
||||
def progress(self, /, *, message: str | None = None) -> None:
|
||||
# Save the las message
|
||||
if message is not None:
|
||||
self.progress_message = message
|
||||
|
||||
# Prepare data
|
||||
data: dict[str, Any] = {
|
||||
'message': self.progress_message,
|
||||
'count': self.progress_count,
|
||||
'total': self.progress_total,
|
||||
}
|
||||
|
||||
self.emit('PROGRESS', data)
|
||||
|
||||
# Update the progress total only
|
||||
def update_total(self, total: int, /, *, add: bool = False) -> None:
|
||||
if add:
|
||||
self.progress_total += total
|
||||
else:
|
||||
self.progress_total = total
|
||||
|
||||
# Update the total
|
||||
def total_progress(self, total: int, /, *, add: bool = False) -> None:
|
||||
self.update_total(total, add=add)
|
||||
|
||||
self.progress()
|
@ -1,93 +0,0 @@
|
||||
from functools import wraps
|
||||
from threading import Thread
|
||||
from typing import Callable, ParamSpec, TYPE_CHECKING, Union
|
||||
|
||||
from flask import copy_current_request_context
|
||||
|
||||
from .configuration_list import BrickConfigurationList
|
||||
from .login import LoginManager
|
||||
if TYPE_CHECKING:
|
||||
from .socket import BrickSocket
|
||||
|
||||
# What a threaded function can return (None or Thread)
|
||||
SocketReturn = Union[None, Thread]
|
||||
|
||||
# Threaded signature (*arg, **kwargs -> (None or Thread)
|
||||
P = ParamSpec('P')
|
||||
SocketCallable = Callable[P, SocketReturn]
|
||||
|
||||
|
||||
# Fail if not authenticated
|
||||
def authenticated_socket(
|
||||
self: 'BrickSocket',
|
||||
/,
|
||||
*,
|
||||
threaded: bool = True,
|
||||
) -> Callable[[SocketCallable], SocketCallable]:
|
||||
def outer(function: SocketCallable, /) -> SocketCallable:
|
||||
@wraps(function)
|
||||
def wrapper(*args, **kwargs) -> SocketReturn:
|
||||
# Needs to be authenticated
|
||||
if LoginManager.is_not_authenticated():
|
||||
self.fail(message='You need to be authenticated')
|
||||
return
|
||||
|
||||
# Apply threading
|
||||
if threaded:
|
||||
return threaded_socket(self)(function)(*args, **kwargs)
|
||||
else:
|
||||
return function(*args, **kwargs)
|
||||
|
||||
return wrapper
|
||||
return outer
|
||||
|
||||
|
||||
# Fail if not ready for Rebrickable (authenticated, API key)
|
||||
# Automatically makes it threaded
|
||||
def rebrickable_socket(
|
||||
self: 'BrickSocket',
|
||||
/,
|
||||
*,
|
||||
threaded: bool = True,
|
||||
) -> Callable[[SocketCallable], SocketCallable]:
|
||||
def outer(function: SocketCallable, /) -> SocketCallable:
|
||||
@wraps(function)
|
||||
# Automatically authenticated
|
||||
@authenticated_socket(self, threaded=False)
|
||||
def wrapper(*args, **kwargs) -> SocketReturn:
|
||||
# Needs the Rebrickable API key
|
||||
try:
|
||||
BrickConfigurationList.error_unless_is_set('REBRICKABLE_API_KEY') # noqa: E501
|
||||
except Exception as e:
|
||||
self.fail(message=str(e))
|
||||
return
|
||||
|
||||
# Apply threading
|
||||
if threaded:
|
||||
return threaded_socket(self)(function)(*args, **kwargs)
|
||||
else:
|
||||
return function(*args, **kwargs)
|
||||
|
||||
return wrapper
|
||||
return outer
|
||||
|
||||
|
||||
# Start the function in a thread if the socket is threaded
|
||||
def threaded_socket(
|
||||
self: 'BrickSocket',
|
||||
/
|
||||
) -> Callable[[SocketCallable], SocketCallable]:
|
||||
def outer(function: SocketCallable, /) -> SocketCallable:
|
||||
@wraps(function)
|
||||
def wrapper(*args, **kwargs) -> SocketReturn:
|
||||
# Start it in a thread if requested
|
||||
if self.threaded:
|
||||
@copy_current_request_context
|
||||
def do_function() -> None:
|
||||
function(*args, **kwargs)
|
||||
|
||||
return self.socket.start_background_task(do_function)
|
||||
else:
|
||||
return function(*args, **kwargs)
|
||||
return wrapper
|
||||
return outer
|
@ -1,399 +0,0 @@
|
||||
from importlib import import_module
|
||||
import logging
|
||||
import os
|
||||
import sqlite3
|
||||
from typing import Any, Final, Tuple
|
||||
|
||||
from flask import current_app, g
|
||||
from jinja2 import Environment, FileSystemLoader
|
||||
from werkzeug.datastructures import FileStorage
|
||||
|
||||
from .exceptions import DatabaseException
|
||||
from .sql_counter import BrickCounter
|
||||
from .sql_migration_list import BrickSQLMigrationList
|
||||
from .sql_stats import BrickSQLStats
|
||||
from .version import __database_version__
|
||||
|
||||
logger = logging.getLogger(__name__)
|
||||
|
||||
G_CONNECTION: Final[str] = 'database_connection'
|
||||
G_ENVIRONMENT: Final[str] = 'database_environment'
|
||||
G_DEFER: Final[str] = 'database_defer'
|
||||
G_STATS: Final[str] = 'database_stats'
|
||||
|
||||
|
||||
# SQLite3 client with our extra features
|
||||
class BrickSQL(object):
|
||||
connection: sqlite3.Connection
|
||||
cursor: sqlite3.Cursor
|
||||
stats: BrickSQLStats
|
||||
version: int
|
||||
|
||||
def __init__(self, /, *, failsafe: bool = False):
|
||||
# Instantiate the database connection in the Flask
|
||||
# application context so that it can be used by all
|
||||
# requests without re-opening connections
|
||||
connection = getattr(g, G_CONNECTION, None)
|
||||
|
||||
# Grab the existing connection if it exists
|
||||
if connection is not None:
|
||||
self.connection = connection
|
||||
self.stats = getattr(g, G_STATS, BrickSQLStats())
|
||||
|
||||
# Grab a cursor
|
||||
self.cursor = self.connection.cursor()
|
||||
else:
|
||||
# Instantiate the stats
|
||||
self.stats = BrickSQLStats()
|
||||
|
||||
# Stats: connect
|
||||
self.stats.connect += 1
|
||||
|
||||
logger.debug('SQLite3: connect')
|
||||
self.connection = sqlite3.connect(
|
||||
current_app.config['DATABASE_PATH']
|
||||
)
|
||||
|
||||
# Setup the row factory to get pseudo-dicts rather than tuples
|
||||
self.connection.row_factory = sqlite3.Row
|
||||
|
||||
# Grab a cursor
|
||||
self.cursor = self.connection.cursor()
|
||||
|
||||
# Grab the version and check
|
||||
try:
|
||||
version = self.fetchone('schema/get_version')
|
||||
|
||||
if version is None:
|
||||
raise Exception('version is None')
|
||||
|
||||
self.version = version[0]
|
||||
except Exception as e:
|
||||
self.version = 0
|
||||
|
||||
raise DatabaseException('Could not get the database version: {error}'.format( # noqa: E501
|
||||
error=str(e)
|
||||
))
|
||||
|
||||
if self.upgrade_too_far():
|
||||
raise DatabaseException('Your database version ({version}) is too far ahead for this version of the application. Expected at most {required}'.format( # noqa: E501
|
||||
version=self.version,
|
||||
required=__database_version__,
|
||||
))
|
||||
|
||||
# Debug: Attach the debugger
|
||||
# Uncomment manually because this is ultra verbose
|
||||
# self.connection.set_trace_callback(print)
|
||||
|
||||
# Save the connection globally for later use
|
||||
setattr(g, G_CONNECTION, self.connection)
|
||||
setattr(g, G_STATS, self.stats)
|
||||
|
||||
if not failsafe:
|
||||
if self.upgrade_needed():
|
||||
raise DatabaseException('Your database need to be upgraded from version {version} to version {required}'.format( # noqa: E501
|
||||
version=self.version,
|
||||
required=__database_version__,
|
||||
))
|
||||
|
||||
# Clear the defer stack
|
||||
def clear_defer(self, /) -> None:
|
||||
setattr(g, G_DEFER, [])
|
||||
|
||||
# Shorthand to commit
|
||||
def commit(self, /) -> None:
|
||||
# Stats: commit
|
||||
self.stats.commit += 1
|
||||
|
||||
# Process the defered stack
|
||||
for item in self.get_defer():
|
||||
self.raw_execute(item[0], item[1])
|
||||
|
||||
self.clear_defer()
|
||||
|
||||
logger.debug('SQLite3: commit')
|
||||
return self.connection.commit()
|
||||
|
||||
# Count the database records
|
||||
def count_records(self) -> list[BrickCounter]:
|
||||
counters: list[BrickCounter] = []
|
||||
|
||||
# Get all tables
|
||||
for table in self.fetchall('schema/tables'):
|
||||
counter = BrickCounter(table['name'])
|
||||
|
||||
# Failsafe this one
|
||||
try:
|
||||
record = self.fetchone('schema/count', table=counter.table)
|
||||
|
||||
if record is not None:
|
||||
counter.count = record['count']
|
||||
except Exception:
|
||||
pass
|
||||
|
||||
counters.append(counter)
|
||||
|
||||
return counters
|
||||
|
||||
# Defer a call to execute
|
||||
def defer(self, query: str, parameters: dict[str, Any], /):
|
||||
defer = self.get_defer()
|
||||
|
||||
logger.debug('SQLite3: defer execute')
|
||||
|
||||
# Add the query and parameters to the defer stack
|
||||
defer.append((query, parameters))
|
||||
|
||||
# Save the defer stack
|
||||
setattr(g, G_DEFER, defer)
|
||||
|
||||
# Shorthand to execute, returning number of affected rows
|
||||
def execute(
|
||||
self,
|
||||
query: str,
|
||||
/,
|
||||
*,
|
||||
parameters: dict[str, Any] = {},
|
||||
defer: bool = False,
|
||||
**context: Any,
|
||||
) -> Tuple[int, str]:
|
||||
# Stats: execute
|
||||
self.stats.execute += 1
|
||||
|
||||
# Load the query
|
||||
query = self.load_query(query, **context)
|
||||
|
||||
# Defer
|
||||
if defer:
|
||||
self.defer(query, parameters)
|
||||
|
||||
return -1, query
|
||||
else:
|
||||
result = self.raw_execute(query, parameters)
|
||||
|
||||
# Stats: changed
|
||||
if result.rowcount > 0:
|
||||
self.stats.changed += result.rowcount
|
||||
|
||||
return result.rowcount, query
|
||||
|
||||
# Shorthand to executescript
|
||||
def executescript(self, query: str, /, **context: Any) -> None:
|
||||
# Load the query
|
||||
query = self.load_query(query, **context)
|
||||
|
||||
# Stats: executescript
|
||||
self.stats.executescript += 1
|
||||
|
||||
logger.debug('SQLite3: executescript')
|
||||
self.cursor.executescript(query)
|
||||
|
||||
# Shorthand to execute and commit
|
||||
def execute_and_commit(
|
||||
self,
|
||||
query: str,
|
||||
/,
|
||||
*,
|
||||
parameters: dict[str, Any] = {},
|
||||
**context: Any,
|
||||
) -> Tuple[int, str]:
|
||||
rows, query = self.execute(query, parameters=parameters, **context)
|
||||
self.commit()
|
||||
|
||||
return rows, query
|
||||
|
||||
# Shorthand to execute and fetchall
|
||||
def fetchall(
|
||||
self,
|
||||
query: str,
|
||||
/,
|
||||
*,
|
||||
parameters: dict[str, Any] = {},
|
||||
**context: Any,
|
||||
) -> list[sqlite3.Row]:
|
||||
_, query = self.execute(query, parameters=parameters, **context)
|
||||
|
||||
# Stats: fetchall
|
||||
self.stats.fetchall += 1
|
||||
|
||||
logger.debug('SQLite3: fetchall')
|
||||
records = self.cursor.fetchall()
|
||||
|
||||
# Stats: fetched
|
||||
self.stats.fetched += len(records)
|
||||
|
||||
return records
|
||||
|
||||
# Shorthand to execute and fetchone
|
||||
def fetchone(
|
||||
self,
|
||||
query: str,
|
||||
/,
|
||||
*,
|
||||
parameters: dict[str, Any] = {},
|
||||
**context: Any,
|
||||
) -> sqlite3.Row | None:
|
||||
_, query = self.execute(query, parameters=parameters, **context)
|
||||
|
||||
# Stats: fetchone
|
||||
self.stats.fetchone += 1
|
||||
|
||||
logger.debug('SQLite3: fetchone')
|
||||
record = self.cursor.fetchone()
|
||||
|
||||
# Stats: fetched
|
||||
if record is not None:
|
||||
self.stats.fetched += len(record)
|
||||
|
||||
return record
|
||||
|
||||
# Grab the defer stack
|
||||
def get_defer(self, /) -> list[Tuple[str, dict[str, Any]]]:
|
||||
defer: list[Tuple[str, dict[str, Any]]] = getattr(g, G_DEFER, [])
|
||||
|
||||
return defer
|
||||
|
||||
# Load a query by name
|
||||
def load_query(self, name: str, /, **context: Any) -> str:
|
||||
# Grab the existing environment if it exists
|
||||
environment = getattr(g, G_ENVIRONMENT, None)
|
||||
|
||||
# Instantiate Jinja environment for SQL files
|
||||
if environment is None:
|
||||
logger.debug('SQLite3: instantiating the Jinja loader')
|
||||
environment = Environment(
|
||||
loader=FileSystemLoader(
|
||||
os.path.join(os.path.dirname(__file__), 'sql/')
|
||||
)
|
||||
)
|
||||
|
||||
# Save the environment globally for later use
|
||||
setattr(g, G_ENVIRONMENT, environment)
|
||||
|
||||
# Grab the template
|
||||
logger.debug('SQLite3: loading {name} (context: {context})'.format(
|
||||
name=name,
|
||||
context=context,
|
||||
))
|
||||
template = environment.get_template('{name}.sql'.format(
|
||||
name=name,
|
||||
))
|
||||
|
||||
return template.render(**context)
|
||||
|
||||
# Raw execute the query without any options
|
||||
def raw_execute(
|
||||
self,
|
||||
query: str,
|
||||
parameters: dict[str, Any],
|
||||
/
|
||||
) -> sqlite3.Cursor:
|
||||
logger.debug('SQLite3: execute: {query}'.format(
|
||||
query=BrickSQL.clean_query(query)
|
||||
))
|
||||
|
||||
return self.cursor.execute(query, parameters)
|
||||
|
||||
# Upgrade the database
|
||||
def upgrade(self) -> None:
|
||||
if self.upgrade_needed():
|
||||
for pending in BrickSQLMigrationList().pending(self.version):
|
||||
logger.info('Applying migration {version}'.format(
|
||||
version=pending.version)
|
||||
)
|
||||
|
||||
# Load context from the migrations if it exists
|
||||
# It looks for a file in migrations/ named after the SQL file
|
||||
# and containing one function named migration_xxxx, also named
|
||||
# after the SQL file, returning a context dict.
|
||||
#
|
||||
# For instance:
|
||||
# - sql/migrations/0007.sql
|
||||
# - migrations/0007.py
|
||||
# - def migration_0007(BrickSQL) -> dict[str, Any]
|
||||
try:
|
||||
module = import_module(
|
||||
'.migrations.{name}'.format(
|
||||
name=pending.name
|
||||
),
|
||||
package='bricktracker'
|
||||
)
|
||||
except Exception:
|
||||
module = None
|
||||
|
||||
# If a module has been loaded, we need to fail if an error
|
||||
# occured while executing the migration function
|
||||
if module is not None:
|
||||
function = getattr(module, 'migration_{name}'.format(
|
||||
name=pending.name
|
||||
))
|
||||
|
||||
context: dict[str, Any] = function(self)
|
||||
else:
|
||||
context: dict[str, Any] = {}
|
||||
|
||||
self.executescript(pending.get_query(), **context)
|
||||
self.execute('schema/set_version', version=pending.version)
|
||||
|
||||
# Tells whether the database needs upgrade
|
||||
def upgrade_needed(self) -> bool:
|
||||
return self.version < __database_version__
|
||||
|
||||
# Tells whether the database is too far
|
||||
def upgrade_too_far(self) -> bool:
|
||||
return self.version > __database_version__
|
||||
|
||||
# Clean the query for debugging
|
||||
@staticmethod
|
||||
def clean_query(query: str, /) -> str:
|
||||
cleaned: list[str] = []
|
||||
|
||||
for line in query.splitlines():
|
||||
# Keep the non-comment side
|
||||
line, sep, comment = line.partition('--')
|
||||
|
||||
# Clean the non-comment side
|
||||
line = line.strip()
|
||||
|
||||
if line:
|
||||
cleaned.append(line)
|
||||
|
||||
return ' '.join(cleaned)
|
||||
|
||||
# Delete the database
|
||||
@staticmethod
|
||||
def delete() -> None:
|
||||
os.remove(current_app.config['DATABASE_PATH'])
|
||||
|
||||
# Info
|
||||
logger.info('The database has been deleted')
|
||||
|
||||
# Drop the database
|
||||
@staticmethod
|
||||
def drop() -> None:
|
||||
BrickSQL().executescript('schema/drop')
|
||||
|
||||
# Info
|
||||
logger.info('The database has been dropped')
|
||||
|
||||
# Replace the database with a new file
|
||||
@staticmethod
|
||||
def upload(file: FileStorage, /) -> None:
|
||||
file.save(current_app.config['DATABASE_PATH'])
|
||||
|
||||
# Info
|
||||
logger.info('The database has been imported using file {file}'.format(
|
||||
file=file.filename
|
||||
))
|
||||
|
||||
|
||||
# Close all existing SQLite3 connections
|
||||
def close() -> None:
|
||||
connection: sqlite3.Connection | None = getattr(g, G_CONNECTION, None)
|
||||
|
||||
if connection is not None:
|
||||
logger.debug('SQLite3: close')
|
||||
connection.close()
|
||||
|
||||
# Remove the database from the context
|
||||
delattr(g, G_CONNECTION)
|
@ -1,66 +0,0 @@
|
||||
-- description: Original database initialization
|
||||
-- FROM sqlite3 app.db .schema > init.sql with extra IF NOT EXISTS, transaction and quotes
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
CREATE TABLE IF NOT EXISTS "wishlist" (
|
||||
"set_num" TEXT,
|
||||
"name" TEXT,
|
||||
"year" INTEGER,
|
||||
"theme_id" INTEGER,
|
||||
"num_parts" INTEGER,
|
||||
"set_img_url" TEXT,
|
||||
"set_url" TEXT,
|
||||
"last_modified_dt" TEXT
|
||||
);
|
||||
|
||||
CREATE TABLE IF NOT EXISTS "sets" (
|
||||
"set_num" TEXT,
|
||||
"name" TEXT,
|
||||
"year" INTEGER,
|
||||
"theme_id" INTEGER,
|
||||
"num_parts" INTEGER,
|
||||
"set_img_url" TEXT,
|
||||
"set_url" TEXT,
|
||||
"last_modified_dt" TEXT,
|
||||
"mini_col" BOOLEAN,
|
||||
"set_check" BOOLEAN,
|
||||
"set_col" BOOLEAN,
|
||||
"u_id" TEXT
|
||||
);
|
||||
|
||||
CREATE TABLE IF NOT EXISTS "inventory" (
|
||||
"set_num" TEXT,
|
||||
"id" INTEGER,
|
||||
"part_num" TEXT,
|
||||
"name" TEXT,
|
||||
"part_img_url" TEXT,
|
||||
"part_img_url_id" TEXT,
|
||||
"color_id" INTEGER,
|
||||
"color_name" TEXT,
|
||||
"quantity" INTEGER,
|
||||
"is_spare" BOOLEAN,
|
||||
"element_id" INTEGER,
|
||||
"u_id" TEXT
|
||||
);
|
||||
|
||||
CREATE TABLE IF NOT EXISTS "minifigures" (
|
||||
"fig_num" TEXT,
|
||||
"set_num" TEXT,
|
||||
"name" TEXT,
|
||||
"quantity" INTEGER,
|
||||
"set_img_url" TEXT,
|
||||
"u_id" TEXT
|
||||
);
|
||||
|
||||
CREATE TABLE IF NOT EXISTS "missing" (
|
||||
"set_num" TEXT,
|
||||
"id" INTEGER,
|
||||
"part_num" TEXT,
|
||||
"part_img_url_id" TEXT,
|
||||
"color_id" INTEGER,
|
||||
"quantity" INTEGER,
|
||||
"element_id" INTEGER,
|
||||
"u_id" TEXT
|
||||
);
|
||||
|
||||
COMMIT;
|
@ -1,13 +0,0 @@
|
||||
-- description: WAL journal, 'None' fix for missing table
|
||||
|
||||
-- Set the journal mode to WAL
|
||||
PRAGMA journal_mode = WAL;
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Fix a bug where 'None' was inserted in missing instead of NULL
|
||||
UPDATE "missing"
|
||||
SET "element_id" = NULL
|
||||
WHERE "missing"."element_id" = 'None';
|
||||
|
||||
COMMIT;
|
@ -1,48 +0,0 @@
|
||||
-- description: Creation of the deduplicated table of Rebrickable sets
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Create a Rebrickable set table: each unique set imported from Rebrickable
|
||||
CREATE TABLE "rebrickable_sets" (
|
||||
"set" TEXT NOT NULL,
|
||||
"number" INTEGER NOT NULL,
|
||||
"version" INTEGER NOT NULL,
|
||||
"name" TEXT NOT NULL,
|
||||
"year" INTEGER NOT NULL,
|
||||
"theme_id" INTEGER NOT NULL,
|
||||
"number_of_parts" INTEGER NOT NULL,
|
||||
"image" TEXT,
|
||||
"url" TEXT,
|
||||
"last_modified" TEXT,
|
||||
PRIMARY KEY("set")
|
||||
);
|
||||
|
||||
-- Insert existing sets into the new table
|
||||
INSERT INTO "rebrickable_sets" (
|
||||
"set",
|
||||
"number",
|
||||
"version",
|
||||
"name",
|
||||
"year",
|
||||
"theme_id",
|
||||
"number_of_parts",
|
||||
"image",
|
||||
"url",
|
||||
"last_modified"
|
||||
)
|
||||
SELECT
|
||||
"sets"."set_num",
|
||||
CAST(SUBSTR("sets"."set_num", 1, INSTR("sets"."set_num", '-') - 1) AS INTEGER),
|
||||
CAST(SUBSTR("sets"."set_num", INSTR("sets"."set_num", '-') + 1) AS INTEGER),
|
||||
"sets"."name",
|
||||
"sets"."year",
|
||||
"sets"."theme_id",
|
||||
"sets"."num_parts",
|
||||
"sets"."set_img_url",
|
||||
"sets"."set_url",
|
||||
"sets"."last_modified_dt"
|
||||
FROM "sets"
|
||||
GROUP BY
|
||||
"sets"."set_num";
|
||||
|
||||
COMMIT;
|
@ -1,25 +0,0 @@
|
||||
-- description: Migrate the Bricktracker sets
|
||||
|
||||
PRAGMA foreign_keys = ON;
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Create a Bricktracker set table: with their unique IDs, and a reference to the Rebrickable set
|
||||
CREATE TABLE "bricktracker_sets" (
|
||||
"id" TEXT NOT NULL,
|
||||
"rebrickable_set" TEXT NOT NULL,
|
||||
PRIMARY KEY("id"),
|
||||
FOREIGN KEY("rebrickable_set") REFERENCES "rebrickable_sets"("set")
|
||||
);
|
||||
|
||||
-- Insert existing sets into the new table
|
||||
INSERT INTO "bricktracker_sets" (
|
||||
"id",
|
||||
"rebrickable_set"
|
||||
)
|
||||
SELECT
|
||||
"sets"."u_id",
|
||||
"sets"."set_num"
|
||||
FROM "sets";
|
||||
|
||||
COMMIT;
|
@ -1,72 +0,0 @@
|
||||
-- description: Creation of the configurable set checkboxes
|
||||
|
||||
PRAGMA foreign_keys = ON;
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Create a table to define each set checkbox: with an ID, a name and if they should be displayed on the grid cards
|
||||
CREATE TABLE "bricktracker_set_checkboxes" (
|
||||
"id" TEXT NOT NULL,
|
||||
"name" TEXT NOT NULL,
|
||||
"displayed_on_grid" BOOLEAN NOT NULL DEFAULT 0,
|
||||
PRIMARY KEY("id")
|
||||
);
|
||||
|
||||
-- Seed our checkbox with the 3 original ones
|
||||
INSERT INTO "bricktracker_set_checkboxes" (
|
||||
"id",
|
||||
"name",
|
||||
"displayed_on_grid"
|
||||
) VALUES (
|
||||
"minifigures_collected",
|
||||
"Minifigures are collected",
|
||||
1
|
||||
);
|
||||
|
||||
INSERT INTO "bricktracker_set_checkboxes" (
|
||||
"id",
|
||||
"name",
|
||||
"displayed_on_grid"
|
||||
) VALUES (
|
||||
"set_checked",
|
||||
"Set is checked",
|
||||
1
|
||||
);
|
||||
|
||||
INSERT INTO "bricktracker_set_checkboxes" (
|
||||
"id",
|
||||
"name",
|
||||
"displayed_on_grid"
|
||||
) VALUES (
|
||||
"set_collected",
|
||||
"Set is collected and boxed",
|
||||
1
|
||||
);
|
||||
|
||||
-- Create a table for the status of each checkbox: with the 3 first status
|
||||
CREATE TABLE "bricktracker_set_statuses" (
|
||||
"bricktracker_set_id" TEXT NOT NULL,
|
||||
"status_minifigures_collected" BOOLEAN NOT NULL DEFAULT 0,
|
||||
"status_set_checked" BOOLEAN NOT NULL DEFAULT 0,
|
||||
"status_set_collected" BOOLEAN NOT NULL DEFAULT 0,
|
||||
PRIMARY KEY("bricktracker_set_id"),
|
||||
FOREIGN KEY("bricktracker_set_id") REFERENCES "bricktracker_sets"("id")
|
||||
);
|
||||
|
||||
INSERT INTO "bricktracker_set_statuses" (
|
||||
"bricktracker_set_id",
|
||||
"status_minifigures_collected",
|
||||
"status_set_checked",
|
||||
"status_set_collected"
|
||||
)
|
||||
SELECT
|
||||
"sets"."u_id",
|
||||
"sets"."mini_col",
|
||||
"sets"."set_check",
|
||||
"sets"."set_col"
|
||||
FROM "sets";
|
||||
|
||||
-- Rename the original table (don't delete it yet?)
|
||||
ALTER TABLE "sets" RENAME TO "sets_old";
|
||||
|
||||
COMMIT;
|
@ -1,42 +0,0 @@
|
||||
-- description: Migrate the whislist to have a Rebrickable sets structure
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Create a Rebrickable wish table: each unique (light) set imported from Rebrickable
|
||||
CREATE TABLE "bricktracker_wishes" (
|
||||
"set" TEXT NOT NULL,
|
||||
"name" TEXT NOT NULL,
|
||||
"year" INTEGER NOT NULL,
|
||||
"theme_id" INTEGER NOT NULL,
|
||||
"number_of_parts" INTEGER NOT NULL,
|
||||
"image" TEXT,
|
||||
"url" TEXT,
|
||||
PRIMARY KEY("set")
|
||||
);
|
||||
|
||||
-- Insert existing wishes into the new table
|
||||
INSERT INTO "bricktracker_wishes" (
|
||||
"set",
|
||||
"name",
|
||||
"year",
|
||||
"theme_id",
|
||||
"number_of_parts",
|
||||
"image",
|
||||
"url"
|
||||
)
|
||||
SELECT
|
||||
"wishlist"."set_num",
|
||||
"wishlist"."name",
|
||||
"wishlist"."year",
|
||||
"wishlist"."theme_id",
|
||||
"wishlist"."num_parts",
|
||||
"wishlist"."set_img_url",
|
||||
"wishlist"."set_url"
|
||||
FROM "wishlist"
|
||||
GROUP BY
|
||||
"wishlist"."set_num";
|
||||
|
||||
-- Rename the original table (don't delete it yet?)
|
||||
ALTER TABLE "wishlist" RENAME TO "wishlist_old";
|
||||
|
||||
COMMIT;
|
@ -1,74 +0,0 @@
|
||||
-- description: Renaming various complicated field names to something simpler, and add a bunch of extra fields for later
|
||||
|
||||
PRAGMA foreign_keys = ON;
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Rename sets table
|
||||
ALTER TABLE "bricktracker_sets" RENAME TO "bricktracker_sets_old";
|
||||
|
||||
-- Create a Bricktracker metadata storage table for later
|
||||
CREATE TABLE "bricktracker_metadata_storages" (
|
||||
"id" TEXT NOT NULL,
|
||||
"name" TEXT NOT NULL,
|
||||
PRIMARY KEY("id")
|
||||
);
|
||||
|
||||
-- Create a Bricktracker metadata purchase location table for later
|
||||
CREATE TABLE "bricktracker_metadata_purchase_locations" (
|
||||
"id" TEXT NOT NULL,
|
||||
"name" TEXT NOT NULL,
|
||||
PRIMARY KEY("id")
|
||||
);
|
||||
|
||||
-- Re-Create a Bricktracker set table with the simplified name
|
||||
CREATE TABLE "bricktracker_sets" (
|
||||
"id" TEXT NOT NULL,
|
||||
"set" TEXT NOT NULL,
|
||||
"description" TEXT,
|
||||
"storage" TEXT, -- Storage bin location
|
||||
"purchase_date" REAL, -- Purchase data
|
||||
"purchase_location" TEXT, -- Purchase location
|
||||
"purchase_price" REAL, -- Purchase price
|
||||
PRIMARY KEY("id"),
|
||||
FOREIGN KEY("set") REFERENCES "rebrickable_sets"("set"),
|
||||
FOREIGN KEY("storage") REFERENCES "bricktracker_metadata_storages"("id"),
|
||||
FOREIGN KEY("purchase_location") REFERENCES "bricktracker_metadata_purchase_locations"("id")
|
||||
);
|
||||
|
||||
-- Insert existing sets into the new table
|
||||
INSERT INTO "bricktracker_sets" (
|
||||
"id",
|
||||
"set"
|
||||
)
|
||||
SELECT
|
||||
"bricktracker_sets_old"."id",
|
||||
"bricktracker_sets_old"."rebrickable_set"
|
||||
FROM "bricktracker_sets_old";
|
||||
|
||||
-- Rename status table
|
||||
ALTER TABLE "bricktracker_set_statuses" RENAME TO "bricktracker_set_statuses_old";
|
||||
|
||||
-- Re-create a table for the status of each checkbox
|
||||
CREATE TABLE "bricktracker_set_statuses" (
|
||||
"id" TEXT NOT NULL,
|
||||
{% if structure %}{{ structure }},{% endif %}
|
||||
PRIMARY KEY("id"),
|
||||
FOREIGN KEY("id") REFERENCES "bricktracker_sets"("id")
|
||||
);
|
||||
|
||||
-- Insert existing status into the new table
|
||||
INSERT INTO "bricktracker_set_statuses" (
|
||||
{% if targets %}{{ targets }},{% endif %}
|
||||
"id"
|
||||
)
|
||||
SELECT
|
||||
{% if sources %}{{ sources }},{% endif %}
|
||||
"bricktracker_set_statuses_old"."bricktracker_set_id"
|
||||
FROM "bricktracker_set_statuses_old";
|
||||
|
||||
-- Delete the original tables
|
||||
DROP TABLE "bricktracker_set_statuses_old";
|
||||
DROP TABLE "bricktracker_sets_old";
|
||||
|
||||
COMMIT;
|
@ -1,30 +0,0 @@
|
||||
-- description: Creation of the deduplicated table of Rebrickable minifigures
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Create a Rebrickable minifigures table: each unique minifigure imported from Rebrickable
|
||||
CREATE TABLE "rebrickable_minifigures" (
|
||||
"figure" TEXT NOT NULL,
|
||||
"number" INTEGER NOT NULL,
|
||||
"name" TEXT NOT NULL,
|
||||
"image" TEXT,
|
||||
PRIMARY KEY("figure")
|
||||
);
|
||||
|
||||
-- Insert existing sets into the new table
|
||||
INSERT INTO "rebrickable_minifigures" (
|
||||
"figure",
|
||||
"number",
|
||||
"name",
|
||||
"image"
|
||||
)
|
||||
SELECT
|
||||
"minifigures"."fig_num",
|
||||
CAST(SUBSTR("minifigures"."fig_num", 5) AS INTEGER),
|
||||
"minifigures"."name",
|
||||
"minifigures"."set_img_url"
|
||||
FROM "minifigures"
|
||||
GROUP BY
|
||||
"minifigures"."fig_num";
|
||||
|
||||
COMMIT;
|
@ -1,32 +0,0 @@
|
||||
-- description: Migrate the Bricktracker minifigures
|
||||
|
||||
PRAGMA foreign_keys = ON;
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Create a Bricktracker minifigures table: an amount of minifigures linked to a Bricktracker set
|
||||
CREATE TABLE "bricktracker_minifigures" (
|
||||
"id" TEXT NOT NULL,
|
||||
"figure" TEXT NOT NULL,
|
||||
"quantity" INTEGER NOT NULL,
|
||||
PRIMARY KEY("id", "figure"),
|
||||
FOREIGN KEY("id") REFERENCES "bricktracker_sets"("id"),
|
||||
FOREIGN KEY("figure") REFERENCES "rebrickable_minifigures"("figure")
|
||||
);
|
||||
|
||||
-- Insert existing sets into the new table
|
||||
INSERT INTO "bricktracker_minifigures" (
|
||||
"id",
|
||||
"figure",
|
||||
"quantity"
|
||||
)
|
||||
SELECT
|
||||
"minifigures"."u_id",
|
||||
"minifigures"."fig_num",
|
||||
"minifigures"."quantity"
|
||||
FROM "minifigures";
|
||||
|
||||
-- Rename the original table (don't delete it yet?)
|
||||
ALTER TABLE "minifigures" RENAME TO "minifigures_old";
|
||||
|
||||
COMMIT;
|
@ -1,42 +0,0 @@
|
||||
-- description: Creation of the deduplicated table of Rebrickable parts, and add a bunch of extra fields for later
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Create a Rebrickable parts table: each unique part imported from Rebrickable
|
||||
CREATE TABLE "rebrickable_parts" (
|
||||
"part" TEXT NOT NULL,
|
||||
"color_id" INTEGER NOT NULL,
|
||||
"color_name" TEXT NOT NULL,
|
||||
"color_rgb" TEXT, -- can be NULL because it was not saved before
|
||||
"color_transparent" BOOLEAN, -- can be NULL because it was not saved before
|
||||
"name" TEXT NOT NULL,
|
||||
"category" INTEGER, -- can be NULL because it was not saved before
|
||||
"image" TEXT,
|
||||
"image_id" TEXT,
|
||||
"url" TEXT, -- can be NULL because it was not saved before
|
||||
"print" INTEGER, -- can be NULL, was not saved before
|
||||
PRIMARY KEY("part", "color_id")
|
||||
);
|
||||
|
||||
-- Insert existing parts into the new table
|
||||
INSERT INTO "rebrickable_parts" (
|
||||
"part",
|
||||
"color_id",
|
||||
"color_name",
|
||||
"name",
|
||||
"image",
|
||||
"image_id"
|
||||
)
|
||||
SELECT
|
||||
"inventory"."part_num",
|
||||
"inventory"."color_id",
|
||||
"inventory"."color_name",
|
||||
"inventory"."name",
|
||||
"inventory"."part_img_url",
|
||||
"inventory"."part_img_url_id"
|
||||
FROM "inventory"
|
||||
GROUP BY
|
||||
"inventory"."part_num",
|
||||
"inventory"."color_id";
|
||||
|
||||
COMMIT;
|
@ -1,73 +0,0 @@
|
||||
-- description: Migrate the Bricktracker parts (and missing parts), and add a bunch of extra fields for later
|
||||
|
||||
PRAGMA foreign_keys = ON;
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Fix: somehow a deletion bug was introduced in an older release?
|
||||
DELETE FROM "inventory"
|
||||
WHERE "inventory"."u_id" NOT IN (
|
||||
SELECT "bricktracker_sets"."id"
|
||||
FROM "bricktracker_sets"
|
||||
);
|
||||
|
||||
DELETE FROM "missing"
|
||||
WHERE "missing"."u_id" NOT IN (
|
||||
SELECT "bricktracker_sets"."id"
|
||||
FROM "bricktracker_sets"
|
||||
);
|
||||
|
||||
-- Create a Bricktracker parts table: an amount of parts linked to a Bricktracker set
|
||||
CREATE TABLE "bricktracker_parts" (
|
||||
"id" TEXT NOT NULL,
|
||||
"figure" TEXT,
|
||||
"part" TEXT NOT NULL,
|
||||
"color" INTEGER NOT NULL,
|
||||
"spare" BOOLEAN NOT NULL,
|
||||
"quantity" INTEGER NOT NULL,
|
||||
"element" INTEGER,
|
||||
"rebrickable_inventory" INTEGER NOT NULL,
|
||||
"missing" INTEGER NOT NULL DEFAULT 0,
|
||||
"damaged" INTEGER NOT NULL DEFAULT 0,
|
||||
PRIMARY KEY("id", "figure", "part", "color", "spare"),
|
||||
FOREIGN KEY("id") REFERENCES "bricktracker_sets"("id"),
|
||||
FOREIGN KEY("figure") REFERENCES "rebrickable_minifigures"("figure"),
|
||||
FOREIGN KEY("part", "color") REFERENCES "rebrickable_parts"("part", "color_id")
|
||||
);
|
||||
|
||||
-- Insert existing parts into the new table
|
||||
INSERT INTO "bricktracker_parts" (
|
||||
"id",
|
||||
"figure",
|
||||
"part",
|
||||
"color",
|
||||
"spare",
|
||||
"quantity",
|
||||
"element",
|
||||
"rebrickable_inventory",
|
||||
"missing"
|
||||
)
|
||||
SELECT
|
||||
"inventory"."u_id",
|
||||
CASE WHEN SUBSTR("inventory"."set_num", 0, 5) = 'fig-' THEN "inventory"."set_num" ELSE NULL END,
|
||||
"inventory"."part_num",
|
||||
"inventory"."color_id",
|
||||
"inventory"."is_spare",
|
||||
"inventory"."quantity",
|
||||
"inventory"."element_id",
|
||||
"inventory"."id",
|
||||
IFNULL("missing"."quantity", 0)
|
||||
FROM "inventory"
|
||||
LEFT JOIN "missing"
|
||||
ON "inventory"."set_num" IS NOT DISTINCT FROM "missing"."set_num"
|
||||
AND "inventory"."id" IS NOT DISTINCT FROM "missing"."id"
|
||||
AND "inventory"."part_num" IS NOT DISTINCT FROM "missing"."part_num"
|
||||
AND "inventory"."color_id" IS NOT DISTINCT FROM "missing"."color_id"
|
||||
AND "inventory"."element_id" IS NOT DISTINCT FROM "missing"."element_id"
|
||||
AND "inventory"."u_id" IS NOT DISTINCT FROM "missing"."u_id";
|
||||
|
||||
-- Rename the original table (don't delete it yet?)
|
||||
ALTER TABLE "inventory" RENAME TO "inventory_old";
|
||||
ALTER TABLE "missing" RENAME TO "missing_old";
|
||||
|
||||
COMMIT;
|
@ -1,7 +0,0 @@
|
||||
-- description: Rename checkboxes to status metadata
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
ALTER TABLE "bricktracker_set_checkboxes" RENAME TO "bricktracker_metadata_statuses";
|
||||
|
||||
COMMIT;
|
@ -1,26 +0,0 @@
|
||||
-- description: Add set owners
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Create a table to define each set owners: an id and a name
|
||||
CREATE TABLE "bricktracker_metadata_owners" (
|
||||
"id" TEXT NOT NULL,
|
||||
"name" TEXT NOT NULL,
|
||||
PRIMARY KEY("id")
|
||||
);
|
||||
|
||||
-- Create a table for the set owners
|
||||
CREATE TABLE "bricktracker_set_owners" (
|
||||
"id" TEXT NOT NULL,
|
||||
PRIMARY KEY("id"),
|
||||
FOREIGN KEY("id") REFERENCES "bricktracker_sets"("id")
|
||||
);
|
||||
|
||||
-- Create a table for the wish owners
|
||||
CREATE TABLE "bricktracker_wish_owners" (
|
||||
"set" TEXT NOT NULL,
|
||||
PRIMARY KEY("set"),
|
||||
FOREIGN KEY("set") REFERENCES "bricktracker_wishes"("set")
|
||||
);
|
||||
|
||||
COMMIT;
|
@ -1,19 +0,0 @@
|
||||
-- description: Add set tags
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Create a table to define each set tags: an id and a name
|
||||
CREATE TABLE "bricktracker_metadata_tags" (
|
||||
"id" TEXT NOT NULL,
|
||||
"name" TEXT NOT NULL,
|
||||
PRIMARY KEY("id")
|
||||
);
|
||||
|
||||
-- Create a table for the set tags
|
||||
CREATE TABLE "bricktracker_set_tags" (
|
||||
"id" TEXT NOT NULL,
|
||||
PRIMARY KEY("id"),
|
||||
FOREIGN KEY("id") REFERENCES "bricktracker_sets"("id")
|
||||
);
|
||||
|
||||
COMMIT;
|
@ -1,32 +0,0 @@
|
||||
-- description: Add number of parts for minifigures
|
||||
|
||||
BEGIN TRANSACTION;
|
||||
|
||||
-- Add the number_of_parts column to the minifigures
|
||||
ALTER TABLE "rebrickable_minifigures"
|
||||
ADD COLUMN "number_of_parts" INTEGER NOT NULL DEFAULT 0;
|
||||
|
||||
-- Update the number of parts for each minifigure
|
||||
UPDATE "rebrickable_minifigures"
|
||||
SET "number_of_parts" = "parts_sum"."number_of_parts"
|
||||
FROM (
|
||||
SELECT
|
||||
"parts"."figure",
|
||||
SUM("parts"."quantity") as "number_of_parts"
|
||||
FROM (
|
||||
SELECT
|
||||
"bricktracker_parts"."figure",
|
||||
"bricktracker_parts"."quantity"
|
||||
FROM "bricktracker_parts"
|
||||
WHERE "bricktracker_parts"."figure" IS NOT NULL
|
||||
GROUP BY
|
||||
"bricktracker_parts"."figure",
|
||||
"bricktracker_parts"."part",
|
||||
"bricktracker_parts"."color",
|
||||
"bricktracker_parts"."spare"
|
||||
) "parts"
|
||||
GROUP BY "parts"."figure"
|
||||
) "parts_sum"
|
||||
WHERE "rebrickable_minifigures"."figure" = "parts_sum"."figure";
|
||||
|
||||
COMMIT;
|
@ -1,37 +0,0 @@
|
||||
SELECT
|
||||
"bricktracker_minifigures"."quantity",
|
||||
"rebrickable_minifigures"."figure",
|
||||
"rebrickable_minifigures"."number",
|
||||
"rebrickable_minifigures"."number_of_parts",
|
||||
"rebrickable_minifigures"."name",
|
||||
"rebrickable_minifigures"."image",
|
||||
{% block total_missing %}
|
||||
NULL AS "total_missing", -- dummy for order: total_missing
|
||||
{% endblock %}
|
||||
{% block total_damaged %}
|
||||
NULL AS "total_damaged", -- dummy for order: total_damaged
|
||||
{% endblock %}
|
||||
{% block total_quantity %}
|
||||
NULL AS "total_quantity", -- dummy for order: total_quantity
|
||||
{% endblock %}
|
||||
{% block total_sets %}
|
||||
NULL AS "total_sets" -- dummy for order: total_sets
|
||||
{% endblock %}
|
||||
FROM "bricktracker_minifigures"
|
||||
|
||||
INNER JOIN "rebrickable_minifigures"
|
||||
ON "bricktracker_minifigures"."figure" IS NOT DISTINCT FROM "rebrickable_minifigures"."figure"
|
||||
|
||||
{% block join %}{% endblock %}
|
||||
|
||||
{% block where %}{% endblock %}
|
||||
|
||||
{% block group %}{% endblock %}
|
||||
|
||||
{% if order %}
|
||||
ORDER BY {{ order }}
|
||||
{% endif %}
|
||||
|
||||
{% if limit %}
|
||||
LIMIT {{ limit }}
|
||||
{% endif %}
|
@ -1,9 +0,0 @@
|
||||
INSERT INTO "bricktracker_minifigures" (
|
||||
"id",
|
||||
"figure",
|
||||
"quantity"
|
||||
) VALUES (
|
||||
:id,
|
||||
:figure,
|
||||
:quantity
|
||||
)
|
@ -1,40 +0,0 @@
|
||||
{% extends 'minifigure/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}
|
||||
SUM(IFNULL("problem_join"."total_missing", 0)) AS "total_missing",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_damaged %}
|
||||
SUM(IFNULL("problem_join"."total_damaged", 0)) AS "total_damaged",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_quantity %}
|
||||
SUM(IFNULL("bricktracker_minifigures"."quantity", 0)) AS "total_quantity",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_sets %}
|
||||
IFNULL(COUNT("bricktracker_minifigures"."id"), 0) AS "total_sets"
|
||||
{% endblock %}
|
||||
|
||||
{% block join %}
|
||||
-- LEFT JOIN + SELECT to avoid messing the total
|
||||
LEFT JOIN (
|
||||
SELECT
|
||||
"bricktracker_parts"."id",
|
||||
"bricktracker_parts"."figure",
|
||||
SUM("bricktracker_parts"."missing") AS "total_missing",
|
||||
SUM("bricktracker_parts"."damaged") AS "total_damaged"
|
||||
FROM "bricktracker_parts"
|
||||
WHERE "bricktracker_parts"."figure" IS NOT NULL
|
||||
GROUP BY
|
||||
"bricktracker_parts"."id",
|
||||
"bricktracker_parts"."figure"
|
||||
) "problem_join"
|
||||
ON "bricktracker_minifigures"."id" IS NOT DISTINCT FROM "problem_join"."id"
|
||||
AND "rebrickable_minifigures"."figure" IS NOT DISTINCT FROM "problem_join"."figure"
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"rebrickable_minifigures"."figure"
|
||||
{% endblock %}
|
@ -1,28 +0,0 @@
|
||||
{% extends 'minifigure/base/base.sql' %}
|
||||
|
||||
{% block total_damaged %}
|
||||
SUM("bricktracker_parts"."damaged") AS "total_damaged",
|
||||
{% endblock %}
|
||||
|
||||
{% block join %}
|
||||
LEFT JOIN "bricktracker_parts"
|
||||
ON "bricktracker_minifigures"."id" IS NOT DISTINCT FROM "bricktracker_parts"."id"
|
||||
AND "rebrickable_minifigures"."figure" IS NOT DISTINCT FROM "bricktracker_parts"."figure"
|
||||
{% endblock %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "rebrickable_minifigures"."figure" IN (
|
||||
SELECT "bricktracker_parts"."figure"
|
||||
FROM "bricktracker_parts"
|
||||
WHERE "bricktracker_parts"."part" IS NOT DISTINCT FROM :part
|
||||
AND "bricktracker_parts"."color" IS NOT DISTINCT FROM :color
|
||||
AND "bricktracker_parts"."figure" IS NOT NULL
|
||||
AND "bricktracker_parts"."damaged" > 0
|
||||
GROUP BY "bricktracker_parts"."figure"
|
||||
)
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"rebrickable_minifigures"."figure"
|
||||
{% endblock %}
|
@ -1,5 +0,0 @@
|
||||
{% extends 'minifigure/base/base.sql' %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "bricktracker_minifigures"."id" IS NOT DISTINCT FROM :id
|
||||
{% endblock %}
|
@ -1,21 +0,0 @@
|
||||
{% extends 'minifigure/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}
|
||||
SUM("bricktracker_parts"."missing") AS "total_missing",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_damaged %}
|
||||
SUM("bricktracker_parts"."damaged") AS "total_damaged",
|
||||
{% endblock %}
|
||||
|
||||
{% block join %}
|
||||
LEFT JOIN "bricktracker_parts"
|
||||
ON "bricktracker_minifigures"."id" IS NOT DISTINCT FROM "bricktracker_parts"."id"
|
||||
AND "rebrickable_minifigures"."figure" IS NOT DISTINCT FROM "bricktracker_parts"."figure"
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"rebrickable_minifigures"."figure",
|
||||
"bricktracker_minifigures"."id"
|
||||
{% endblock %}
|
@ -1,28 +0,0 @@
|
||||
{% extends 'minifigure/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}
|
||||
SUM("bricktracker_parts"."missing") AS "total_missing",
|
||||
{% endblock %}
|
||||
|
||||
{% block join %}
|
||||
LEFT JOIN "bricktracker_parts"
|
||||
ON "bricktracker_minifigures"."id" IS NOT DISTINCT FROM "bricktracker_parts"."id"
|
||||
AND "rebrickable_minifigures"."figure" IS NOT DISTINCT FROM "bricktracker_parts"."figure"
|
||||
{% endblock %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "rebrickable_minifigures"."figure" IN (
|
||||
SELECT "bricktracker_parts"."figure"
|
||||
FROM "bricktracker_parts"
|
||||
WHERE "bricktracker_parts"."part" IS NOT DISTINCT FROM :part
|
||||
AND "bricktracker_parts"."color" IS NOT DISTINCT FROM :color
|
||||
AND "bricktracker_parts"."figure" IS NOT NULL
|
||||
AND "bricktracker_parts"."missing" > 0
|
||||
GROUP BY "bricktracker_parts"."figure"
|
||||
)
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"rebrickable_minifigures"."figure"
|
||||
{% endblock %}
|
@ -1,21 +0,0 @@
|
||||
{% extends 'minifigure/base/base.sql' %}
|
||||
|
||||
{% block total_quantity %}
|
||||
SUM("bricktracker_minifigures"."quantity") AS "total_quantity",
|
||||
{% endblock %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "rebrickable_minifigures"."figure" IN (
|
||||
SELECT "bricktracker_parts"."figure"
|
||||
FROM "bricktracker_parts"
|
||||
WHERE "bricktracker_parts"."part" IS NOT DISTINCT FROM :part
|
||||
AND "bricktracker_parts"."color" IS NOT DISTINCT FROM :color
|
||||
AND "bricktracker_parts"."figure" IS NOT NULL
|
||||
GROUP BY "bricktracker_parts"."figure"
|
||||
)
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"rebrickable_minifigures"."figure"
|
||||
{% endblock %}
|
@ -1,40 +0,0 @@
|
||||
{% extends 'minifigure/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}
|
||||
IFNULL("problem_join"."total_missing", 0) AS "total_missing",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_damaged %}
|
||||
IFNULL("problem_join"."total_damaged", 0) AS "total_damaged",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_quantity %}
|
||||
SUM(IFNULL("bricktracker_minifigures"."quantity", 0)) AS "total_quantity",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_sets %}
|
||||
IFNULL(COUNT(DISTINCT "bricktracker_minifigures"."id"), 0) AS "total_sets"
|
||||
{% endblock %}
|
||||
|
||||
{% block join %}
|
||||
-- LEFT JOIN + SELECT to avoid messing the total
|
||||
LEFT JOIN (
|
||||
SELECT
|
||||
"bricktracker_parts"."figure",
|
||||
SUM("bricktracker_parts"."missing") AS "total_missing",
|
||||
SUM("bricktracker_parts"."damaged") AS "total_damaged"
|
||||
FROM "bricktracker_parts"
|
||||
WHERE "bricktracker_parts"."figure" IS NOT DISTINCT FROM :figure
|
||||
GROUP BY "bricktracker_parts"."figure"
|
||||
) "problem_join"
|
||||
ON "rebrickable_minifigures"."figure" IS NOT DISTINCT FROM "problem_join"."figure"
|
||||
{% endblock %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "rebrickable_minifigures"."figure" IS NOT DISTINCT FROM :figure
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"rebrickable_minifigures"."figure"
|
||||
{% endblock %}
|
@ -1,6 +0,0 @@
|
||||
{% extends 'minifigure/base/base.sql' %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "bricktracker_minifigures"."id" IS NOT DISTINCT FROM :id
|
||||
AND "rebrickable_minifigures"."figure" IS NOT DISTINCT FROM :figure
|
||||
{% endblock %}
|
@ -1,59 +0,0 @@
|
||||
SELECT
|
||||
"bricktracker_parts"."id",
|
||||
"bricktracker_parts"."figure",
|
||||
"bricktracker_parts"."part",
|
||||
"bricktracker_parts"."color",
|
||||
"bricktracker_parts"."spare",
|
||||
"bricktracker_parts"."quantity",
|
||||
"bricktracker_parts"."element",
|
||||
--"bricktracker_parts"."rebrickable_inventory",
|
||||
"bricktracker_parts"."missing",
|
||||
"bricktracker_parts"."damaged",
|
||||
--"rebrickable_parts"."part",
|
||||
--"rebrickable_parts"."color_id",
|
||||
"rebrickable_parts"."color_name",
|
||||
"rebrickable_parts"."color_rgb",
|
||||
"rebrickable_parts"."color_transparent",
|
||||
"rebrickable_parts"."name",
|
||||
--"rebrickable_parts"."category",
|
||||
"rebrickable_parts"."image",
|
||||
"rebrickable_parts"."image_id",
|
||||
"rebrickable_parts"."url",
|
||||
"rebrickable_parts"."print",
|
||||
{% block total_missing %}
|
||||
NULL AS "total_missing", -- dummy for order: total_missing
|
||||
{% endblock %}
|
||||
{% block total_damaged %}
|
||||
NULL AS "total_damaged", -- dummy for order: total_damaged
|
||||
{% endblock %}
|
||||
{% block total_quantity %}
|
||||
NULL AS "total_quantity", -- dummy for order: total_quantity
|
||||
{% endblock %}
|
||||
{% block total_spare %}
|
||||
NULL AS "total_spare", -- dummy for order: total_spare
|
||||
{% endblock %}
|
||||
{% block total_sets %}
|
||||
NULL AS "total_sets", -- dummy for order: total_sets
|
||||
{% endblock %}
|
||||
{% block total_minifigures %}
|
||||
NULL AS "total_minifigures" -- dummy for order: total_minifigures
|
||||
{% endblock %}
|
||||
FROM "bricktracker_parts"
|
||||
|
||||
INNER JOIN "rebrickable_parts"
|
||||
ON "bricktracker_parts"."part" IS NOT DISTINCT FROM "rebrickable_parts"."part"
|
||||
AND "bricktracker_parts"."color" IS NOT DISTINCT FROM "rebrickable_parts"."color_id"
|
||||
|
||||
{% block join %}{% endblock %}
|
||||
|
||||
{% block where %}{% endblock %}
|
||||
|
||||
{% block group %}{% endblock %}
|
||||
|
||||
{% if order %}
|
||||
ORDER BY {{ order }}
|
||||
{% endif %}
|
||||
|
||||
{% if limit %}
|
||||
LIMIT {{ limit }}
|
||||
{% endif %}
|
@ -1,19 +0,0 @@
|
||||
INSERT INTO "bricktracker_parts" (
|
||||
"id",
|
||||
"figure",
|
||||
"part",
|
||||
"color",
|
||||
"spare",
|
||||
"quantity",
|
||||
"element",
|
||||
"rebrickable_inventory"
|
||||
) VALUES (
|
||||
:id,
|
||||
:figure,
|
||||
:part,
|
||||
:color,
|
||||
:spare,
|
||||
:quantity,
|
||||
:element,
|
||||
:rebrickable_inventory
|
||||
)
|
@ -1,34 +0,0 @@
|
||||
{% extends 'part/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}
|
||||
SUM("bricktracker_parts"."missing") AS "total_missing",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_damaged %}
|
||||
SUM("bricktracker_parts"."damaged") AS "total_damaged",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_quantity %}
|
||||
SUM("bricktracker_parts"."quantity" * IFNULL("bricktracker_minifigures"."quantity", 1)) AS "total_quantity",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_sets %}
|
||||
IFNULL(COUNT(DISTINCT "bricktracker_parts"."id"), 0) AS "total_sets",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_minifigures %}
|
||||
SUM(IFNULL("bricktracker_minifigures"."quantity", 0)) AS "total_minifigures"
|
||||
{% endblock %}
|
||||
|
||||
{% block join %}
|
||||
LEFT JOIN "bricktracker_minifigures"
|
||||
ON "bricktracker_parts"."id" IS NOT DISTINCT FROM "bricktracker_minifigures"."id"
|
||||
AND "bricktracker_parts"."figure" IS NOT DISTINCT FROM "bricktracker_minifigures"."figure"
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"bricktracker_parts"."part",
|
||||
"bricktracker_parts"."color",
|
||||
"bricktracker_parts"."spare"
|
||||
{% endblock %}
|
@ -1,21 +0,0 @@
|
||||
|
||||
{% extends 'part/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}
|
||||
SUM("bricktracker_parts"."missing") AS "total_missing",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_damaged %}
|
||||
SUM("bricktracker_parts"."damaged") AS "total_damaged",
|
||||
{% endblock %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "bricktracker_parts"."figure" IS NOT DISTINCT FROM :figure
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"bricktracker_parts"."part",
|
||||
"bricktracker_parts"."color",
|
||||
"bricktracker_parts"."spare"
|
||||
{% endblock %}
|
@ -1,18 +0,0 @@
|
||||
|
||||
{% extends 'part/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}{% endblock %}
|
||||
|
||||
{% block total_damaged %}{% endblock %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "rebrickable_parts"."print" IS NOT DISTINCT FROM :print
|
||||
AND "bricktracker_parts"."color" IS NOT DISTINCT FROM :color
|
||||
AND "bricktracker_parts"."part" IS DISTINCT FROM :part
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"bricktracker_parts"."part",
|
||||
"bricktracker_parts"."color"
|
||||
{% endblock %}
|
@ -1,35 +0,0 @@
|
||||
{% extends 'part/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}
|
||||
SUM("bricktracker_parts"."missing") AS "total_missing",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_damaged %}
|
||||
SUM("bricktracker_parts"."damaged") AS "total_damaged",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_sets %}
|
||||
IFNULL(COUNT("bricktracker_parts"."id"), 0) - IFNULL(COUNT("bricktracker_parts"."figure"), 0) AS "total_sets",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_minifigures %}
|
||||
SUM(IFNULL("bricktracker_minifigures"."quantity", 0)) AS "total_minifigures"
|
||||
{% endblock %}
|
||||
|
||||
{% block join %}
|
||||
LEFT JOIN "bricktracker_minifigures"
|
||||
ON "bricktracker_parts"."id" IS NOT DISTINCT FROM "bricktracker_minifigures"."id"
|
||||
AND "bricktracker_parts"."figure" IS NOT DISTINCT FROM "bricktracker_minifigures"."figure"
|
||||
{% endblock %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "bricktracker_parts"."missing" > 0
|
||||
OR "bricktracker_parts"."damaged" > 0
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"bricktracker_parts"."part",
|
||||
"bricktracker_parts"."color",
|
||||
"bricktracker_parts"."spare"
|
||||
{% endblock %}
|
@ -1,15 +0,0 @@
|
||||
|
||||
{% extends 'part/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}
|
||||
IFNULL("bricktracker_parts"."missing", 0) AS "total_missing",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_damaged %}
|
||||
IFNULL("bricktracker_parts"."damaged", 0) AS "total_damaged",
|
||||
{% endblock %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "bricktracker_parts"."id" IS NOT DISTINCT FROM :id
|
||||
AND "bricktracker_parts"."figure" IS NOT DISTINCT FROM :figure
|
||||
{% endblock %}
|
@ -1,17 +0,0 @@
|
||||
|
||||
{% extends 'part/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}{% endblock %}
|
||||
|
||||
{% block total_damaged %}{% endblock %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "bricktracker_parts"."color" IS DISTINCT FROM :color
|
||||
AND "bricktracker_parts"."part" IS NOT DISTINCT FROM :part
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"bricktracker_parts"."part",
|
||||
"bricktracker_parts"."color"
|
||||
{% endblock %}
|
@ -1,34 +0,0 @@
|
||||
{% extends 'part/base/base.sql' %}
|
||||
|
||||
{% block total_missing %}
|
||||
SUM("bricktracker_parts"."missing") AS "total_missing",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_damaged %}
|
||||
SUM("bricktracker_parts"."damaged") AS "total_damaged",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_quantity %}
|
||||
SUM((NOT "bricktracker_parts"."spare") * "bricktracker_parts"."quantity" * IFNULL("bricktracker_minifigures"."quantity", 1)) AS "total_quantity",
|
||||
{% endblock %}
|
||||
|
||||
{% block total_spare %}
|
||||
SUM("bricktracker_parts"."spare" * "bricktracker_parts"."quantity" * IFNULL("bricktracker_minifigures"."quantity", 1)) AS "total_spare",
|
||||
{% endblock %}
|
||||
|
||||
{% block join %}
|
||||
LEFT JOIN "bricktracker_minifigures"
|
||||
ON "bricktracker_parts"."id" IS NOT DISTINCT FROM "bricktracker_minifigures"."id"
|
||||
AND "bricktracker_parts"."figure" IS NOT DISTINCT FROM "bricktracker_minifigures"."figure"
|
||||
{% endblock %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "bricktracker_parts"."part" IS NOT DISTINCT FROM :part
|
||||
AND "bricktracker_parts"."color" IS NOT DISTINCT FROM :color
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"bricktracker_parts"."part",
|
||||
"bricktracker_parts"."color"
|
||||
{% endblock %}
|
@ -1,18 +0,0 @@
|
||||
{% extends 'part/base/base.sql' %}
|
||||
|
||||
{% block where %}
|
||||
WHERE "bricktracker_parts"."id" IS NOT DISTINCT FROM :id
|
||||
AND "bricktracker_parts"."figure" IS NOT DISTINCT FROM :figure
|
||||
AND "bricktracker_parts"."part" IS NOT DISTINCT FROM :part
|
||||
AND "bricktracker_parts"."color" IS NOT DISTINCT FROM :color
|
||||
AND "bricktracker_parts"."spare" IS NOT DISTINCT FROM :spare
|
||||
{% endblock %}
|
||||
|
||||
{% block group %}
|
||||
GROUP BY
|
||||
"bricktracker_parts"."id",
|
||||
"bricktracker_parts"."figure",
|
||||
"bricktracker_parts"."part",
|
||||
"bricktracker_parts"."color",
|
||||
"bricktracker_parts"."spare"
|
||||
{% endblock %}
|
@ -1,7 +0,0 @@
|
||||
UPDATE "bricktracker_parts"
|
||||
SET "damaged" = :damaged
|
||||
WHERE "bricktracker_parts"."id" IS NOT DISTINCT FROM :id
|
||||
AND "bricktracker_parts"."figure" IS NOT DISTINCT FROM :figure
|
||||
AND "bricktracker_parts"."part" IS NOT DISTINCT FROM :part
|
||||
AND "bricktracker_parts"."color" IS NOT DISTINCT FROM :color
|
||||
AND "bricktracker_parts"."spare" IS NOT DISTINCT FROM :spare
|
@ -1,7 +0,0 @@
|
||||
UPDATE "bricktracker_parts"
|
||||
SET "missing" = :missing
|
||||
WHERE "bricktracker_parts"."id" IS NOT DISTINCT FROM :id
|
||||
AND "bricktracker_parts"."figure" IS NOT DISTINCT FROM :figure
|
||||
AND "bricktracker_parts"."part" IS NOT DISTINCT FROM :part
|
||||
AND "bricktracker_parts"."color" IS NOT DISTINCT FROM :color
|
||||
AND "bricktracker_parts"."spare" IS NOT DISTINCT FROM :spare
|
@ -1,20 +0,0 @@
|
||||
INSERT OR IGNORE INTO "rebrickable_minifigures" (
|
||||
"figure",
|
||||
"number",
|
||||
"name",
|
||||
"image",
|
||||
"number_of_parts"
|
||||
) VALUES (
|
||||
:figure,
|
||||
:number,
|
||||
:name,
|
||||
:image,
|
||||
:number_of_parts
|
||||
)
|
||||
ON CONFLICT("figure")
|
||||
DO UPDATE SET
|
||||
"number" = :number,
|
||||
"name" = :name,
|
||||
"image" = :image,
|
||||
"number_of_parts" = :number_of_parts
|
||||
WHERE "rebrickable_minifigures"."figure" IS NOT DISTINCT FROM :figure
|
@ -1,6 +0,0 @@
|
||||
SELECT
|
||||
"rebrickable_minifigures"."figure",
|
||||
"rebrickable_minifigures"."number",
|
||||
"rebrickable_minifigures"."name",
|
||||
"rebrickable_minifigures"."image"
|
||||
FROM "rebrickable_minifigures"
|
@ -1,8 +0,0 @@
|
||||
SELECT
|
||||
"rebrickable_minifigures"."figure",
|
||||
"rebrickable_minifigures"."number",
|
||||
"rebrickable_minifigures"."name",
|
||||
"rebrickable_minifigures"."image"
|
||||
FROM "rebrickable_minifigures"
|
||||
|
||||
WHERE "rebrickable_minifigures"."figure" IS NOT DISTINCT FROM :figure
|
@ -1,38 +0,0 @@
|
||||
INSERT OR IGNORE INTO "rebrickable_parts" (
|
||||
"part",
|
||||
"color_id",
|
||||
"color_name",
|
||||
"color_rgb",
|
||||
"color_transparent",
|
||||
"name",
|
||||
"category",
|
||||
"image",
|
||||
"image_id",
|
||||
"url",
|
||||
"print"
|
||||
) VALUES (
|
||||
:part,
|
||||
:color_id,
|
||||
:color_name,
|
||||
:color_rgb,
|
||||
:color_transparent,
|
||||
:name,
|
||||
:category,
|
||||
:image,
|
||||
:image_id,
|
||||
:url,
|
||||
:print
|
||||
)
|
||||
ON CONFLICT("part", "color_id")
|
||||
DO UPDATE SET
|
||||
"color_name" = :color_name,
|
||||
"color_rgb" = :color_rgb,
|
||||
"color_transparent" = :color_transparent,
|
||||
"name" = :name,
|
||||
"category" = :category,
|
||||
"image" = :image,
|
||||
"image_id" = :image_id,
|
||||
"url" = :url,
|
||||
"print" = :print
|
||||
WHERE "rebrickable_parts"."part" IS NOT DISTINCT FROM :part
|
||||
AND "rebrickable_parts"."color_id" IS NOT DISTINCT FROM :color_id
|
@ -1,13 +0,0 @@
|
||||
SELECT
|
||||
"rebrickable_parts"."part",
|
||||
"rebrickable_parts"."color_id",
|
||||
"rebrickable_parts"."color_name",
|
||||
"rebrickable_parts"."color_rgb",
|
||||
"rebrickable_parts"."color_transparent",
|
||||
"rebrickable_parts"."name",
|
||||
"rebrickable_parts"."category",
|
||||
"rebrickable_parts"."image",
|
||||
"rebrickable_parts"."image_id",
|
||||
"rebrickable_parts"."url",
|
||||
"rebrickable_parts"."print"
|
||||
FROM "rebrickable_parts"
|
@ -1,16 +0,0 @@
|
||||
SELECT
|
||||
"rebrickable_parts"."part",
|
||||
"rebrickable_parts"."color_id",
|
||||
"rebrickable_parts"."color_name",
|
||||
"rebrickable_parts"."color_rgb",
|
||||
"rebrickable_parts"."color_transparent",
|
||||
"rebrickable_parts"."name",
|
||||
"rebrickable_parts"."category",
|
||||
"rebrickable_parts"."image",
|
||||
"rebrickable_parts"."image_id",
|
||||
"rebrickable_parts"."url",
|
||||
"rebrickable_parts"."print"
|
||||
FROM "rebrickable_parts"
|
||||
|
||||
WHERE "rebrickable_minifigures"."part" IS NOT DISTINCT FROM :figure
|
||||
AND "rebrickable_minifigures"."color_id" IS NOT DISTINCT FROM :color
|
@ -1,35 +0,0 @@
|
||||
INSERT OR IGNORE INTO "rebrickable_sets" (
|
||||
"set",
|
||||
"number",
|
||||
"version",
|
||||
"name",
|
||||
"year",
|
||||
"theme_id",
|
||||
"number_of_parts",
|
||||
"image",
|
||||
"url",
|
||||
"last_modified"
|
||||
) VALUES (
|
||||
:set,
|
||||
:number,
|
||||
:version,
|
||||
:name,
|
||||
:year,
|
||||
:theme_id,
|
||||
:number_of_parts,
|
||||
:image,
|
||||
:url,
|
||||
:last_modified
|
||||
)
|
||||
ON CONFLICT("set")
|
||||
DO UPDATE SET
|
||||
"number" = :number,
|
||||
"version" = :version,
|
||||
"name" = :name,
|
||||
"year" = :year,
|
||||
"theme_id" = :theme_id,
|
||||
"number_of_parts" = :number_of_parts,
|
||||
"image" = :image,
|
||||
"url" = :url,
|
||||
"last_modified" = :last_modified
|
||||
WHERE "rebrickable_sets"."set" IS NOT DISTINCT FROM :set
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
x
Reference in New Issue
Block a user